stm32f103c8t6 同时使用两个iic
时间: 2023-10-01 17:09:05 浏览: 332
在 STM32F103C8T6 上同时使用两个 I2C 总线,需要配置两个不同的 I2C 外设,并分别连接到不同的 I2C 设备上。以下是简单的步骤:
1. 配置 GPIO 端口作为 I2C 的 SDA 和 SCL 引脚。
2. 配置 I2C 外设的速度、时钟极性、时钟相位等参数。
3. 在程序中分别使用不同的 I2C 外设进行数据传输。
下面是一个简单的示例代码,可以同时使用两个 I2C 总线,分别连接到两个不同的设备上:
```c
#include "stm32f10x.h"
#include "stm32f10x_i2c.h"
#define I2C1_SCL_PIN GPIO_Pin_6
#define I2C1_SDA_PIN GPIO_Pin_7
#define I2C2_SCL_PIN GPIO_Pin_10
#define I2C2_SDA_PIN GPIO_Pin_11
void I2C1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
I2C_InitTypeDef I2C_InitStruct;
/* Enable GPIOB clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
/* Configure I2C1 pins */
GPIO_InitStruct.GPIO_Pin = I2C1_SCL_PIN | I2C1_SDA_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStruct);
/* Enable I2C1 clock */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
/* Configure I2C1 */
I2C_InitStruct.I2C_Mode = I2C_Mode_I2C;
I2C_InitStruct.I2C_DutyCycle = I2C_DutyCycle_2;
I2C_InitStruct.I2C_OwnAddress1 = 0x00;
I2C_InitStruct.I2C_Ack = I2C_Ack_Enable;
I2C_InitStruct.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit;
I2C_InitStruct.I2C_ClockSpeed = 100000;
I2C_Init(I2C1, &I2C_InitStruct);
/* Enable I2C1 */
I2C_Cmd(I2C1, ENABLE);
}
void I2C2_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
I2C_InitTypeDef I2C_InitStruct;
/* Enable GPIOB clock */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
/* Configure I2C2 pins */
GPIO_InitStruct.GPIO_Pin = I2C2_SCL_PIN | I2C2_SDA_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_OD;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStruct);
/* Enable I2C2 clock */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C2, ENABLE);
/* Configure I2C2 */
I2C_InitStruct.I2C_Mode = I2C_Mode_I2C;
I2C_InitStruct.I2C_DutyCycle = I2C_DutyCycle_2;
I2C_InitStruct.I2C_OwnAddress1 = 0x00;
I2C_InitStruct.I2C_Ack = I2C_Ack_Enable;
I2C_InitStruct.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit;
I2C_InitStruct.I2C_ClockSpeed = 100000;
I2C_Init(I2C2, &I2C_InitStruct);
/* Enable I2C2 */
I2C_Cmd(I2C2, ENABLE);
}
int main(void)
{
uint8_t data1, data2;
/* Initialize I2C1 and I2C2 */
I2C1_Init();
I2C2_Init();
/* Read data from device connected to I2C1 */
I2C_Read(I2C1, 0x50, &data1, 1);
/* Read data from device connected to I2C2 */
I2C_Read(I2C2, 0x60, &data2, 1);
while(1)
{
/* Do something */
}
}
```
其中,`I2C1_Init()` 和 `I2C2_Init()` 分别初始化了 I2C1 和 I2C2 两个外设。在 `main()` 函数中,首先调用 `I2C_Read()` 函数从连接到 I2C1 的设备读取数据,然后调用 `I2C_Read()` 函数从连接到 I2C2 的设备读取数据。
阅读全文
相关推荐
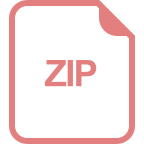
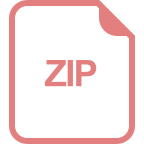
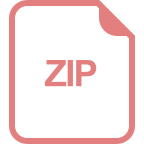















