ValueError: input array must be 2-d
时间: 2024-05-13 07:18:58 浏览: 22
这个错误通常表示你的输入数组不是二维数组,而是一维数组。在大多数情况下,你需要将输入数组转换为二维数组,以便它可以被正确地传递给你的函数或模型。
你可以使用NumPy库的reshape()函数将一维数组转换为二维数组。例如,如果你有一个一维数组x,你可以使用以下代码将其转换为形状为(n,1)的二维数组:
```python
import numpy as np
x = np.array([1, 2, 3, 4])
x = x.reshape(-1, 1)
```
这将创建一个形状为(4,1)的二维数组,其中每个元素都是一个单独的行向量。现在,你可以将这个数组作为参数传递给你的函数或模型,而不会出现“input array must be 2-d”的错误。
相关问题
ValueError: Input array must be 1 dimensional
这个错误通常是由于输入的数组是一个多维数组,而某个函数只能处理一维数组,导致出现这个错误。例如,有些函数只能处理一维的标签数据,而不能处理多维的矩阵数据。
解决这个错误的方法是检查代码中使用的函数是否能够处理多维数组,如果不能,就需要将多维数组转换为一维数组。常见的方法是使用`numpy`库的`flatten`函数或`ravel`函数将多维数组转换为一维数组。
下面是一个示例,假设我们有一个二维数组`a`,我们需要将其传递给某个函数,但是函数只能处理一维数组,就会出现上述错误:
``` python
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
# 函数只能处理一维数组,出现ValueError: Input array must be 1 dimensional错误
result = some_function(a)
```
这个错误的解决方法是使用`flatten`函数或`ravel`函数将多维数组转换为一维数组:
``` python
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
# 将二维数组a转换为一维数组
a_flat = a.flatten()
# 或者使用ravel函数
a_ravel = np.ravel(a)
# 将一维数组传递给函数
result = some_function(a_flat)
```
这样就可以解决这个错误。需要注意的是,使用`flatten`函数或`ravel`函数转换数组时,如果对新的数组进行修改,会影响原始数组。因此,在使用这些函数时需要注意这一点。
raise ValueError("Input must be >= 2-d.") ValueError: Input must be >= 2-d.
This error message indicates that the input provided to a function or method should be a matrix or an array with at least two dimensions. In other words, the input should have multiple rows and columns, rather than being a one-dimensional list or array.
To fix this error, you can modify the input so that it has at least two dimensions. For example, if you have a list of numbers, you can convert it to a 2D array using numpy:
```
import numpy as np
my_list = [1, 2, 3, 4, 5]
my_array = np.array(my_list).reshape(-1, 1)
```
This will create a 2D array with one column and five rows. If you need a different shape, you can adjust the reshape arguments accordingly. Once you have a 2D array, you can pass it to the function or method without encountering the ValueError.
相关推荐
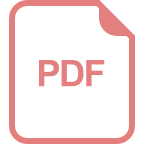
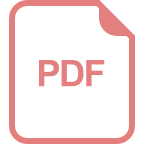
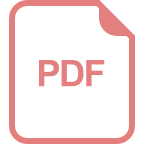













