在native层中,使用sp<SurfaceControl>SurfaceControl获取宽度和高度,以及对应的头文件
时间: 2024-03-11 20:48:48 浏览: 24
要在 native 层中使用 `sp<SurfaceControl>` 获取 SurfaceControl 的宽度和高度,可以使用 `SurfaceControl` 类的成员函数 `getWidth()` 和 `getHeight()`。同时,您需要包含以下头文件才能在 native 层中使用 SurfaceControl:
```c++
#include <gui/SurfaceControl.h>
#include <gui/Surface.h>
#include <android/native_window.h>
```
在您的代码中,您可以使用以下方式获取 SurfaceControl 的宽度和高度:
```c++
sp<SurfaceControl> surfaceControl;
int width, height;
// 获取 SurfaceControl 对象
surfaceControl = SurfaceControl::makeSurface(...);
// 获取 SurfaceControl 的宽度和高度
width = surfaceControl->getWidth();
height = surfaceControl->getHeight();
```
其中,`makeSurface(...)` 函数用于创建 SurfaceControl 对象,您需要根据您的具体需求填写参数。
相关问题
native层 ANativeWindow* nativeWindow 如何获取sp<SurfaceControl> control
在Native层,可以通过`SurfaceControl`对象获取`ANativeWindow`对象,而要获取`SurfaceControl`对象,则需要在Java层创建并传递给Native层。下面是示例代码:
在Java层创建`SurfaceControl`对象,并将其传递给Native层:
```java
// 创建SurfaceControl对象
SurfaceControl control = new SurfaceControl();
control.setBufferSize(width, height); // 设置宽高
control.setSecure(isSecure); // 设置是否安全
control.setFormat(format); // 设置像素格式
// 将SurfaceControl对象传递给Native层
nativeMethod(control);
```
在Native层,通过`ANativeWindow_fromSurfaceControl`函数获取`ANativeWindow`对象,并通过`ASurfaceControl_fromSurfaceControl`函数获取`SurfaceControl`对象:
```c++
#include <android/native_window.h>
#include <android/surface_control.h>
extern "C" JNIEXPORT void JNICALL
Java_com_example_NativeClass_nativeMethod(JNIEnv* env, jobject thiz, jobject surfaceControlObj) {
// 获取ANativeWindow对象
ANativeWindow* nativeWindow = ANativeWindow_fromSurfaceControl(env, surfaceControlObj);
// 获取SurfaceControl对象
ASurfaceControl* surfaceControl = ASurfaceControl_fromSurfaceControl(env, surfaceControlObj);
// 渲染代码...
}
```
在示例代码中,我们首先通过`ANativeWindow_fromSurfaceControl`函数获取`ANativeWindow`对象,然后通过`ASurfaceControl_fromSurfaceControl`函数获取`SurfaceControl`对象。最后,我们可以使用`ANativeWindow`对象进行渲染。
函数间智能指针传递sp<SurfaceControl>
智能指针是一种现代 C++ 中非常常用的内存管理工具,它可以自动管理对象的生命周期,避免内存泄漏等问题。而 Android 系统中的 sp<SurfaceControl> 则是一种特殊的智能指针,用于管理 SurfaceControl 对象的生命周期。
在函数间传递 sp<SurfaceControl> 指针时,可以使用引用传递的方式。例如:
```
void foo(sp<SurfaceControl>& surface) {
// do something with surface
}
int main() {
sp<SurfaceControl> surface = new SurfaceControl();
foo(surface);
return 0;
}
```
在上面的例子中,foo 函数通过引用传递的方式获取了 surface 智能指针,可以在函数内部对其进行操作,同时不会影响到原来的对象。
另外需要注意的是,使用 sp<SurfaceControl> 智能指针时,需要避免循环引用的问题,否则可能会导致内存泄漏。可以使用 wp<SurfaceControl> 弱指针来解决这个问题。
相关推荐
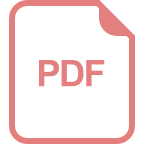
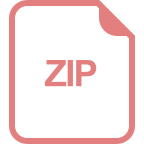












