c++文件中如何判断是否是中文句号
时间: 2024-02-15 09:01:53 浏览: 20
在 C++ 文件中,可以使用标准库中的字符编码库来判断一个字符是否是中文句号。具体实现如下:
```c++
#include <locale> // 头文件
#include <iostream>
int main() {
std::locale loc("zh_CN.UTF-8"); // 设置本地环境,使用 UTF-8 编码
wchar_t ch = L'。'; // 中文句号的 Unicode 编码是 0x3002
if (std::iswpunct(ch, loc)) { // 判断字符是否是标点符号
std::wcout << "中文句号" << std::endl;
}
return 0;
}
```
这里使用了 `std::locale` 类来设置本地环境,使用 UTF-8 编码。然后将字符转换成宽字符 `wchar_t`,使用 `std::iswpunct` 函数来判断字符是否是标点符号。如果是中文句号,就输出提示信息。
注意,在使用 `std::locale` 时需要确保本地环境中包含了相应的字符集和编码,否则可能无法正确识别中文句号。
相关问题
c++打开文件判断是否存在
在 C++ 中判断文件是否存在可以使用 `std::ifstream` 类,该类用于从文件中读取数据。你可以使用 `open()` 函数打开文件,如果文件不存在则无法打开。以下是示例代码:
```c++
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
if (!file.is_open()) {
std::cout << "File does not exist." << std::endl;
} else {
std::cout << "File exists." << std::endl;
}
return 0;
}
```
在上面的代码中,我们创建了一个 `std::ifstream` 对象并尝试打开名为 "example.txt" 的文件。如果文件不存在,则 `is_open()` 函数将返回 `false`,否则将返回 `true`。
C++,如何判断一篇中文文章中句号的个数
在 C++ 中,可以通过遍历字符串的方式来判断一篇中文文章中句号的个数。具体实现如下:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string article = "这是一篇中文文章。它包含多个句子。";
int count = 0;
for (int i = 0; i < article.length(); i++) {
if (article[i] == '。') {
count++;
}
}
cout << "句号的个数为:" << count << endl;
return 0;
}
```
上述代码中,首先定义了一个字符串变量 `article`,表示要统计句号个数的中文文章。然后使用一个计数器变量 `count`,初始化为 0。接着,通过 `for` 循环遍历字符串中的每一个字符,判断是否为句号,如果是则将计数器变量加 1。最后输出统计结果。
相关推荐
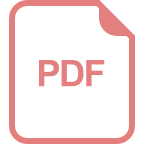
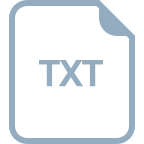











