java 根据ipv6/掩码,获取所有ipv6
时间: 2023-12-10 15:42:04 浏览: 261
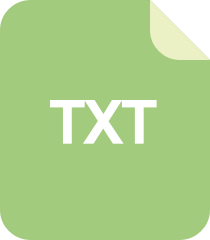
IPv6地址格式校验,压缩,补全,以及进制转换
可以使用 `java.net.InetAddress` 类来实现根据 IPv6 和掩码获取所有 IPv6 的功能。具体的实现过程如下所示:
```java
import java.net.InetAddress;
import java.net.UnknownHostException;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.List;
public class IPv6Utils {
public static List<String> getAllIPv6(String ipv6, int mask) {
List<String> allIPv6 = new ArrayList<>();
try {
InetAddress inetAddress = InetAddress.getByName(ipv6);
byte[] addressBytes = inetAddress.getAddress();
ByteBuffer buffer = ByteBuffer.wrap(addressBytes);
int[] ipv6Bytes = new int[8];
for (int i = 0; i < 8; i++) {
ipv6Bytes[i] = buffer.getShort() & 0xffff;
}
int[] maskBytes = new int[8];
for (int i = 0; i < 8; i++) {
if (mask >= 16) {
maskBytes[i] = 0xffff;
mask -= 16;
} else if (mask > 0) {
maskBytes[i] = (1 << mask) - 1;
mask = 0;
}
}
int[] networkBytes = new int[8];
for (int i = 0; i < 8; i++) {
networkBytes[i] = ipv6Bytes[i] & maskBytes[i];
}
for (int i = networkBytes[0]; i <= (ipv6Bytes[0] | ~maskBytes[0]) & 0xffff; i++) {
for (int j = networkBytes[1]; j <= (ipv6Bytes[1] | ~maskBytes[1]) & 0xffff; j++) {
for (int k = networkBytes[2]; k <= (ipv6Bytes[2] | ~maskBytes[2]) & 0xffff; k++) {
for (int l = networkBytes[3]; l <= (ipv6Bytes[3] | ~maskBytes[3]) & 0xffff; l++) {
for (int m = networkBytes[4]; m <= (ipv6Bytes[4] | ~maskBytes[4]) & 0xffff; m++) {
for (int n = networkBytes[5]; n <= (ipv6Bytes[5] | ~maskBytes[5]) & 0xffff; n++) {
for (int o = networkBytes[6]; o <= (ipv6Bytes[6] | ~maskBytes[6]) & 0xffff; o++) {
for (int p = networkBytes[7]; p <= (ipv6Bytes[7] | ~maskBytes[7]) & 0xffff; p++) {
StringBuilder sb = new StringBuilder();
sb.append(String.format("%x", i)).append(":")
.append(String.format("%x", j)).append(":")
.append(String.format("%x", k)).append(":")
.append(String.format("%x", l)).append(":")
.append(String.format("%x", m)).append(":")
.append(String.format("%x", n)).append(":")
.append(String.format("%x", o)).append(":")
.append(String.format("%x", p));
allIPv6.add(sb.toString());
}
}
}
}
}
}
}
}
} catch (UnknownHostException e) {
e.printStackTrace();
}
return allIPv6;
}
}
```
其中,输入参数为 IPv6 地址和掩码长度,返回结果为符合该掩码的所有 IPv6 地址的列表。
阅读全文
相关推荐







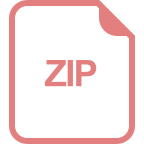
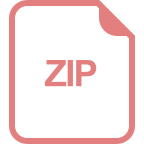
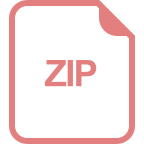
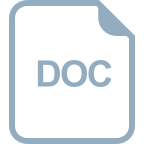
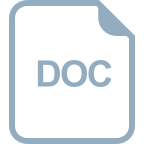



