深度应用NLP技术:Python数据挖掘中的文本分析
发布时间: 2024-08-31 22:19:00 阅读量: 244 订阅数: 96 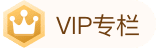
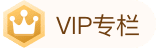
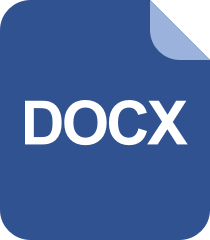
数据挖掘与数据分析应用案例 数据挖掘算法实践 基于Python的卷积神经网络的文本分类.docx
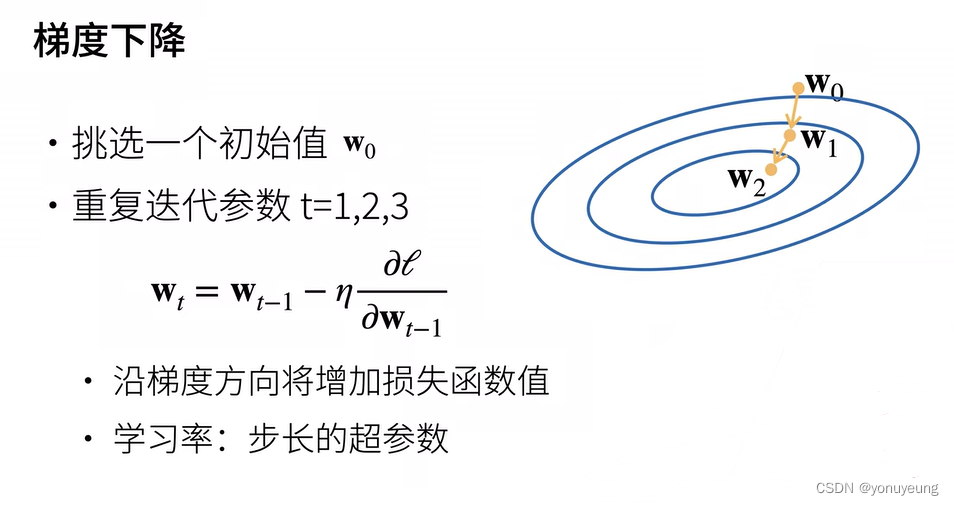
# 1. Python数据挖掘与文本分析概述
数据挖掘和文本分析是当今信息技术领域中的热点议题。Python,作为一种高级编程语言,因其简洁、易读和强大的库支持,在数据挖掘和文本分析方面表现出色。本章将概述Python在数据挖掘与文本分析中的应用,为读者提供一个全面的初步认识。
## 1.1 数据挖掘的概念
数据挖掘,是使用统计学、模式识别和机器学习等方法从大量数据中提取或“挖掘”有价值信息和知识的过程。它在商业智能、市场分析、生物信息学等领域有着广泛的应用。
## 1.2 文本分析的重要性
文本分析则是数据挖掘的一个子领域,专注于从文本数据中提取有用信息。随着互联网和移动设备的普及,文本数据量急剧增加,文本分析的实用价值日益凸显。它可以帮助企业了解顾客情感、市场趋势,甚至支持安全监控等任务。
## 1.3 Python在文本分析中的作用
Python拥有多样的数据处理库,如Pandas用于数据分析、NumPy用于数值计算等,而NLTK和spaCy等库则为文本分析提供了丰富的工具和算法。这些库简化了从数据准备到模型构建的整个流程,使得文本分析变得更加高效和直观。
通过引入具体的应用案例和代码片段,下一章将详细介绍文本分析的基础理论与Python的具体应用。
# 2. 文本分析的基础理论
## 2.1 自然语言处理(NLP)的入门知识
### 2.1.1 NLP的定义与发展
自然语言处理(Natural Language Processing,NLP)是计算机科学、人工智能和语言学领域的一个交叉领域,其目标是构建能够理解和生成人类语言的算法和模型。在人类的日常沟通中,自然语言扮演了重要的角色,然而,对机器来说,理解自然语言的细微差别却是极具挑战性的。NLP技术的发展让机器能够处理、理解和解释人类语言,使得人机交互变得更加自然和高效。
NLP的起源可以追溯到20世纪50年代,随着计算机技术的兴起,研究者开始尝试让计算机理解和生成语言。早期的NLP研究集中在语言的结构分析上,比如句法树的构建。随着时间的推移,NLP开始与统计学方法结合,尤其是在20世纪90年代之后,机器学习的引入极大地推动了NLP技术的进步。近年来,深度学习的兴起进一步加速了NLP的发展,使模型能在大量数据上学习复杂的语言模式,从而在各种NLP任务上达到前所未有的性能。
### 2.1.2 NLP中的核心概念与挑战
在深入NLP领域时,有几个核心概念是必须理解的。首先是“语义”,它涉及语言背后的意义和意图的理解。其次是“语境”,即语言使用的情境,对于理解语义至关重要。最后是“语言的歧义”,这是NLP面临的最大挑战之一,包括词义的多样性(多义词)和结构的多样性(同一句话可能有多种语法结构)。
NLP中的挑战还包括但不限于:
- **语言的复杂性**:自然语言充满歧义和不规则性,如俚语、双关语和隐喻。
