深入挖掘:Python数据预处理技术的终极指南
发布时间: 2024-08-31 22:04:23 阅读量: 298 订阅数: 96 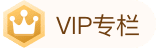
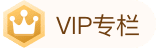
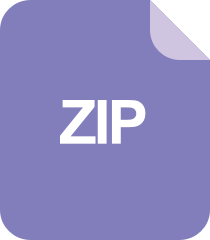
PyDataPreprocessing:《 Python数据预处理技术与实践》源码下载
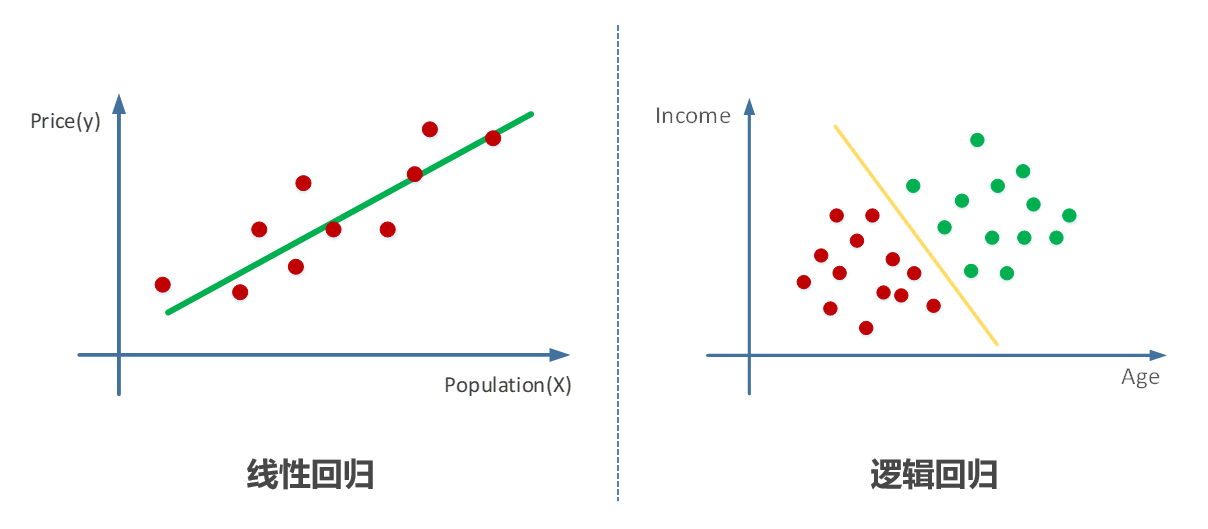
# 1. 数据预处理的基础概念
在数据分析和机器学习领域,数据预处理是至关重要的一步。它通常涉及将原始数据转换成适合模型处理的格式,目的是提高数据质量,增强模型的性能。数据预处理涵盖一系列技术,包括数据清洗、特征工程、数据集划分等,这些技术有助于减少噪声、处理缺失和异常值,提取和选择有用的特征,以及为模型训练准备数据集。了解和掌握数据预处理的基本概念和方法对于任何数据科学项目的成功都至关重要。在接下来的章节中,我们将深入探讨数据预处理的各个方面,包括具体的技术、操作流程和实战案例。
# 2. ```
# 第二章:数据清洗技术详解
数据清洗作为数据预处理的一个重要环节,是确保数据质量的关键步骤。在这一章节中,我们将深入探讨数据清洗中的核心技术,包括对缺失值、异常值的处理,数据类型的转换,以及数据标准化与归一化的方法。
## 2.1 缺失值处理
### 2.1.1 缺失值识别方法
缺失值是数据集中常见的问题之一,它可能是由于数据收集不完整、数据录入错误或者数据传输过程中的丢失等原因造成的。识别缺失值是处理缺失值的第一步,有多种方法可以用来识别数据集中的缺失值。
- **观察数据集**: 通过观察数据集,检查是否有空格、问号或“NA”等标记。Pandas库中提供了`isnull()`和`notnull()`函数,可以用来检测数据集中的缺失值。
- **描述性统计**: 使用`describe()`函数可以快速得到数据集的统计描述,其中会明确显示数据集中的缺失值数量。
- **可视化分析**: 利用条形图、热力图等可视化手段,可以直观地识别出数据集中缺失值的分布情况。
```python
import pandas as pd
# 加载数据集
df = pd.read_csv('data.csv')
# 检测缺失值
missing_values = df.isnull().sum()
# 输出缺失值统计
print(missing_values)
```
在上述代码中,`isnull()`函数返回一个布尔型DataFrame,其中True表示相应位置的值是缺失的。通过对这个布尔型DataFrame进行求和,我们可以得到每个特征缺失值的数量。
### 2.1.2 缺失值填充策略
在识别出缺失值之后,接下来需要根据实际情况采取合适的填充策略。
- **删除缺失值**: 如果数据集很大,且缺失值占比小,可以考虑直接删除含有缺失值的行或列。
- **填充缺失值**: 可以使用某个统计值(如平均值、中位数、众数)填充,或者使用预测模型来预测缺失值。
```python
# 使用列的均值填充缺失值
df['column'] = df['column'].fillna(df['column'].mean())
```
在上述代码中,`fillna()`函数用于填充缺失值,这里采用的是列均值填充策略。根据数据的特性,我们也可以选择中位数、众数等其他统计值进行填充。
## 2.2 异常值检测与处理
### 2.2.1 异常值检测技术
异常值是数据集中那些不符合预期模式或行为的值。这些值可能是由于错误、噪声或者真实的极端情况造成的。
- **统计方法**: 使用标准差、四分位数范围等统计方法来定义异常值。
- **基于模型的方法**: 使用聚类分析、密度估计等模型来识别异常值。
```python
# 使用四分位数检测异常值
Q1 = df['column'].quantile(0.25)
Q3 = df['column'].quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
# 检测异常值
outliers = df[(df['column'] < lower_bound) | (df['column'] > upper_bound)]
```
上述代码片段中,我们计算了指定列的四分位数和四分位数范围(IQR),然后定义了异常值的上下界。任何超出这个范围的值被认为是异常值。
### 2.2.2 异常值处理方法
处理异常值的方法包括但不限于以下几种:
