c++:读写ini文件(附完整源码)
时间: 2023-07-03 13:02:15 浏览: 83
### 回答1:
以下是使用C语言读写ini文件的完整源代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LENGTH 256
int getValueFromIniFile(const char* filename, const char* section, const char* key, char* value)
{
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file %s\n", filename);
return 0;
}
char currentSection[MAX_LINE_LENGTH] = "";
char line[MAX_LINE_LENGTH];
int foundSection = 0;
while (fgets(line, sizeof(line), file) != NULL) {
// Remove newline character at the end
line[strcspn(line, "\n")] = '\0';
// Check if it is a section
if (line[0] == '[' && line[strlen(line)-1] == ']') {
strncpy(currentSection, line+1, strlen(line)-2);
currentSection[strlen(line)-2] = '\0';
if (strcmp(currentSection, section) == 0) {
foundSection = 1;
} else {
foundSection = 0;
}
} else if (foundSection) {
// Check if it is the desired key
char* delimiter = strchr(line, '=');
if (delimiter != NULL) {
// Split the line into key and value
char currentKey[MAX_LINE_LENGTH] = "";
strncpy(currentKey, line, delimiter - line);
currentKey[delimiter - line] = '\0';
if (strcmp(currentKey, key) == 0) {
strncpy(value, delimiter + 1, MAX_LINE_LENGTH);
value[strcspn(value, "\n")] = '\0'; // Remove newline character
fclose(file);
return 1;
}
}
}
}
fclose(file);
return 0;
}
int setValueToIniFile(const char* filename, const char* section, const char* key, const char* value)
{
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file %s\n", filename);
return 0;
}
// Create a temporary file for writing
FILE* tempFile = fopen("temp.ini", "w");
if (tempFile == NULL) {
printf("Failed to create temporary file\n");
fclose(file);
return 0;
}
char currentSection[MAX_LINE_LENGTH] = "";
char line[MAX_LINE_LENGTH];
int foundSection = 0;
int keyFoundInSection = 0;
int valueWritten = 0;
while (fgets(line, sizeof(line), file) != NULL) {
// Check if it is a section
if (line[0] == '[' && line[strlen(line)-1] == ']') {
strncpy(currentSection, line+1, strlen(line)-2);
currentSection[strlen(line)-2] = '\0';
if (strcmp(currentSection, section) == 0) {
foundSection = 1;
} else {
foundSection = 0;
}
fprintf(tempFile, "%s", line);
} else if (foundSection) {
// Check if it is the desired key
char* delimiter = strchr(line, '=');
if (delimiter != NULL) {
// Split the line into key and value
char currentKey[MAX_LINE_LENGTH] = "";
strncpy(currentKey, line, delimiter - line);
currentKey[delimiter - line] = '\0';
if (strcmp(currentKey, key) == 0) {
fprintf(tempFile, "%s=%s\n", key, value);
keyFoundInSection = 1;
valueWritten = 1;
} else {
fprintf(tempFile, "%s", line);
}
} else {
fprintf(tempFile, "%s", line);
}
} else {
fprintf(tempFile, "%s", line);
}
}
// If the key is not found, append it at the end of the section
if (!keyFoundInSection) {
fprintf(tempFile, "%s=%s\n", key, value);
valueWritten = 1;
}
fclose(file);
fclose(tempFile);
// Replace the original file with the updated temporary file
if (valueWritten) {
remove(filename);
rename("temp.ini", filename);
} else {
remove("temp.ini");
}
return 1;
}
int main()
{
// 读取ini文件
char value[MAX_LINE_LENGTH];
if (getValueFromIniFile("example.ini", "Section1", "Key1", value)) {