- **资源的不均衡**:对于资源丰富的语言(如英语),数据和研究较多,而对于资源稀缺语言则较少。
- **计算效率**:处理大规模文本数据需要高效的算法和模型,以保证实时性和资源消耗在可接受范围内。
- **跨领域适应性**:模型需要能适应不同的领域和风格,例如从文学文本到科技文章。
理解这些核心概念和挑战对于在NLP领域进行深入研究和实践至关重要。下一节将详细介绍文本分析的基本流程。
## 2.2 文本分析的基本流程
### 2.2.1 数据清洗与预处理
在进行文本分析之前,数据清洗与预处理是必不可少的一步。这一步骤的目的是确保分析数据的质量,去除噪声,使文本对分析模型更加友好。数据清洗包括去除无关内容(如HTML标签、特殊符号)、转换大小写、纠正拼写错误、去除停用词(如“的”、“是”、“在”等常见但对文本分析意义不大的词汇)等。预处理则可能包括词干提取(提取单词的词根形式)、词形还原(将词汇还原为基本形式)等。
一个典型的文本清洗与预处理流程示例:
```python
import nltk
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
# 假定已经安装了NLTK数据包,并导入了需要的库
nltk.download('stopwords')
nltk.download('wordnet')
nltk.download('omw-1.4')
# 示例文本
text = "NLTK is a leading platform for building Python programs to work with human language data."
# 转换为小写
text = text.lower()
# 移除标点符号
text = "".join([char for char in text if char.isalnum() or char.isspace()])
# 分词
words = text.split()
# 移除停用词
stop_words = set(stopwords.words('english'))
filtered_words = [word for word in words if word not in stop_words]
# 词形还原
lemmatizer = WordNetLemmatizer()
lemmatized_words = [lemmatizer.lemmatize(word) for word in filtered_words]
# 构建预处理后的文本
preprocessed_text = " ".join(lemmatized_words)
```
### 2.2.2 分词与词性标注
分词(Tokenization)是将文本切割成单词或其他有意义的元素(称为“tokens”)。对于英语等以空格分隔的语言来说,分词相对简单。但中文等连续书写且没有明显分隔符的语言则需要更复杂的分词方法。词性标注(Part-of-Speech Tagging)则是给分词后的每个单词标记词性(名词、动词等)。
```python
from nltk.tokenize import word_tokenize
from nltk import pos_tag
# 示例文本
text = "NLTK is a leading platform for building Python programs to work with human language data."