- **删除异常值**: 如果异常值不影响研究目标,可以考虑直接删除这些值。
- **修正异常值**: 如果异常值是由于明显的错误造成的,可以尝试使用合适的方法修正这些值。
- **保留异常值**: 如果异常值是数据集的重要部分,可以考虑保留这些值,或者在分析时进行特殊处理。
```python
# 删除异常值所在行
df_cleaned = df[~((df['column'] < lower_bound) | (df['column'] > upper_bound))]
```
在此代码片段中,我们使用了布尔索引来过滤掉异常值所在的行,从而得到一个清理后的数据集。
## 2.3 数据类型转换
### 2.3.1 字符串与数值转换
在数据集中,某些特征可能是以字符串形式存在的,但为了模型训练需要转换成数值型。常见的字符串与数值转换包括编码技术、标签化等。
```python
# 将字符串类别转换为数值
df['categorical_column'] = df['categorical_column'].astype('category').cat.codes
```
在该代码片段中,我们使用了Pandas的`astype`方法和`cat.codes`属性来将分类数据转换为数值型。这是一种非常常见的类型转换方式。
### 2.3.2 分类数据的编码技术
分类数据的编码通常涉及到将类别型特征转换为模型能够理解的数值型特征。这包括:
- **标签编码(Label Encoding)**: 将每个类别分配一个唯一的整数。
- **独热编码(One-hot Encoding)**: 为每个类别创建一个新的二进制特征列。
- **二进制编码(Binary Encoding)**: 将标签编码转换成二进制形式。
```python
from sklearn.preprocessing import OneHotEncoder
# 创建独热编码器实例
enc = OneHotEncoder()
# 将分类数据转换为独热编码
encoded_data = enc.fit_transform(df[['categorical_column']])
```
在上述代码中,我们使用了Scikit-learn库中的`OneHotEncoder`类来将分类数据转换为独热编码格式。这是一种在处理分类数据时常用的技术。
## 2.4 数据标准化与归一化
### 2.4.1 标准化方法
标准化是将特征值缩放到一个标准正态分布的过程,即均值为0,标准差为1。标准化对于许多机器学习算法来说是重要的预处理步骤。
```python
from sklearn.preprocessing import StandardScaler
# 创建标准化器实例
scaler = StandardScaler()
# 对数据集进行标准化
df_scaled = scaler.fit_transform(df[['numeric_column']])
```
上述代码使用了Scikit-learn的`StandardScaler`类对指定数值列进行标准化处理。标准化之后的数据能够帮助模型更好地收敛。
### 2.4.2 归一化技巧
归一化是将特征值缩放到一个小的特定区间,通常是[0, 1],或者[-1, 1]。在一些特定算法中,如神经网络和k-最近邻,归一化是非常关键的。
```python
from sklearn.preprocessing import MinMaxScaler
# 创建归一化器实例
min_max_scaler = MinMaxScaler()
# 对数据集进行归一化处理
df_normalized = min_max_scaler.fit_transform(df[['numeric_column']])
```
在上述代码中,使用了Scikit-learn的`MinMaxScaler`类对指定的数值列进行归一化处理。归一化之后的数据分布在一个固定的区间内,有助于算法的稳定性和收敛性。
在本章节中,我们详细讲解了数据清洗过程中涉及的多项关键技术,包括缺失值处理、异常值检测与处理、数据类型转换、数据标准化与归一化。理解并掌握这些技术对于保证数据质量至关重要,并且它们将在后续的数据分析和建模过程中发挥关键作用。
```
# 3. 数据特征工程技术
## 3.1 特征提取基础
### 3.1.1 主成分分析(PCA)
主成分分析(PCA)是一种统计方法,通过正交变换将可能相关的变量转换成一组线性不相关的变量,称为主成分。在多变量分析中,它常用于降维,即减少数据集中的特征数量,同时尽可能保留数据的原始结构和信息。
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.decomposition import PCA
