c++判断一个字符串是否是回文
时间: 2023-06-21 10:16:35 浏览: 38
可以使用双指针的方法判断一个字符串是否是回文,具体实现如下:
```c++
#include <iostream>
#include <string>
using namespace std;
bool isPalindrome(string s) {
int left = 0, right = s.size() - 1;
while (left < right) {
if (s[left] != s[right]) {
return false;
}
left++;
right--;
}
return true;
}
int main() {
string s = "abcba";
if (isPalindrome(s)) {
cout << s << " is palindrome" << endl;
} else {
cout << s << " is not palindrome" << endl;
}
return 0;
}
```
输出结果为:
```
abcba is palindrome
```
另外,还可以使用函数库中的 `reverse()` 函数来判断一个字符串是否是回文,具体实现如下:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
bool isPalindrome(string s) {
string s_copy = s;
reverse(s_copy.begin(), s_copy.end());
return s == s_copy;
}
int main() {
string s = "abcba";
if (isPalindrome(s)) {
cout << s << " is palindrome" << endl;
} else {
cout << s << " is not palindrome" << endl;
}
return 0;
}
```
输出结果与前面的实现相同。
相关推荐
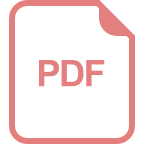














