vue的拖拽移动一个元素让另一个也跟着走
时间: 2024-03-11 22:51:30 浏览: 16
你可以使用Vue的事件绑定和计算属性来实现拖拽移动一个元素让另一个也跟着走的效果。具体实现步骤如下:
1. 绑定mousedown事件,记录鼠标点击位置和拖拽元素的位置;
2. 绑定mousemove事件,计算鼠标移动距离,更新拖拽元素的位置;
3. 绑定mouseup事件,取消mousemove事件绑定;
4. 计算属性computed中计算第二个元素的位置,确保其跟随第一个元素的位置变化。
下面是一个简单的示例代码:
```html
<template>
<div class="container" @mousedown="handleMouseDown">
<div class="item1" :style="{ left: item1Left + 'px', top: item1Top + 'px' }"></div>
<div class="item2" :style="{ left: item2Left + 'px', top: item2Top + 'px' }"></div>
</div>
</template>
<script>
export default {
data() {
return {
startX: 0,
startY: 0,
item1Left: 0,
item1Top: 0,
item2Left: 0,
item2Top: 0
};
},
computed: {
item2Style() {
return {
left: this.item1Left + 'px',
top: this.item1Top + 'px'
};
}
},
methods: {
handleMouseDown(e) {
this.startX = e.clientX;
this.startY = e.clientY;
this.item1Left = e.target.offsetLeft;
this.item1Top = e.target.offsetTop;
document.addEventListener('mousemove', this.handleMouseMove);
document.addEventListener('mouseup', this.handleMouseUp);
},
handleMouseMove(e) {
const diffX = e.clientX - this.startX;
const diffY = e.clientY - this.startY;
this.item1Left += diffX;
this.item1Top += diffY;
this.startX = e.clientX;
this.startY = e.clientY;
},
handleMouseUp() {
document.removeEventListener('mousemove', this.handleMouseMove);
document.removeEventListener('mouseup', this.handleMouseUp);
}
}
};
</script>
<style>
.container {
position: relative;
width: 500px;
height: 500px;
}
.item1 {
position: absolute;
width: 100px;
height: 100px;
background-color: red;
}
.item2 {
position: absolute;
width: 50px;
height: 50px;
background-color: blue;
}
</style>
```
在这个示例中,我们使用了data属性来存储一些状态,使用computed属性来计算第二个元素的位置。在方法中,我们绑定了mousedown、mousemove、mouseup事件,通过计算鼠标移动距离来更新第一个元素的位置。在mouseup事件中,移除mousemove事件的绑定,结束拖拽。
相关推荐
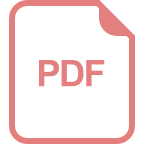
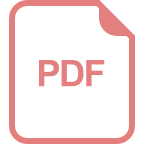
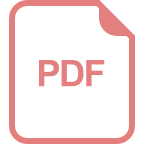