from sklearn.preprocessing import StandardScaler
# 假设我们有以下数据集
X = np.array([[1, 2], [3, 4], [5, 6]])
# 对数据进行标准化处理
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# 应用PCA
pca = PCA(n_components=2)
X_pca = pca.fit_transform(X_scaled)
# 显示原始数据和PCA后的数据
plt.scatter(X_scaled[:, 0], X_scaled[:, 1], label='Original Data')
plt.scatter(X_pca[:, 0], X_pca[:, 1], color='r', label='PCA Data')
plt.legend()
plt.show()
# 输出主成分
print('主成分方向:', ***ponents_)
print('主成分方差解释率:', pca.explained_variance_ratio_)
```
在上述代码中,我们首先对数据进行了标准化处理,以确保PCA可以正确地找到数据的主成分。然后,我们初始化PCA并拟合数据,将原始数据集转换为新的主成分表示。通过输出主成分方向和方差解释率,我们可以理解PCA在数据降维中如何工作以及每个主成分的重要性。
### 3.1.2 线性判别分析(LDA)
线性判别分析(LDA)是一种分类算法,用于在数据集中区分不同类别的数据点。LDA尝试找到一个线性组合的特征,这个线性组合可以最大化不同类之间的距离,并同时最小化同类之间的距离。这使得LDA不仅用于特征提取,还用于分类。
```python
from sklearn.discriminant_analysis import LinearDiscriminantAnalysis
# 假设X和Y分别代表不同的类别数据
X = np.array([[1, 2], [3, 4], [5, 6]])
Y = np.array([1, 2, 1])
# 应用LDA
lda = LinearDiscriminantAnalysis(n_components=1)
X_lda = lda.fit_transform(X, Y)
# 显示LDA后的数据
plt.scatter(X[:, 0], X[:, 1], c=Y, cmap='rainbow', label='Original Data')
plt.scatter(X_lda[:, 0], np.zeros((3, 1)), color='red', label='LDA Data')
plt.legend()
plt.show()
# 输出LDA方向
print('LDA方向:', lda.coef_)
```
通过上述代码,我们对数据进行了LDA分析,并将数据投影到一维空间中。这种降维方式使得数据更加适合进行线性分类。通过可视化和输出LDA方向,我们可以看到LDA如何优化数据的可分性。
## 3.2 特征构造方法
### 3.2.1 基于聚合的方法
在机器学习中,特征构造是增加模型预测能力的一种重要方式。基于聚合的方法涉及将原始数据的多个特征合并成一个新的特征,以表示更复杂的数据关系或模式。常见的聚合方法包括取最大值、最小值、平均值、中位数等。
```python
import pandas as pd
# 创建一个简单的DataFrame作为示例数据
df = pd.DataFrame({
'feature1': [1, 3, 5],
'feature2': [2, 4, 6]
})
# 应用聚合函数
df['max_feature'] = df[['feature1', 'f
```
0
0
相关推荐
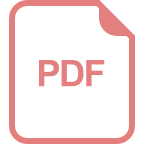
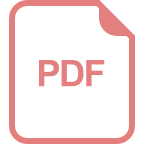
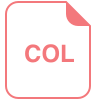
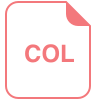
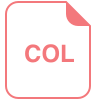
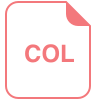
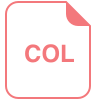
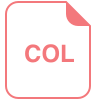