通过Java设计一个酒店管理系统,并对MySQL数据库的连接读写
时间: 2024-02-28 19:57:15 浏览: 94
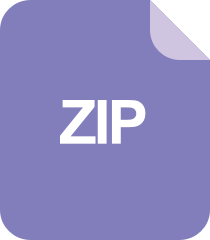
酒店管理系统(JAVA+MYSQL)

好的,我可以为您提供一些思路和代码示例,但是由于时间和篇幅的限制,我无法为您提供完整的代码实现。请您谅解。
首先,我们需要了解一下酒店管理系统的基本功能和要求,包括客房管理、员工管理、订单管理等。然后我们就可以开始设计系统架构和数据库结构,确定需要用到的表、字段和数据类型等。接着,我们可以使用Java进行编码实现,并利用MySQL数据库进行数据的读写操作。
以下是一个简单的Java代码示例,用于连接MySQL数据库并查询数据:
```java
import java.sql.*;
public class HotelManagementSystem {
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 加载MySQL驱动
Class.forName("com.mysql.jdbc.Driver");
// 连接MySQL数据库
String url = "jdbc:mysql://localhost:3306/hotel";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 执行SQL查询语句
stmt = conn.createStatement();
String sql = "SELECT * FROM room";
rs = stmt.executeQuery(sql);
// 输出查询结果
while (rs.next()) {
int id = rs.getInt("id");
String type = rs.getString("type");
int price = rs.getInt("price");
System.out.println("Room: " + id + " " + type + " " + price);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
上述代码中,我们首先加载MySQL驱动,然后使用`DriverManager.getConnection()`方法连接MySQL数据库。接着,我们使用`createStatement()`方法创建`Statement`对象,执行SQL查询语句,并通过`ResultSet`对象获取查询结果。最后,我们通过`close()`方法关闭数据库连接和相关对象。
当然,这只是一个简单的示例代码,实际的酒店管理系统需要更复杂的功能和更完善的代码实现。希望这些信息对您有所帮助。
阅读全文
相关推荐
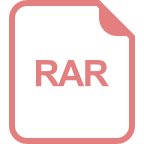
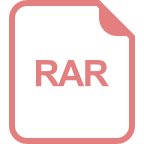
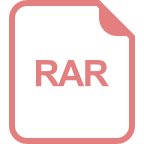
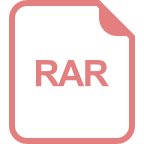
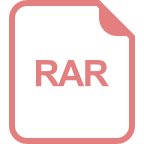
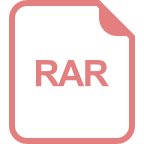
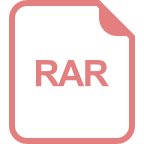
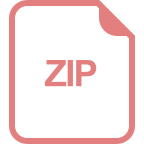
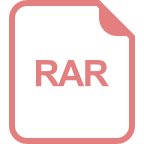
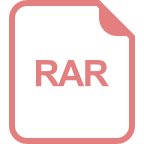
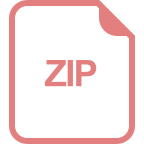
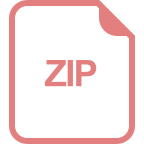
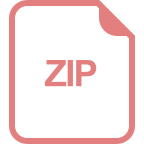
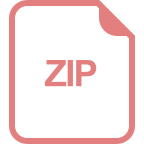
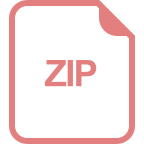
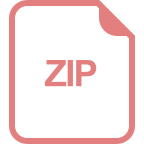
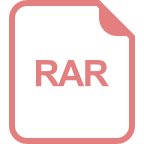