采用链表的方式来实现Josephus问题,并将创建链表,数小孩,小孩离开的步骤封装成函数,并用多文件结构管理程序
时间: 2024-03-07 15:49:29 浏览: 16
好的,我会尽力回答你的问题。
首先,我们需要定义一个链表结构体,以及创建链表、数小孩、小孩离开的函数。我们将这些函数放在一个单独的文件中,命名为josephus.h:
```c
// josephus.h
#ifndef JOSEPHUS_H
#define JOSEPHUS_H
// 定义链表结构体
typedef struct Node {
int num; // 小孩编号
struct Node *next; // 指向下一个小孩的指针
} Node;
// 创建链表,返回链表头指针
Node *create_list(int n);
// 数小孩,返回最后一个小孩的编号
int count_off(Node *head, int k);
// 小孩离开,返回下一个小孩的指针
Node *leave(Node *prev);
#endif
```
接下来,我们实现这些函数,并将它们放在一个单独的文件中,命名为josephus.c:
```c
// josephus.c
#include "josephus.h"
#include <stdio.h>
#include <stdlib.h>
Node *create_list(int n) {
Node *head = NULL, *tail = NULL;
for (int i = 1; i <= n; i++) {
Node *node = (Node*)malloc(sizeof(Node));
node->num = i;
node->next = NULL;
if (head == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
tail->next = head; // 将尾节点指向头节点,形成环形链表
return head;
}
int count_off(Node *head, int k) {
Node *prev = head;
while (prev->next != head) {
prev = prev->next;
}
Node *curr = head;
while (prev != curr) {
for (int i = 1; i < k; i++) {
prev = curr;
curr = curr->next;
}
prev->next = curr->next; // 将当前小孩从链表中删除
printf("%d ", curr->num);
free(curr);
curr = prev->next;
}
printf("\n");
return curr->num;
}
Node *leave(Node *prev) {
Node *curr = prev->next;
prev->next = curr->next; // 将当前小孩从链表中删除
printf("%d ", curr->num);
free(curr);
return prev->next;
}
```
最后,我们编写一个主函数来调用这些函数,将主函数也放在单独的文件中,命名为main.c:
```c
// main.c
#include "josephus.h"
#include <stdio.h>
int main() {
int n = 10;
int k = 3;
Node *head = create_list(n);
int last = count_off(head, k);
printf("The last one is %d\n", last);
return 0;
}
```
现在,我们已经将程序分成了多个文件,可以使用多文件结构来管理程序。我们需要将josephus.h和josephus.c编译成静态库或动态库,然后将其链接到主函数中。这里我们以静态库为例,编译命令如下:
```
gcc -c josephus.c
ar rcs libjosephus.a josephus.o
gcc main.c -L. -ljosephus -o main
```
其中,-c选项表示只编译不链接,-L选项指定静态库路径,-l选项指定静态库名称,-o选项指定输出文件名。运行main可执行文件即可得到结果。
希望我的回答对你有所帮助!
相关推荐
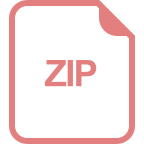














