qt用dijkstra算最短路径,给出程序
时间: 2024-05-07 21:15:51 浏览: 91
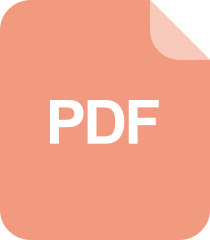
基于dijkstra算法的最短路径的求解
以下是使用Qt实现Dijkstra算法的最短路径程序示例:
```cpp
#include <QtCore/QCoreApplication>
#include <QtCore>
#include <QtGui>
#include <iostream>
#include <set>
#include <vector>
#include <map>
#include <algorithm>
using namespace std;
class Edge
{
public:
int from, to, weight;
};
class Node
{
public:
int id;
int distance;
int prev;
};
bool operator<(const Node& lhs, const Node& rhs)
{
return lhs.distance < rhs.distance;
}
class Graph
{
public:
Graph(vector<Edge>& edges)
{
for (auto& edge : edges)
{
adjacencyList[edge.from].push_back(edge);
}
}
vector<int> dijkstra(int start, int end)
{
map<int, Node> nodes;
for (auto& pair : adjacencyList)
{
int id = pair.first;
nodes[id].id = id;
nodes[id].distance = INT_MAX;
nodes[id].prev = -1;
}
nodes[start].distance = 0;
set<Node> queue;
for (auto& pair : nodes)
{
queue.insert(pair.second);
}
while (!queue.empty())
{
Node current = *queue.begin();
queue.erase(queue.begin());
if (current.id == end) break;
for (auto& edge : adjacencyList[current.id])
{
int neighbor = edge.to;
int distance = current.distance + edge.weight;
if (distance < nodes[neighbor].distance)
{
queue.erase(nodes[neighbor]);
nodes[neighbor].distance = distance;
nodes[neighbor].prev = current.id;
queue.insert(nodes[neighbor]);
}
}
}
vector<int> path;
for (int id = end; id != -1; id = nodes[id].prev)
{
path.push_back(id);
}
reverse(path.begin(), path.end());
return path;
}
private:
map<int, vector<Edge>> adjacencyList;
};
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
vector<Edge> edges{ {0, 1, 10}, {0, 4, 5}, {1, 2, 1}, {1, 4, 2}, {2, 3, 4}, {3, 2, 6}, {4, 1, 3}, {4, 2, 9}, {4, 3, 2} };
Graph graph(edges);
vector<int> path = graph.dijkstra(0, 3);
for (auto& id : path)
{
cout << id << " ";
}
return a.exec();
}
```
该程序使用了STL中的set和map容器,以及算法库中的reverse和sort函数,因此需要添加相关头文件。在此基础上,定义了边(Edge)和节点(Node)类,并使用邻接表(adjacencyList)表示图。在dijkstra函数中,使用了set容器来存储节点信息,以便按照距离排序。最后,程序打印出了从0到3的最短路径。
阅读全文
相关推荐





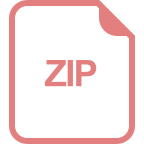
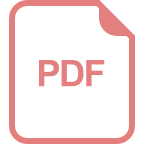
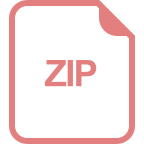
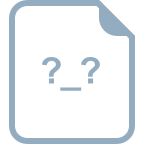
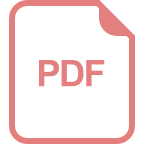
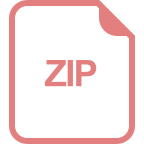
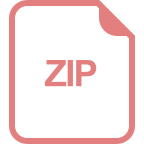