linux内核线程池例子
时间: 2023-10-23 19:12:09 浏览: 199
好的,以下是一个简单的 Linux 内核线程池的例子:
```
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/init.h>
#include <linux/err.h>
#include <linux/slab.h>
#include <linux/kthread.h>
static struct task_struct *thread_pool[5];
static struct mutex thread_pool_mutex;
static int thread_func(void *unused)
{
int ret = 0;
printk(KERN_INFO "Thread [%d] started\n", current->pid);
while (!kthread_should_stop()) {
mutex_lock(&thread_pool_mutex);
/* Do some work */
mutex_unlock(&thread_pool_mutex);
}
printk(KERN_INFO "Thread [%d] stopping\n", current->pid);
return ret;
}
static int __init example_init(void)
{
int i, err = 0;
mutex_init(&thread_pool_mutex);
for (i = 0; i < 5 ; i++) {
thread_pool[i] = kthread_create(thread_func, NULL, "pool_thread");
if (IS_ERR(thread_pool[i])) {
err = PTR_ERR(thread_pool[i]);
thread_pool[i] = NULL;
goto exit;
}
wake_up_process(thread_pool[i]);
}
printk(KERN_INFO "Example init successful\n");
return 0;
exit:
printk(KERN_ERR "Failed to create thread_pool[%d]\n", i);
while (--i >= 0) {
if (thread_pool[i]) {
kthread_stop(thread_pool[i]);
}
}
return err;
}
static void __exit example_exit(void)
{
int i;
mutex_lock(&thread_pool_mutex);
for (i = 0; i < 5; i++) {
kthread_stop(thread_pool[i]);
}
mutex_unlock(&thread_pool_mutex);
printk(KERN_INFO "Example exit successful\n");
}
module_init(example_init);
module_exit(example_exit);
MODULE_LICENSE("GPL");
```
这个例子展示了如何在 Linux 内核中创建线程池。首先,我们创建一个互斥锁以保护线程池。然后,我们使用 `kthread_create` 函数创建 5 个内核线程,并通过 `wake_up_process` 函数启动它们。这些线程会不断循环执行,并在获取到互斥锁之后执行一些工作。最后,在卸载模块时,我们通过 `kthread_stop` 函数停止线程池的所有线程。
阅读全文
相关推荐
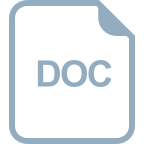
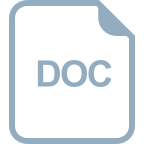
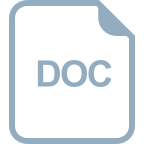


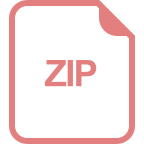
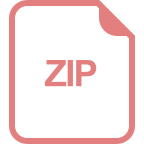
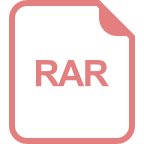
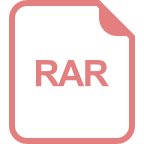
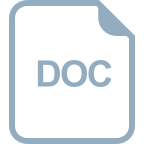
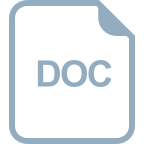
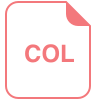
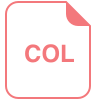
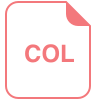
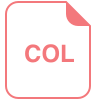
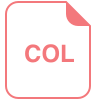
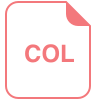
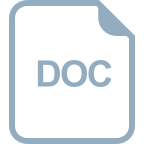
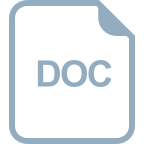