#include <iostream> #include <vector> #include <fstream> using namespace std; // 商品类 class Product { private: string name; double price; int quantity; public: Product(string name, double price, int quantity) { this->name = name; this->price = price; this->quantity = quantity; } string getName() { return name; } double getPrice() { return price; } int getQuantity() { return quantity; } }; // 零食销售管理系统类 class SnackSalesSystem { private: vector<Product> products; public: void addProduct(Product product) { products.push_back(product); } void displayProducts() { cout << "商品列表:" << endl; for (int i = 0; i < products.size(); i++) { cout << "名称:" << products[i].getName() << ",价格:" << products[i].getPrice() << ",库存数量:" << products[i].getQuantity() << endl; } } void saveToFile(string filename) { ofstream file(filename); if (file.is_open()) { for (int i = 0; i < products.size(); i++) { file << products[i].getName() << "," << products[i].getPrice() << "," << products[i].getQuantity() << endl; } file.close(); cout << "保存成功!" << endl; } else { cout << "保存失败!" << endl; } } void loadFromFile(string filename) { ifstream file(filename); if (file.is_open()) { products.clear(); string line; while (getline(file, line)) { string name, priceStr, quantityStr; double price; int quantity; size_t pos = line.find(","); name = line.substr(0, pos); line = line.substr(pos + 1); pos = line.find(","); priceStr = line.substr(0, pos); line = line.substr(pos + 1); quantityStr
时间: 2024-02-10 13:24:14 浏览: 64
这段代码是一个简单的零食销售管理系统,包含了商品类和零食销售管理系统类。在商品类中,包含了商品的名称、价格和库存数量信息。在零食销售管理系统类中,可以添加商品、显示商品列表、将商品信息保存到文件和从文件加载商品信息。
具体来说,代码中的`Product`类表示一个商品,包含了商品的名称、价格和库存数量。`SnackSalesSystem`类是一个零食销售管理系统,可以对商品进行添加、显示、保存和加载操作。
`addProduct`函数用于向系统中添加一个商品。`displayProducts`函数用于显示当前系统中的所有商品信息。`saveToFile`函数将商品信息保存到指定的文件中。`loadFromFile`函数从指定的文件中加载商品信息。
你有什么问题吗?
相关问题
#include <iostream> #include <fstream> #include <vector> #include <string> using namespace std; //
这是一段C++程序的头文件部分,包含了一些标准库的头文件,具体介绍如下:
1. iostream:提供了输入输出流的基本功能,如cin、cout等。
2. fstream:提供了文件操作相关的功能,如读写文件等。
3. vector:提供了可变大小数组的功能,可以方便地进行动态管理。
4. string:提供了字符串相关的功能,如字符串的拼接、截取等。
其中,using namespace std; 表示使用标准命名空间,可以简化程序中的代码。
优化这段代码#include <iostream> #include <fstream> #include <vector> using namespace std; class student { private: string name; const char* uni = "云南大学";//const常量 string department; string major; int age; long number; public: //static成员 static char* ident; //不带参数的构造函数 student() { number = 20201120425; name = "王文言"; department = "软件学院"; age = 21; major = "软件工程专业"; }
这段代码已经很简洁,但仍有一些可以优化的地方:
1. 类成员变量的初始化
可以使用成员初始化列表来初始化类成员变量,以提高效率。这样,成员变量的初始化将在对象构造时一次性完成,而不是先默认初始化,再赋值。
2. const常量的赋值方式
可以将const常量的值在定义时直接赋值,而无需在构造函数中进行赋值。
综上所述,优化后的代码如下:
```C++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
class student {
private:
string name;
const char* uni = "云南大学";
string department;
string major;
int age;
long number;
public:
//static成员
static char* ident;
//不带参数的构造函数
student() : name("王文言"), department("软件学院"), major("软件工程专业"), age(21), number(20201120425) {}
};
```
在上面的代码中,我们使用了成员初始化列表来初始化成员变量,将const常量的值在定义时直接赋值,而无需在构造函数中进行赋值。
阅读全文
相关推荐
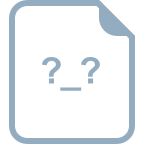
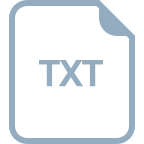
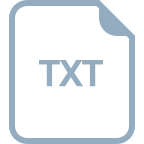
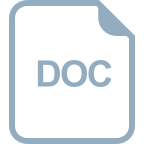
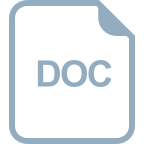
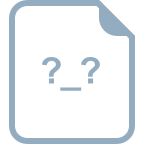
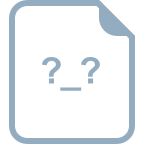
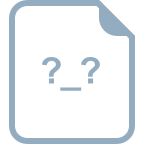
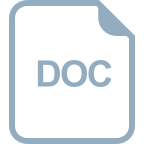
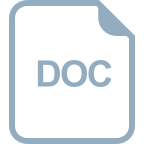
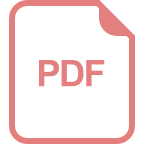
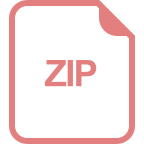
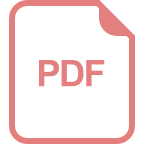
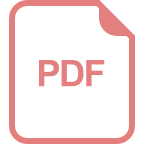
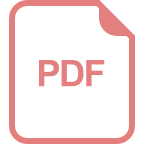