# 分词
tokens = word_tokenize(text)
# 词性标注
tagged_tokens = pos_tag(tokens)
print(tagged_tokens)
```
### 2.2.3 语义分析与情感分析
语义分析旨在理解文本的含义,它超越了简单的词法和句法分析,试图挖掘文本的真实意图。例如,通过解析句子结构和语境来确定“张三打了李四”中张三和李四的关系。情感分析(Sentiment Analysis)则是NLP的一个应用实例,它尝试识别和分类文本中的主观信息,比如从评论中判断出正面或负面情绪。
情感分析的代码示例:
```python
from textblob import TextBlob
# 示例文本
text = "I love this product!"
# 使用TextBlob进行情感分析
blob = TextBlob(text)
sentiment = blob.sentiment
print("Polarity:", sentiment.polarity)
print("Subjectivity:", sentiment.subjectivity)
```
通过上述步骤,文本分析从原始数据中提取有用信息,为进一步的分析和应用打下基础。接下来,我们将探讨文本分析中常用的模型与算法。
# 3. Python在文本分析中的应用实践
## 3.1 Python文本分析库的使用
### 3.1.1 NLTK库的介绍与应用
自然语言处理工具包(Natural Language Toolkit,NLTK)是一个用于符号和统计自然语言处理的Python库。NLTK包含用于处理文本和构建自然语言处理系统的模块和程序库。它提供了易于使用的接口、丰富的文档和教程,非常适合于教学和研究。
NLTK的主要特点如下:
- **语料库和词汇资源**:提供了包括句子分词器、词性标注器、句法解析器等在内的多种自然语言处理工具。
- **预处理工具**:支持文本清洗、分词、标注等基本文本处理任务。
- **分类和标注**:拥有文本分类、标注和解析模块。
- **性能评估**:能够进行准确度测试、交叉验证和混淆矩阵生成等。
- **数据挖掘**:支持发现词和短语之间的关系、相似度计算、信息检索等。
#### 使用NLTK构建一个简单的文本分类器
```python
import nltk
from nltk.corpus import movie_reviews
from nltk.stem import WordNetLemmatizer
from nltk import NaiveBayesClassifier
from nltk.classify.util import accuracy
# 定义一个函数来转换给定的文档为单词的特征集
def document_features(document):
lemmatizer = WordNetLemmatizer()
return {word: True for word in nltk.word_tokenize(document.lower())}
# 加载电影评论语料集
nltk.download('movie_reviews')
documents = [(list(movie_reviews.words(fileid)), category)
for category in movie_reviews.categories()
for fileid in movie_reviews.fileids(category)]
# 为我们的分类器创建一个特征集
features = [(document_features(d), c) for (d,c) in documents]
# 划分训练集和测试集
train_set, test_set = features[100:], features[:100]
# 创建一个朴素贝叶斯分类器
classifier = NaiveBayesClassifier.train(train_set)
# 测试分类器准确度
print('Accuracy is', accuracy(classifier, test_set))
```
在上述代码中,我们首先导入了`nltk`库,然后加载了NLTK自带的电影评论数据集,接着定义了一个特征提取函数`document_features`。然后我们创建了训练集和测试集,并使用朴素贝叶斯分类器进行训练。最后,我们测试了分类器在测试集上的准确度。
### 3.1.2 spaCy库的介绍与应用
spaCy是一个用于高级自然语言处理的Python库,专为生产环境设计。它支持多种语言,并且性能优秀,非常适合处理大型文档。
spaCy的特点包括:
- **高效的处理速度**:特别优化用于处理大型文本的性能。
- **预训练模型**:提供了多种语言的预训练模型,支
0
0
相关推荐
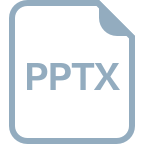
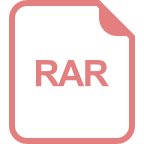
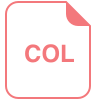
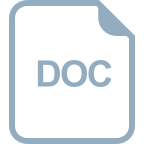
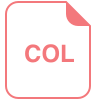
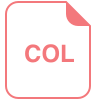
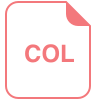
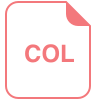