printf("Value found: %s\n", value);
} else {
printf("Value not found\n");
}
// 写入ini文件
if (setValueToIniFile("example.ini", "Section1", "Key1", "NewValue")) {
printf("Value updated\n");
} else {
printf("Failed to update value\n");
}
return 0;
}
```
以上代码为一个简单的读写ini文件的示例。通过`getValueFromIniFile`函数可以从指定的ini文件中获取指定的section和key的值,通过`setValueToIniFile`函数可以更新或添加ini文件中指定section和key的值。可根据实际需要修改和使用此代码。请确保在使用前要确保文件存在且有读写权限。
### 回答2:
ini文件是一种常见的文本文件格式,用于存储配置信息。读写ini文件可以通过解析文件的内容来获取配置项的值,也可以通过修改文件中的值来修改配置项。
以下是一个简单的读写ini文件的示例代码:
```python
import configparser
# 创建一个ConfigParser对象
config = configparser.ConfigParser()
# 读取ini文件
config.read('config.ini')
# 获取配置项的值
value = config.get('section_name', 'key_name') # section_name为节名,key_name为键名
# 修改配置项的值
config.set('section_name', 'key_name', 'new_value') # section_name为节名,key_name为键名,new_value为新值
# 保存修改后的ini文件
with open('config.ini', 'w') as configfile:
config.write(configfile)
```
在这个示例代码中,首先通过导入`configparser`模块创建了一个`ConfigParser`对象。然后使用`read()`方法读取了名为`config.ini`的ini文件。
通过调用`get()`方法可以获取`config.ini`文件中`section_name`节下`key_name`键的值。
通过调用`set()`方法可以将`section_name`节下`key_name`键的值修改为`new_value`。
最后,可以使用`write()`方法将修改后的配置信息写入到`config.ini`文件中。
需要注意的是,以上代码只是一个简单的示例,实际使用时需要根据具体的需求和ini文件的结构来进行相应的修改和处理。
### 回答3:
以下是一个使用C语言读写ini文件的完整源码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_SIZE 256
// 读取ini文件
int readIniFile(const char* filename, const char* section, const char* key, char* value) {
FILE* file = fopen(filename, "r");
if (file == NULL) {
printf("无法打开ini文件\n");
return -1;
}
char line[MAX_LINE_SIZE];
char currentSection[MAX_LINE_SIZE];
char currentKey[MAX_LINE_SIZE];
int foundSection = 0;
int foundKey = 0;
while (fgets(line, MAX_LINE_SIZE, file) != NULL) {
// 移除换行符
line[strcspn(line, "\n")] = '\0';
// 处理注释和空行
if (line[0] == '#' || line[0] == '\0') {
continue;
}
// 处理section
if (line[0] == '[') {
if (foundSection) {
printf("找到了指定的section,但没有找到指定的key\n");
return -1;
}
sscanf(line, "[%[^]]", currentSection);
if (strcmp(currentSection, section) == 0) {
foundSection = 1;
}
} else if (foundSection) {
sscanf(line, "%[^= ] = %[^\n]", currentKey, value);
if (strcmp(currentKey, key) == 0) {
foundKey = 1;
break;
}
}
}
fclose(file);
if (foundSection && foundKey) {
return 0;
} else {
printf("没有找到指定的section或key\n");
return -1;
}
}
// 写入ini文件
int writeIniFile(const char* filename, const char* section, const char* key, const char* value) {
FILE* file = fopen(filename, "a");
if (file == NULL) {
printf("无法打开ini文件\n");
return -1;
}
fprintf(file, "\n[%s]\n%s = %s\n", section, key, value);
fclose(file);
return 0;
}
int main() {
char value[MAX_LINE_SIZE];
// 读取ini文件
if (readIniFile("config.ini", "section1", "key1", value) == 0) {
printf("value1: %s\n", value);
}
// 写入ini文件
if (writeIniFile("config.ini", "section2", "key2", "value2") == 0) {
printf("成功写入ini文件\n");
}
return 0;
}
```
以上源码中的readIniFile函数用于读取指定section和key对应的value,writeIniFile函数用于向ini文件中写入新的section和key/value对。
使用main函数作为示例,打开名为"config.ini"的ini文件,并读取名为"section1"的section中的"key1"对应的value,并打印出来。然后向ini文件中写入一个名为"section2"的section,其中包含一个名为"key2"的key和"value2"的value。
相关推荐
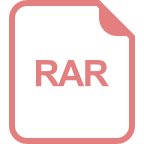














