用C++编写程序:设计一个father类、一个mother类和一个child类,其中child类继承father类和mother类。father类和mother类都包含姓和名两个数据成员,child类仅包含名数据成员。要求一个child类的对象能够输出其父、其母和自己的姓名
时间: 2024-05-16 15:18:18 浏览: 9
以下是对应的C++代码:
```c++
#include <iostream>
using namespace std;
class father {
protected:
string first_name;
string last_name;
public:
father(string fn, string ln) : first_name(fn), last_name(ln) {}
string getFullName() { return first_name + " " + last_name; }
};
class mother {
protected:
string first_name;
string last_name;
public:
mother(string fn, string ln) : first_name(fn), last_name(ln) {}
string getFullName() { return first_name + " " + last_name; }
};
class child : public father, public mother {
private:
string first_name;
public:
child(string fn, string ffn, string fln, string mfn, string mln)
: first_name(fn), father(ffn, fln), mother(mfn, mln) {}
void printName() {
cout << "Father's name: " << father.getFullName() << endl;
cout << "Mother's name: " << mother.getFullName() << endl;
cout << "Child's name: " << first_name << endl;
}
};
int main() {
child c("Tom", "John", "Doe", "Jane", "Smith");
c.printName();
return 0;
}
```
在这个程序中,我们定义了三个类:father、mother和child。father和mother类都包含了一个名字和姓氏,作为其数据成员。child类则继承了father和mother类,并仅包含了一个名字作为其数据成员。
在child类的构造函数中,我们需要调用其父类和母类的构造函数,以初始化其数据成员。在printName函数中,我们调用了父类和母类的getFullName函数,以输出其父母和自己的姓名。
最后,在主函数中,我们创建了一个child对象,并调用了其printName函数,以输出其姓名。输出结果如下:
```
Father's name: John Doe
Mother's name: Jane Smith
Child's name: Tom
```
相关推荐
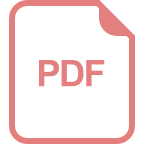












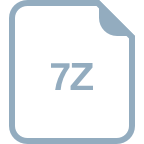
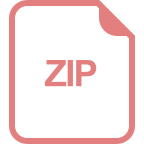
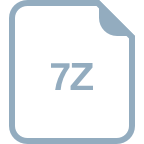
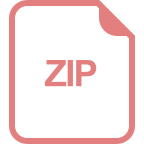