angualr 发送数据请求
时间: 2023-11-02 07:07:00 浏览: 142
的方式有哪些?
Angular发送数据请求的常见方式包括:HTTP GET请求、HTTP POST请求、HTTP PUT请求和HTTP DELETE请求。具体的实现方式可以通过Angular的HttpClient模块进行实现。例如,可以使用Angular的HttpClient.get()、HttpClient.post()、HttpClient.put()和HttpClient.delete()方法进行相应请求的发送。同时,也可以通过HttpHeaders对象设置请求头信息,以及使用HttpParams对象附加查询参数。
相关问题
angular post 发送数据
在Angular中,可以使用HttpClient模块来发送POST请求并发送数据。下面是一个简单的示例:
首先,确保你已经导入了HttpClient模块,并在你的组件或服务中注入了HttpClient。
```typescript
import { HttpClient } from '@angular/common/http';
constructor(private http: HttpClient) { }
```
然后,你可以使用http.post()方法发送POST请求。在该方法中,你需要提供请求的URL和要发送的数据。
```typescript
const url = 'http://example.com/api/endpoint';
const data = { name: 'John', age: 30 };
this.http.post(url, data).subscribe(
response => {
console.log('Post request successful', response);
},
error => {
console.error('Error occurred', error);
}
);
```
在上面的示例中,我们定义了一个URL和一个包含name和age属性的数据对象。然后,我们使用http.post()方法发送POST请求,并使用subscribe()方法来处理响应和错误。
请注意,http.post()方法返回一个Observable对象,因此我们需要使用subscribe()方法来订阅它并处理响应或错误。
angular中post请求的body
在Angular中,POST请求通常被用于向服务器发送数据,比如提交表单数据或者创建一个新的资源。POST请求可以在请求体中包含数据。您可以使用HttpClient模块的post()方法来发送POST请求,并且需要提供请求体中的数据。例如:
```
import { HttpClient, HttpHeaders } from '@angular/common/http';
// 构造请求头
const httpOptions = {
headers: new HttpHeaders({
'Content-Type': 'application/json'
})
};
constructor(private http: HttpClient) {}
const data = { // 请求体中的数据
name: 'John Doe',
email: 'johndoe@example.com'
};
this.http.post('https://example.com/api/data', data, httpOptions).subscribe(response => {
console.log(response);
});
```
在上面的示例中,我们首先构造了一个包含请求头的httpOptions对象,指定请求体的数据格式为JSON。然后我们在post()方法中传递请求的URL、请求体中的数据和请求头。服务器可以从请求体中读取数据,并进行相应的处理。
阅读全文
相关推荐
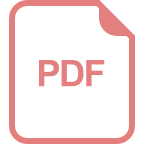
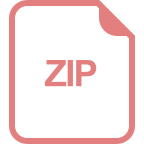
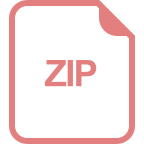


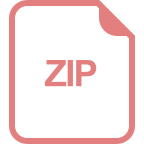
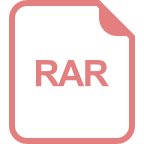
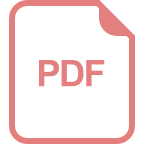
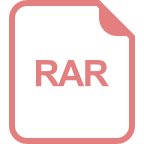
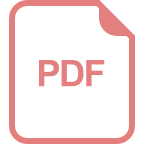
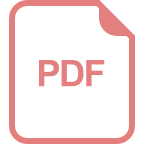
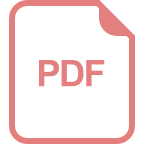
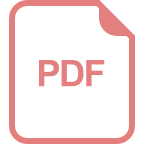
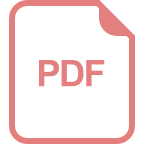
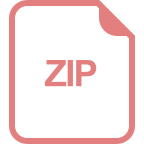