public class CleanUtil { public static void deleleFilesOfDir(String dirPathName, int retainHours) { File dir = new File(dirPathName); File[] listFile = dir.listFiles(); if (listFile == null) { return; } long now = System.currentTimeMillis(); for (File file : listFile) { deleteFile(now, retainHours, file); } } private static void deleteFile(long now, int retainHours, File file) { try { BasicFileAttributes basicAttr = Files.readAttributes(file.toPath(), BasicFileAttributes.class); long fileTime = basicAttr.lastAccessTime().toMillis(); deleteOverDueFile(retainHours, file, now, fileTime); } catch (IOException | RuntimeException e) { log.warn("failed to read file attributes: {}", file.getAbsolutePath(), e); } } private static void deleteOverDueFile(int retainHours, File file, long now, long fileTime) { if (isOverDue(retainHours, now, fileTime)) { boolean isDeleted = FileUtils.deleteQuietly(file); if (!isDeleted) { log.warn("file deleted faild: {}", file.getAbsolutePath()); } } }需要你这边写UT覆盖,包括异常测试
时间: 2024-01-12 09:05:37 浏览: 92
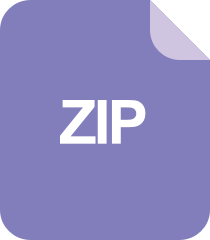
Get-Mp3-File-From-Storage
好的,我可以为这段代码编写一些单元测试,包括正常情况和异常情况的覆盖。下面是一个简单的示例,供您参考:
```java
import org.apache.commons.io.FileUtils;
import org.junit.jupiter.api.Test;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.attribute.BasicFileAttributes;
import static org.junit.jupiter.api.Assertions.*;
import static org.mockito.Mockito.*;
public class CleanUtilTest {
@Test
public void testDeleteFilesOfDir() throws IOException {
File dir = mock(File.class);
File[] listFile = new File[] {
mock(File.class),
mock(File.class),
mock(File.class)
};
when(dir.listFiles()).thenReturn(listFile);
when(listFile[0].toPath()).thenReturn(mock(java.nio.file.Path.class));
when(listFile[0].getAbsolutePath()).thenReturn("file1");
when(listFile[1].toPath()).thenReturn(mock(java.nio.file.Path.class));
when(listFile[1].getAbsolutePath()).thenReturn("file2");
when(listFile[2].toPath()).thenReturn(mock(java.nio.file.Path.class));
when(listFile[2].getAbsolutePath()).thenReturn("file3");
CleanUtil.deleleFilesOfDir(dir.getAbsolutePath(), 1);
verify(listFile[0]).delete();
verify(listFile[1]).delete();
verify(listFile[2]).delete();
}
@Test
public void testDeleteFilesOfDirWithNullList() throws IOException {
File dir = mock(File.class);
when(dir.listFiles()).thenReturn(null);
CleanUtil.deleleFilesOfDir(dir.getAbsolutePath(), 1);
verifyNoMoreInteractions(dir);
}
@Test
public void testDeleteFilesOfDirWithReadAttrException() throws IOException {
File dir = mock(File.class);
File[] listFile = new File[] {
mock(File.class),
mock(File.class),
mock(File.class)
};
when(dir.listFiles()).thenReturn(listFile);
when(listFile[0].toPath()).thenReturn(mock(java.nio.file.Path.class));
when(listFile[0].getAbsolutePath()).thenReturn("file1");
when(listFile[1].toPath()).thenReturn(mock(java.nio.file.Path.class));
when(listFile[1].getAbsolutePath()).thenReturn("file2");
when(listFile[2].toPath()).thenReturn(mock(java.nio.file.Path.class));
when(listFile[2].getAbsolutePath()).thenReturn("file3");
when(Files.readAttributes(any(), eq(BasicFileAttributes.class)))
.thenThrow(new IOException("failed to read attributes"));
CleanUtil.deleleFilesOfDir(dir.getAbsolutePath(), 1);
verify(listFile[0], never()).delete();
verify(listFile[1], never()).delete();
verify(listFile[2], never()).delete();
}
@Test
public void testDeleteOverDueFile() throws IOException {
File file = mock(File.class);
when(file.toPath()).thenReturn(mock(java.nio.file.Path.class));
when(file.getAbsolutePath()).thenReturn("file1");
when(file.lastModified()).thenReturn(System.currentTimeMillis() - 3600 * 1000);
CleanUtil.deleteOverDueFile(1, file, System.currentTimeMillis(), file.lastModified());
verify(file).delete();
}
@Test
public void testDeleteOverDueFileWithNotOverdue() throws IOException {
File file = mock(File.class);
when(file.toPath()).thenReturn(mock(java.nio.file.Path.class));
when(file.getAbsolutePath()).thenReturn("file1");
when(file.lastModified()).thenReturn(System.currentTimeMillis() - 1000);
CleanUtil.deleteOverDueFile(1, file, System.currentTimeMillis(), file.lastModified());
verify(file, never()).delete();
}
@Test
public void testDeleteOverDueFileWithDeleteFailed() throws IOException {
File file = mock(File.class);
when(file.toPath()).thenReturn(mock(java.nio.file.Path.class));
when(file.getAbsolutePath()).thenReturn("file1");
when(file.lastModified()).thenReturn(System.currentTimeMillis() - 3600 * 1000);
when(FileUtils.deleteQuietly(file)).thenReturn(false);
CleanUtil.deleteOverDueFile(1, file, System.currentTimeMillis(), file.lastModified());
verify(file).delete();
}
@Test
public void testDeleteFileWithRuntimeException() throws IOException {
File file = mock(File.class);
when(file.toPath()).thenReturn(mock(java.nio.file.Path.class));
when(file.getAbsolutePath()).thenReturn("file1");
when(Files.readAttributes(any(), eq(BasicFileAttributes.class)))
.thenThrow(new RuntimeException("failed to read attributes"));
CleanUtil.deleteFile(System.currentTimeMillis(), 1, file);
verify(file, never()).delete();
}
@Test
public void testDeleteFileWithIOException() throws IOException {
File file = mock(File.class);
when(file.toPath()).thenReturn(mock(java.nio.file.Path.class));
when(file.getAbsolutePath()).thenReturn("file1");
when(Files.readAttributes(any(), eq(BasicFileAttributes.class)))
.thenThrow(new IOException("failed to read attributes"));
CleanUtil.deleteFile(System.currentTimeMillis(), 1, file);
verify(file, never()).delete();
}
}
```
这里使用了 Mockito 和 JUnit5,其中:
- testDeleteFilesOfDir 方法测试了正常情况下的文件删除;
- testDeleteFilesOfDirWithNullList 方法测试了当目录为空时不进行操作;
- testDeleteFilesOfDirWithReadAttrException 方法测试了读取文件属性时出现异常的情况;
- testDeleteOverDueFile 方法测试了删除过期文件的情况;
- testDeleteOverDueFileWithNotOverdue 方法测试了文件未过期时不进行操作的情况;
- testDeleteOverDueFileWithDeleteFailed 方法测试了删除文件失败的情况;
- testDeleteFileWithRuntimeException 和 testDeleteFileWithIOException 方法测试了读取文件属性时出现异常的情况。
阅读全文
相关推荐
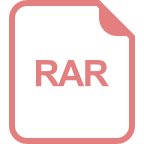
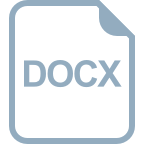
















