python贪吃蛇最简单代码
时间: 2023-07-03 21:06:19 浏览: 76
下面是一个简单的 Python 贪吃蛇游戏的代码:
```python
import pygame, sys, time, random
from pygame.locals import *
# 初始化 Pygame
pygame.init()
# 设置游戏界面大小及标题
width = 640
height = 480
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption('Snake')
# 定义颜色
red = pygame.Color(255, 0, 0)
green = pygame.Color(0, 255, 0)
black = pygame.Color(0, 0, 0)
white = pygame.Color(255, 255, 255)
# 设置游戏帧率
fps = pygame.time.Clock()
# 定义蛇的初始位置和大小
snake_position = [100, 100]
snake_body = [[100, 100], [90, 100], [80, 100]]
# 定义食物的初始位置
food_position = [300, 300]
food_spawned = 1
# 定义方向
direction = 'RIGHT'
change_to = direction
# 定义分数
score = 0
# 定义游戏结束函数
def game_over():
font = pygame.font.SysFont('Arial', 30)
game_over_text = font.render('Game Over!', True, white)
game_over_rect = game_over_text.get_rect()
game_over_rect.midtop = (width / 2, height / 4)
screen.blit(game_over_text, game_over_rect)
pygame.display.flip()
time.sleep(2)
pygame.quit()
sys.exit()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == KEYDOWN:
if event.key == K_RIGHT or event.key == ord('d'):
change_to = 'RIGHT'
if event.key == K_LEFT or event.key == ord('a'):
change_to = 'LEFT'
if event.key == K_UP or event.key == ord('w'):
change_to = 'UP'
if event.key == K_DOWN or event.key == ord('s'):
change_to = 'DOWN'
if event.key == K_ESCAPE:
pygame.event.post(pygame.event.Event(QUIT))
# 判断方向是否相反
if change_to == 'RIGHT' and not direction == 'LEFT':
direction = 'RIGHT'
if change_to == 'LEFT' and not direction == 'RIGHT':
direction = 'LEFT'
if change_to == 'UP' and not direction == 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and not direction == 'UP':
direction = 'DOWN'
# 根据方向移动蛇头位置
if direction == 'RIGHT':
snake_position[0] += 10
if direction == 'LEFT':
snake_position[0] -= 10
if direction == 'UP':
snake_position[1] -= 10
if direction == 'DOWN':
snake_position[1] += 10
# 判断是否吃到食物
if snake_position[0] == food_position[0] and snake_position[1] == food_position[1]:
food_spawned = 0
score += 1
else:
# 移除蛇尾
snake_body.pop()
# 生成食物
if not food_spawned:
food_position = [random.randrange(1, (width // 10)) * 10,
random.randrange(1, (height // 10)) * 10]
food_spawned = 1
# 添加蛇头
snake_body.insert(0, list(snake_position))
# 绘制游戏界面
screen.fill(black)
for pos in snake_body:
pygame.draw.rect(screen, green, Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(screen, red, Rect(food_position[0], food_position[1], 10, 10))
# 判断游戏是否结束
if snake_position[0] < 0 or snake_position[0] > width - 10:
game_over()
if snake_position[1] < 0 or snake_position[1] > height - 10:
game_over()
for block in snake_body[1:]:
if snake_position[0] == block[0] and snake_position[1] == block[1]:
game_over()
# 显示分数
font = pygame.font.SysFont('Arial', 20)
score_text = font.render('Score: %s' % (score), True, white)
score_rect = score_text.get_rect()
score_rect.topleft = (10, 10)
screen.blit(score_text, score_rect)
# 更新游戏帧率
pygame.display.flip()
fps.tick(15)
```
这个代码可能有点长,但是实际上并不难理解。它使用 Pygame 库来实现游戏循环、事件处理、界面绘制等功能。在游戏循环中,不断地根据用户输入移动蛇头、判断是否吃到食物、生成食物、添加蛇身、判断游戏是否结束等等。最后,通过 Pygame 的界面绘制功能来实现游戏界面的绘制,以及分数等信息的显示。
阅读全文
相关推荐

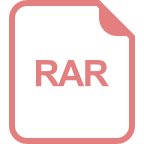
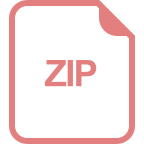
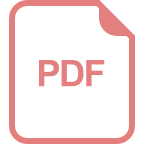
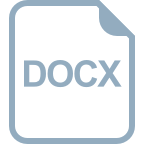
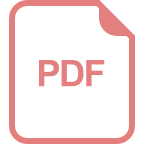







