springboot单点登录代码实现
时间: 2024-06-13 07:02:47 浏览: 304
Spring Boot 单点登录(Single Sign-On,简称 SSO)的实现通常涉及到以下几个关键步骤:
1. **集中认证服务器**:创建一个独立的服务,如使用 Spring Security 的 `CasServer` 或者 OAuth2 的 `/oauth/token` 接口,负责处理用户的登录验证。
```java
@Autowired
private AuthenticationManager authenticationManager;
@PostMapping("/login")
public ResponseEntity<?> authenticate(@RequestBody LoginRequest loginRequest) {
try {
Authentication authentication = authenticationManager.authenticate(loginRequest);
// 将认证信息存入到Token或Cookie
return new ResponseEntity<>(authentication, HttpStatus.OK);
} catch (AuthenticationException e) {
return new ResponseEntity<>(e.getMessage(), HttpStatus.UNAUTHORIZED);
}
}
```
2. **客户端应用配置**:每个依赖于单点登录的应用需要配置 Spring Security 或者其他身份验证框架,使其能够从统一的认证服务器获取令牌。这通常涉及配置 `SecurityFilterChain` 或 `WebSecurityConfigurerAdapter`。
3. **JWT 令牌管理**:可以使用 JWT(JSON Web Token)来存储和验证用户的登录状态。Spring Security 提供了集成,如 `@EnableWebSecurity` 和 `JwtAuthenticationEntryPoint`。
4. **过滤器或拦截器**:在客户端应用中设置一个过滤器或拦截器,用于在每个请求到达时检查 JWT 令牌的有效性。如果令牌有效,用户就无需再次登录。
```java
@Component
public class JwtFilter extends OncePerRequestFilter {
private final UserDetailsService userDetailsService;
private final JwtTokenProvider jwtTokenProvider;
// 构造函数注入依赖
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain)
throws ServletException, IOException {
// 验证JWT
String jwt = extractJwtFromHeader(request);
if (jwt != null && jwtTokenProvider.validate(jwt)) {
SecurityContextHolder.getContext().setAuthentication(authenticationFromJwt(jwt));
}
chain.doFilter(request, response);
}
}
```
5. **跨域支持**:如果你的应用部署在不同的域下,可能需要添加 CORS 头部以允许跨域访问。
阅读全文
相关推荐
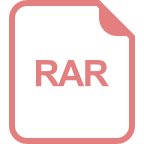
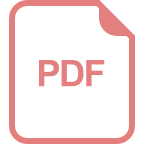
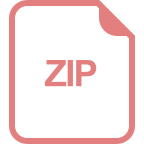
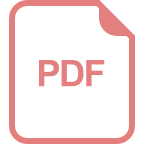
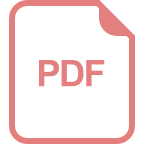
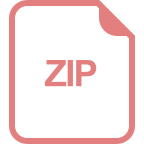
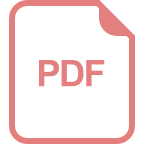
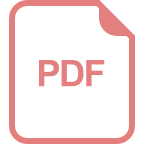
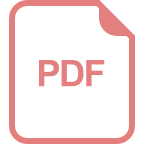
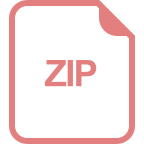
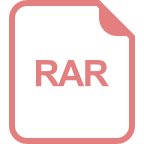
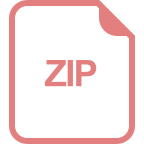
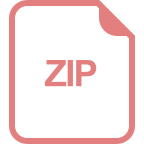
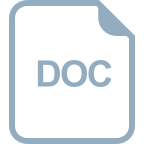
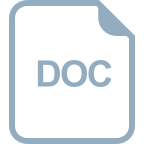

