设计友元函数friend ostream& operator << (ostream& Cout,CInteger& Integer);输出nInteger的值,是否有阶乘,若有阶乘则输出阶乘值以及nInteger是否素数
时间: 2023-03-15 08:24:25 浏览: 87
我可以用中文解释友元函数friend ostream:友元函数friend ostream是一种特殊类型的函数,它可以访问类的私有成员,从而实现特定的输出功能。友元函数可以在类中定义,也可以在类外定义。
相关问题
ostream & operator<<(ostream& cout,Person& p)
这是一个重载输出流运算符的函数,它的作用是将一个 Person 类型的对象输出到标准输出流中。具体实现方式可以参考以下代码:
```c++
#include<iostream>
#include<string>
using namespace std;
class Person{
public:
Person(string name,int age):m_name(name),m_age(age){}
friend ostream & operator<<(ostream& cout,Person& p);
private:
string m_name;
int m_age;
};
ostream & operator<<(ostream& cout,Person& p){
cout<<"Name: "<<p.m_name<<", Age: "<<p.m_age<<endl;
return cout;
}
int main(){
Person p("Tom", 18);
cout << p; // 输出 "Name: Tom, Age: 18"
return 0;
}
```
在上述代码中,重载输出流运算符的函数 `operator<<` 被定义为 `Person` 类的友元函数,它可以直接访问 `Person` 类的私有成员变量 `m_name` 和 `m_age`,并将它们输出到标准输出流中。在主函数中,我们创建一个 `Person` 对象 `p`,然后通过 `cout << p` 的方式将其输出到标准输出流中。
friend ostream&opterator<<<T> (ostream & out, LogUser & obj); 该句为友元声明无效如何解决
如果 `friend ostream& operator<< <T> (ostream & out, LogUser & obj);` 这个友元声明无效,可能是因为模板参数 `T` 没有被正确识别,可以尝试以下解决方法:
1. 将模板参数 `T` 显式指定为具体类型。例如,假设要输出 `LogUser` 对象的成员变量 `value`,可以这样实现:
```
template<typename T>
friend ostream& operator<<(ostream& out, const LogUser& obj)
{
out << obj.value;
return out;
}
```
在使用时,可以显式指定 `T` 的具体类型,比如:
```
LogUser<int> user(42);
cout << user << endl; // 显示 42
```
2. 将友元声明提到类的外部,并在声明时指定 `T` 的具体类型,例如:
```
template<typename T>
class LogUser {
public:
LogUser(T v): value(v) {}
template<typename U>
friend ostream& operator<<(ostream& out, const LogUser<U>& obj);
private:
T value;
};
template<typename U>
ostream& operator<<(ostream& out, const LogUser<U>& obj)
{
out << obj.value;
return out;
}
```
这样,就可以正常地输出 `LogUser` 对象的成员变量了。
相关推荐
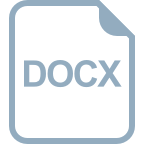
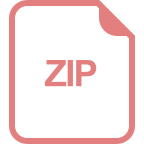
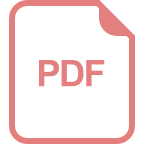













