.h文件和.cpp文件的区别
时间: 2024-01-15 07:04:58 浏览: 270
.h文件和.cpp文件是两种常见的源代码文件扩展名。它们在C++编程中有不同的作用和用途。
.h文件是头文件(Header File)的缩写,用于声明函数、类、结构体、宏定义以及其他数据类型的定义。头文件通常包含在.cpp文件中,以便在程序中引用和使用。头文件中通常只包含函数、类和变量的声明,而不包含具体的实现代码。
.cpp文件是C++源文件的扩展名,包含实际的代码实现。它包含了函数、类和变量的定义和实现细节。.cpp文件中可以包含头文件,并将其中声明的函数、类和变量的实现代码写入其中。
因此,.h文件主要用于声明和定义,而.cpp文件主要用于实现和定义。头文件和源文件的分离有助于模块化编程和代码的重用性。通过在头文件中声明函数、类和变量,可以使其他.cpp文件在需要时引用并使用这些声明,避免了重复编写代码的问题。
相关问题
.hpp文件和.h文件,.cpp文件有什么区别
.h和.hpp文件都是C++头文件,用于声明类、函数、变量等元素的接口,供其他源文件调用。.h文件通常用于C++的标准库头文件,而.hpp文件通常用于开发者自己编写的类库头文件。
.h文件是C++的传统头文件格式,其内容通常包括类、函数等的声明,但不包括实现。.cpp文件是C++的源文件,包含函数和类的实现。
.hpp文件是C++11新增的头文件格式,与.h文件类似,但支持更多的C++11特性,如模板别名、constexpr函数等。.hpp文件通常包含类和函数的声明和实现。
总的来说,.h文件和.hpp文件都是用于声明C++接口的头文件,而.cpp文件则是用于实现C++代码的源文件。区别在于.h文件是传统的头文件格式,而.hpp文件是C++11新增的头文件格式,支持更多的C++11特性。
将上述程序分成.h文件和.cpp文件
以下是将获取所有窗口句柄的程序分成.h文件和.cpp文件的示例代码:
main.cpp:
```c++
#include <QApplication>
#include "mainwindow.h"
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
mainwindow.h:
```c++
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QWidget>
#include <QVector>
#include <Windows.h>
class QTextEdit;
class MainWindow : public QWidget
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
private:
static BOOL CALLBACK EnumWindowsProc(HWND hwnd, LPARAM lParam);
QTextEdit* textEdit;
};
#endif // MAINWINDOW_H
```
mainwindow.cpp:
```c++
#include "mainwindow.h"
#include <QVBoxLayout>
#include <QTextEdit>
MainWindow::MainWindow(QWidget *parent)
: QWidget(parent)
{
QVBoxLayout* layout = new QVBoxLayout(this);
textEdit = new QTextEdit(this);
layout->addWidget(textEdit);
setLayout(layout);
QVector<HWND> windows;
EnumWindows(EnumWindowsProc, reinterpret_cast<LPARAM>(&windows));
for (auto hwnd : windows)
{
QString title;
title.resize(GetWindowTextLength(hwnd));
GetWindowText(hwnd, title.data(), title.size());
textEdit->append(title);
}
}
BOOL CALLBACK MainWindow::EnumWindowsProc(HWND hwnd, LPARAM lParam)
{
QVector<HWND>* windows = reinterpret_cast<QVector<HWND>*>(lParam);
if (IsWindowVisible(hwnd))
{
windows->push_back(hwnd);
}
return TRUE;
}
```
在这个示例程序中,我们将MainWindow类的定义放在了mainwindow.h中,并将实现放在了mainwindow.cpp中。在mainwindow.cpp中,我们包含了mainwindow.h,并实现了MainWindow类的构造函数和EnumWindowsProc静态函数。我们在构造函数中创建了QTextEdit控件,并将窗口句柄的遍历和标题的获取放到了构造函数中。在mainwindow.h中,我们声明了MainWindow类,并将QTextEdit声明为一个前置声明。
阅读全文
相关推荐
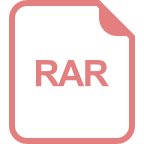
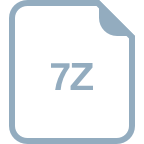
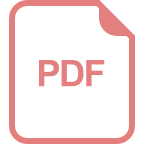













