quasi-random用python实现
时间: 2024-05-04 22:21:03 浏览: 115
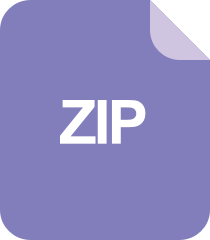
quasistatic-parallel-2D
实现 quasi-random 的一种常见方法是使用 Sobol 序列。以下是一个使用 Python 和 NumPy 库实现 Sobol 序列的示例代码:
```python
import numpy as np
# Define generator matrix
V = np.array([[1, 0, 0, 0, 0, 0, 0, 0, 0],
[1, 1/2, 0, 0, 0, 0, 0, 0, 0],
[1, 1/2, 1/3, 0, 0, 0, 0, 0, 0],
[1, 1/2, 1/3, 1/4, 0, 0, 0, 0, 0],
[1, 1/2, 1/3, 1/4, 1/5, 0, 0, 0, 0],
[1, 1/2, 1/3, 1/4, 1/5, 1/6, 0, 0, 0],
[1, 1/2, 1/3, 1/4, 1/5, 1/6, 1/7, 0, 0],
[1, 1/2, 1/3, 1/4, 1/5, 1/6, 1/7, 1/8, 0],
[1, 1/2, 1/3, 1/4, 1/5, 1/6, 1/7, 1/8, 1/9]])
# Initialize previous direction numbers
prev_n = np.zeros(9)
def sobol(n):
global prev_n
# XOR with direction numbers
xor = np.bitwise_xor(int(n), np.arange(prev_n.size, dtype=np.uint32))
# Convert to binary
bits = np.unpackbits(xor.view(np.uint8))
# Compute next direction numbers
q = np.zeros(bits.size)
for i in range(bits.size):
q[i] = np.sum(V[i, :i+1] * bits[:i+1][::-1])
prev_n = q
# Normalize to [0, 1)
return q / 2**bits.size
# Generate 10 quasi-random points
for i in range(10):
print(sobol(i))
```
请注意,此示例代码只生成了一个维度的 Sobol 序列。要生成多个维度,请将方向数矩阵 V 扩展为相应的维度,并将输出的 q 合并为一个矢量。
阅读全文
相关推荐
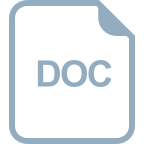
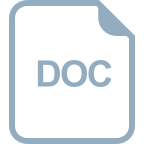
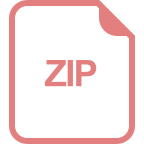
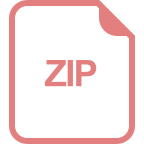
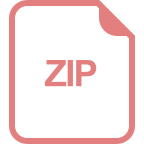
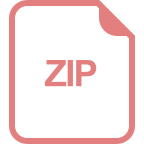
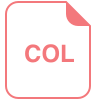
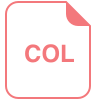
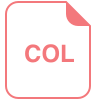



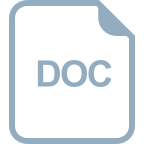
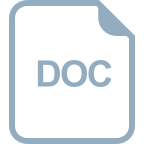
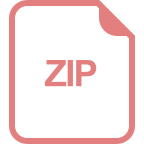
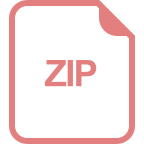
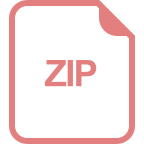