基于C++,使用QT,创建6个check box,分别为温度、时间、长度、高度、宽度、大小,选中其中任意,在widget中显示出其名称和取值范围,并且可以输入范围内的数据
时间: 2024-03-09 21:51:43 浏览: 14
好的,您可以按照以下步骤在 QT 中用 C++ 实现该功能:
1. 在 QT Designer 中创建一个新的窗口,然后向窗口中添加 6 个 QCheckBox 控件,分别设置其名称为 温度、时间、长度、高度、宽度、大小。
2. 在 QT Creator 中打开该窗口的 UI 文件,在头文件中添加以下代码:
```c++
#include <QCheckBox>
#include <QLabel>
#include <QLineEdit>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
QCheckBox *temp_check_box;
QCheckBox *time_check_box;
QCheckBox *length_check_box;
QCheckBox *height_check_box;
QCheckBox *width_check_box;
QCheckBox *size_check_box;
QLabel *range_label;
QLineEdit *value_edit;
private slots:
void show_range();
};
```
在 MainWindow 类中,我们声明了 6 个 QCheckBox 控件,一个 QLabel 控件和一个 QLineEdit 控件,用于显示范围和输入数据。我们还声明了一个名为 show_range 的私有槽函数,该函数将在用户单击任何一个 QCheckBox 控件时被调用。
3. 在源文件中,实现 MainWindow 类的构造函数和析构函数:
```c++
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
temp_check_box = new QCheckBox("温度", this);
temp_check_box->move(20, 20);
connect(temp_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
time_check_box = new QCheckBox("时间", this);
time_check_box->move(20, 50);
connect(time_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
length_check_box = new QCheckBox("长度", this);
length_check_box->move(20, 80);
connect(length_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
height_check_box = new QCheckBox("高度", this);
height_check_box->move(20, 110);
connect(height_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
width_check_box = new QCheckBox("宽度", this);
width_check_box->move(20, 140);
connect(width_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
size_check_box = new QCheckBox("大小", this);
size_check_box->move(20, 170);
connect(size_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
range_label = new QLabel(this);
range_label->move(20, 200);
value_edit = new QLineEdit(this);
value_edit->setGeometry(20, 230, 200, 30);
value_edit->setEnabled(false);
}
MainWindow::~MainWindow()
{
delete temp_check_box;
delete time_check_box;
delete length_check_box;
delete height_check_box;
delete width_check_box;
delete size_check_box;
delete range_label;
delete value_edit;
}
```
在构造函数中,我们创建了 6 个 QCheckBox 控件,并将它们移动到合适的位置,然后将它们的 clicked 信号连接到 show_range 槽函数上。我们还创建了一个 QLabel 控件和一个 QLineEdit 控件,分别用于显示范围和输入数据。
在析构函数中,我们销毁这些控件,以释放它们占用的内存。
4. 在源文件中,实现 show_range 槽函数:
```c++
void MainWindow::show_range()
{
QString range;
QString unit;
QCheckBox *check_box = qobject_cast<QCheckBox *>(sender());
if (check_box == temp_check_box) {
range = "-100 to 100";
unit = "℃";
} else if (check_box == time_check_box) {
range = "0 to 24";
unit = "h";
} else if (check_box == length_check_box) {
range = "0 to 1000";
unit = "m";
} else if (check_box == height_check_box) {
range = "0 to 1000";
unit = "m";
} else if (check_box == width_check_box) {
range = "0 to 1000";
unit = "m";
} else if (check_box == size_check_box) {
range = "0 to 1000000";
unit = "m³";
}
range_label->setText(check_box->text() + "范围:" + range + unit);
value_edit->setEnabled(true);
}
```
在 show_range 槽函数中,我们首先获取当前被单击的 QCheckBox 控件的指针,然后根据其名称设置范围和单位。最后,我们将范围和单位显示在 QLabel 控件中,并启用 QLineEdit 控件。
5. 在 main 函数中,创建一个 MainWindow 对象,并显示窗口:
```c++
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
这样,当用户单击任何一个 QCheckBox 控件时,程序都会在 QLabel 中显示其名称和取值范围,并在 QLineEdit 中添加一个输入框以便用户输入数据。
完整的源代码如下:
mainwindow.h:
```c++
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QCheckBox>
#include <QLabel>
#include <QLineEdit>
#include <QMainWindow>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
QCheckBox *temp_check_box;
QCheckBox *time_check_box;
QCheckBox *length_check_box;
QCheckBox *height_check_box;
QCheckBox *width_check_box;
QCheckBox *size_check_box;
QLabel *range_label;
QLineEdit *value_edit;
private slots:
void show_range();
};
#endif // MAINWINDOW_H
```
mainwindow.cpp:
```c++
#include "mainwindow.h"
#include <QApplication>
#include <QCheckBox>
#include <QLabel>
#include <QLineEdit>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
temp_check_box = new QCheckBox("温度", this);
temp_check_box->move(20, 20);
connect(temp_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
time_check_box = new QCheckBox("时间", this);
time_check_box->move(20, 50);
connect(time_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
length_check_box = new QCheckBox("长度", this);
length_check_box->move(20, 80);
connect(length_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
height_check_box = new QCheckBox("高度", this);
height_check_box->move(20, 110);
connect(height_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
width_check_box = new QCheckBox("宽度", this);
width_check_box->move(20, 140);
connect(width_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
size_check_box = new QCheckBox("大小", this);
size_check_box->move(20, 170);
connect(size_check_box, SIGNAL(clicked()), this, SLOT(show_range()));
range_label = new QLabel(this);
range_label->move(20, 200);
value_edit = new QLineEdit(this);
value_edit->setGeometry(20, 230, 200, 30);
value_edit->setEnabled(false);
}
MainWindow::~MainWindow()
{
delete temp_check_box;
delete time_check_box;
delete length_check_box;
delete height_check_box;
delete width_check_box;
delete size_check_box;
delete range_label;
delete value_edit;
}
void MainWindow::show_range()
{
QString range;
QString unit;
QCheckBox *check_box = qobject_cast<QCheckBox *>(sender());
if (check_box == temp_check_box) {
range = "-100 to 100";
unit = "℃";
} else if (check_box == time_check_box) {
range = "0 to 24";
unit = "h";
} else if (check_box == length_check_box) {
range = "0 to 1000";
unit = "m";
} else if (check_box == height_check_box) {
range = "0 to 1000";
unit = "m";
} else if (check_box == width_check_box) {
range = "0 to 1000";
unit = "m";
} else if (check_box == size_check_box) {
range = "0 to 1000000";
unit = "m³";
}
range_label->setText(check_box->text() + "范围:" + range + unit);
value_edit->setEnabled(true);
}
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
相关推荐
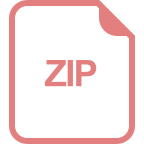
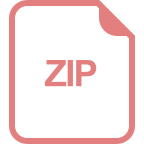
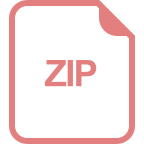














