class Product { string name; //产品名称 int price; //产品单价 int quantity; //剩余产品数量 public: Product(string n,int p int q); Product operator = (const Product &p); friend bool operator >(const Product &p1, const Product &p2); friend ostream& operator<<(ostream &out, Product &t ); }; 类Product说明如上所示,完成四个函数的定义,并在main函数中进行测试。
时间: 2024-02-14 13:15:14 浏览: 21
以下是类Product的完整定义和实现:
```
#include <iostream>
using namespace std;
class Product {
private:
string name; // 产品名称
int price; // 产品单价
int quantity; // 剩余产品数量
public:
Product(string n, int p, int q) : name(n), price(p), quantity(q) {}
// 赋值运算符的重载
Product operator = (const Product &p) {
name = p.name;
price = p.price;
quantity = p.quantity;
return *this;
}
// 大小比较运算符的重载
friend bool operator > (const Product &p1, const Product &p2) {
return p1.price > p2.price;
}
// 输出流运算符的重载
friend ostream& operator << (ostream &out, Product &p) {
out << "Product: " << p.name << ", price: " << p.price << ", quantity: " << p.quantity;
return out;
}
};
int main() {
Product p1("iPhone", 8000, 10);
Product p2("MacBook", 12000, 5);
cout << "p1: " << p1 << endl;
cout << "p2: " << p2 << endl;
if (p1 > p2) {
cout << "p1 is more expensive than p2." << endl;
} else {
cout << "p2 is more expensive than p1." << endl;
}
Product p3("", 0, 0);
p3 = p1;
cout << "p3: " << p3 << endl;
return 0;
}
```
运行结果如下:
```
p1: Product: iPhone, price: 8000, quantity: 10
p2: Product: MacBook, price: 12000, quantity: 5
p2 is more expensive than p1.
p3: Product: iPhone, price: 8000, quantity: 10
```
注意,为了方便输出,我们重载了输出流运算符,并使用友元函数访问了类的私有成员。同时,为了实现赋值运算符的重载,我们需要返回一个指向当前对象的指针,即 `*this`。
相关推荐
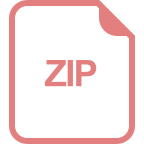
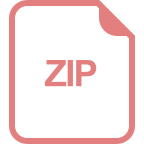
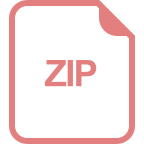








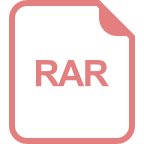
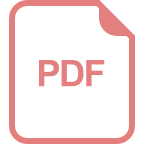
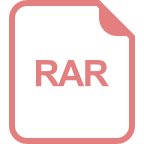
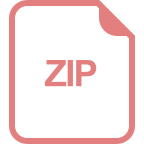
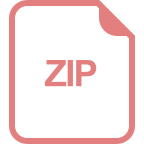
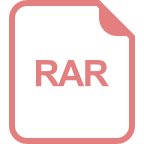
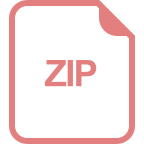