写一个vgg16分析fer2013数据集的代码
时间: 2023-12-01 10:56:15 浏览: 35
好的,以下是使用VGG16模型对FER2013数据集进行图像分类的代码(Python):
```
# 导入必要的库
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Dropout, Flatten
from tensorflow.keras.layers import Conv2D, MaxPooling2D
from tensorflow.keras.models import Model
# 定义模型架构
def vgg16(input_shape, num_classes):
inputs = Input(shape=input_shape)
# Block 1
x = Conv2D(64, (3, 3), activation='relu', padding='same', name='block1_conv1')(inputs)
x = Conv2D(64, (3, 3), activation='relu', padding='same', name='block1_conv2')(x)
x = MaxPooling2D((2, 2), strides=(2, 2), name='block1_pool')(x)
# Block 2
x = Conv2D(128, (3, 3), activation='relu', padding='same', name='block2_conv1')(x)
x = Conv2D(128, (3, 3), activation='relu', padding='same', name='block2_conv2')(x)
x = MaxPooling2D((2, 2), strides=(2, 2), name='block2_pool')(x)
# Block 3
x = Conv2D(256, (3, 3), activation='relu', padding='same', name='block3_conv1')(x)
x = Conv2D(256, (3, 3), activation='relu', padding='same', name='block3_conv2')(x)
x = Conv2D(256, (3, 3), activation='relu', padding='same', name='block3_conv3')(x)
x = MaxPooling2D((2, 2), strides=(2, 2), name='block3_pool')(x)
# Block 4
x = Conv2D(512, (3, 3), activation='relu', padding='same', name='block4_conv1')(x)
x = Conv2D(512, (3, 3), activation='relu', padding='same', name='block4_conv2')(x)
x = Conv2D(512, (3, 3), activation='relu', padding='same', name='block4_conv3')(x)
x = MaxPooling2D((2, 2), strides=(2, 2), name='block4_pool')(x)
# Block 5
x = Conv2D(512, (3, 3), activation='relu', padding='same', name='block5_conv1')(x)
x = Conv2D(512, (3, 3), activation='relu', padding='same', name='block5_conv2')(x)
x = Conv2D(512, (3, 3), activation='relu', padding='same', name='block5_conv3')(x)
x = MaxPooling2D((2, 2), strides=(2, 2), name='block5_pool')(x)
# 加载预训练的VGG16权重
vgg16 = tf.keras.applications.VGG16(include_top=False, weights='imagenet')
vgg16_output = vgg16(x)
# 在顶部添加自己的全连接层
x = Flatten(name='flatten')(vgg16_output)
x = Dense(4096, activation='relu', name='fc1')(x)
x = Dropout(0.5)(x)
x = Dense(4096, activation='relu', name='fc2')(x)
x = Dropout(0.5)(x)
outputs = Dense(num_classes, activation='softmax', name='predictions')(x)
model = Model(inputs=inputs, outputs=outputs, name='VGG16')
return model
# 加载FER2013数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.fer2013.load_data()
# 预处理数据
x_train = x_train.reshape(x_train.shape[0], 48, 48, 1)
x_test = x_test.reshape(x_test.shape[0], 48, 48, 1)
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
x_train /= 255
x_test /= 255
num_classes = 7
# 训练模型
model = vgg16((48, 48, 1), num_classes)
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
model.fit(x_train, tf.keras.utils.to_categorical(y_train, num_classes), batch_size=128, epochs=20, validation_data=(x_test, tf.keras.utils.to_categorical(y_test, num_classes)))
# 评估模型
score = model.evaluate(x_test, tf.keras.utils.to_categorical(y_test, num_classes), verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
```
此代码建立了一个对FER2013数据集进行分类的VGG16模型,并对模型进行训练和评估。
相关推荐
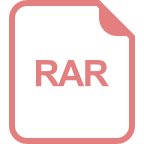














