帮忙写一份多线程访问数据库获取大量数据的demo
时间: 2023-12-10 07:37:32 浏览: 43
好的,以下是一个简单的多线程访问数据库获取大量数据的demo,使用Python语言和MySQL数据库:
```python
import mysql.connector
from mysql.connector import Error
import threading
# 定义一个函数用于查询数据库
def query_database(offset):
try:
connection = mysql.connector.connect(host='localhost',
database='mydatabase',
user='myusername',
password='mypassword')
if connection.is_connected():
# 定义查询语句
query = "SELECT * FROM mytable LIMIT 100 OFFSET {}".format(offset)
cursor = connection.cursor()
cursor.execute(query)
# 获取查询结果
results = cursor.fetchall()
for row in results:
print(row)
cursor.close()
except Error as e:
print("Error while connecting to MySQL", e)
finally:
# 关闭数据库连接
if connection.is_connected():
connection.close()
# 定义一个函数用于创建多个线程
def create_threads():
threads = []
# 定义需要查询的数据总量
total_data = 1000
# 定义每个线程需要查询的数据量
data_per_thread = 100
# 计算需要创建的线程数量
num_threads = total_data // data_per_thread
# 创建多个线程并启动
for i in range(num_threads):
offset = i * data_per_thread
t = threading.Thread(target=query_database, args=(offset,))
threads.append(t)
t.start()
# 等待所有线程结束
for t in threads:
t.join()
# 调用函数创建多个线程并查询数据库
create_threads()
```
以上代码会创建多个线程,每个线程都会查询数据库的一部分数据。为了避免多个线程同时访问数据库造成冲突,我们使用Python内置的`threading`模块来创建线程,并在每个线程中打开一个新的数据库连接。由于每个线程都是独立的,因此它们可以同时运行,从而提高了程序的效率。
相关推荐
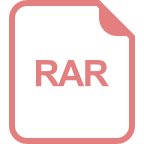














