vue实现前端登录注册
时间: 2023-08-30 22:05:24 浏览: 38
Vue可以通过axios库向后端发送HTTP请求来实现前端登录注册功能。下面是一个简单的示例:
1. 安装axios库
```bash
npm install axios --save
```
2. 创建登录注册组件
在组件中,需要定义data中的变量来存储用户输入的用户名和密码,并通过方法来发送HTTP请求。
```html
<template>
<div>
<h2>登录</h2>
<form>
<input type="text" v-model="username" placeholder="用户名">
<input type="password" v-model="password" placeholder="密码">
<button type="button" @click="login">登录</button>
</form>
<h2>注册</h2>
<form>
<input type="text" v-model="username" placeholder="用户名">
<input type="password" v-model="password" placeholder="密码">
<button type="button" @click="register">注册</button>
</form>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
username: '',
password: ''
}
},
methods: {
login() {
axios.post('/api/login', {
username: this.username,
password: this.password
}).then(response => {
console.log(response.data)
}).catch(error => {
console.log(error)
})
},
register() {
axios.post('/api/register', {
username: this.username,
password: this.password
}).then(response => {
console.log(response.data)
}).catch(error => {
console.log(error)
})
}
}
}
</script>
```
3. 在main.js中引入axios库
```js
import axios from 'axios'
Vue.prototype.$http = axios
```
4. 在后端实现登录注册接口
根据前端发送的请求,后端需要实现对应的登录和注册接口。
例如,使用Node.js和Express实现登录注册接口:
```js
const express = require('express')
const bodyParser = require('body-parser')
const app = express()
app.use(bodyParser.json())
app.post('/api/login', (req, res) => {
const { username, password } = req.body
// TODO: 验证用户名和密码
if (username === 'admin' && password === 'admin') {
res.json({ success: true })
} else {
res.json({ success: false, message: '用户名或密码错误' })
}
})
app.post('/api/register', (req, res) => {
const { username, password } = req.body
// TODO: 注册新用户
res.json({ success: true })
})
app.listen(3000, () => {
console.log('Server is running at http://localhost:3000')
})
```
以上是一个简单的Vue前端登录注册示例,仅供参考。实际项目中,需要根据具体需求进行修改和完善。
相关推荐
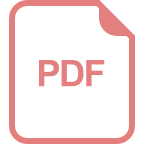
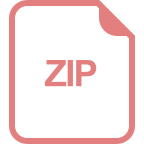














