type WebsiteNewsListResp struct { *decorator.ResponseCommon Data []WebsiteNewsList `json:"data"` }
时间: 2024-04-01 22:38:04 浏览: 20
这是一个 Golang 结构体定义,其中包含一个指向 `decorator.ResponseCommon` 结构体的指针和一个名为 `Data` 的切片,切片中存储了多个 `WebsiteNewsList` 结构体。这个结构体可能用于表示一个网站上的新闻列表响应。`json:"data"` 表示在将结构体转换为 JSON 格式时,`Data` 字段将被转换为名为 `data` 的 JSON 属性。
相关问题
func (c *cAsset) CreatComponent(r *ghttp.Request) { var req *v1.CreateComponentReq if err := r.Parse(&req); err != nil { r.Response.WriteJson(g.Map{ "code": 1, "msg": err.Error(), }) } createRequest := &creativecomponent.CreateRequest{ AdvertiserID: req.AdvertiserID, } res, err := service.Asset().Create(createRequest) if err != nil { r.Response.WriteJson(g.Map{ "code": 2, "msg": err.Error(), }) } r.Response.WriteJson(res) }这段代码中creativecomponent.CreateRequest的过滤条件为type CreateRequest struct { // AdvertiserID 广告主ID AdvertiserID uint64 `json:"advertiser_id,omitempty"` // ComponentInfo 组件信息 ComponentInfo *ComponentInfo `json:"component_info,omitempty"` },其中ComponentInfo为type ComponentInfo struct { // ComponentID 组件ID ComponentID model.Uint64 `json:"component_id,omitempty"` // ComponentType 组件类型 ComponentType enum.ComponentType `json:"component_type,omitempty"` // ComponentName 组件名称。长度小于等于20。一个中文长度为2 ComponentName string `json:"component_name,omitempty"` // ComponentData 组件详细信息。不同的component_type对应的值不同,具体的结构见创建或更新接口定义 ComponentData ComponentData `json:"component_data,omitempty"` // CreateTime 创建时间。格式"2020-12-25 15:12:08" CreateTime string `json:"create_time,omitempty"` // Status 组件审核状态。 Status enum.ComponentStatus `json:"status,omitempty"` }想要把ComponentInfo作为参数放到createRequest中,该怎么做?请详一点
要将ComponentInfo作为参数放入createRequest中,你可以按照以下步骤进行修改:
首先,你需要在CreateComponentReq结构体中添加一个ComponentInfo字段,用于接收组件信息,如下所示:
```go
type CreateComponentReq struct {
AdvertiserID uint64 `json:"advertiser_id" v:"required" dc:"广告主id"`
ComponentInfo *ComponentInfo `json:"component_info,omitempty"`
}
```
接下来,在CreateRequest结构体中将ComponentInfo字段的类型更改为*ComponentInfo,如下所示:
```go
type CreateRequest struct {
AdvertiserID uint64 `json:"advertiser_id,omitempty"`
ComponentInfo *ComponentInfo `json:"component_info,omitempty"`
}
```
然后,你可以根据实际需求创建一个ComponentInfo结构体,并将其作为参数传递给createRequest。以下是示例代码:
```go
func (c *cAsset) CreatComponent(r *ghttp.Request) {
var req *v1.CreateComponentReq
if err := r.Parse(&req); err != nil {
r.Response.WriteJson(g.Map{
"code": 1,
"msg": err.Error(),
})
}
// 创建ComponentInfo结构体
componentInfo := &creativecomponent.ComponentInfo{
ComponentID: model.Uint64(123), // 组件ID
ComponentType: enum.ComponentType("example"), // 组件类型
ComponentName: "Example Component", // 组件名称
// 具体的组件详细信息,根据实际情况进行填充
ComponentData: creativecomponent.ComponentData{
// ...
},
CreateTime: "2022-01-01 10:00:00", // 创建时间
Status: enum.ComponentStatus("pending"), // 组件审核状态
}
createRequest := &creativecomponent.CreateRequest{
AdvertiserID: req.AdvertiserID,
ComponentInfo: componentInfo, // 将ComponentInfo作为参数赋值给createRequest
}
res, err := service.Asset().Create(createRequest)
if err != nil {
r.Response.WriteJson(g.Map{
"code": 2,
"msg": err.Error(),
})
}
r.Response.WriteJson(res)
}
```
在上述示例中,我们创建了一个ComponentInfo结构体,并将其作为参数赋值给createRequest的ComponentInfo字段。你可以根据实际情况填充ComponentInfo结构体中的字段。请确保在填充ComponentData字段时,根据组件类型进行相应的赋值。
func (c *cAsset) GetComponentList(r *ghttp.Request) {var req *v1.GetComponentListReq if err := r.Parse(&req); err != nil {r.Response.WriteJson(g.Map{"code": 1,"msg": err.Error(),})} filtering := &creativecomponent.GetFiltering{ComponentID: req.ComponentId,ComponentName: req.ComponentName, ComponentTypes: []enum.ComponentType{},Status: []enum.ComponentStatus{},} getRequest := &creativecomponent.GetRequest{AdvertiserID: req.AdvertiserId,Page: req.Page,PageSize: req.PageSize,Filtering: filtering,} res, err := service.Asset().Get(getRequest) if err != nil {r.Response.WriteJson(g.Map{"code": 2,"msg": err.Error(),})} r.Response.WriteJson(res)} 这段代码中GetComponentListReq的过滤条件为type GetComponentListReq struct {g.Meta path:"/get_component_list" tags:"查询组件列表" method:"post" sm:"组件列表"AdvertiserId uint64 json:"advertiser_id" v:"required" dc:"广告主id" Page int json:"page" dc:"页码" PageSize int json:"page_size" dc:"每页数量。默认值20,最新值10,最大值40" ComponentId uint64 json:"component_id" dc:"组件id" ComponentName string json:"component_name" dc:"组件名称" ComponentTypes string json:"component_types" dc:"组件类型" Status string json:"status" dc:"组件审核状态" } creativecomponent.GetRequest的过滤条件为type GetRequest struct { AdvertiserID uint64 json:"advertiser_id,omitempty" Page int json:"page,omitempty" PageSize int json:"page_size,omitempty" Filtering *GetFiltering json:"filtering,omitempty" },GetFiltering的过滤条件为type GetFiltering struct { // ComponentID 组件ID ComponentID uint64 json:"component_id,omitempty" // ComponentName 组件名称。支持模糊查询 ComponentName string json:"component_name,omitempty" // ComponentTypes 组件类型,不传查全部。 ComponentTypes []enum.ComponentType json:"component_types,omitempty" // Status 组件审核状态,不传查全部。 Status []enum.ComponentStatus json:"status,omitempty" }。现在想要把ComponentTypes参数放到[]enum.ComponentType{}中,Status参数放到[]enum.ComponentStatus{}中,使代码能够运行,该怎么做?请详细一点
要将ComponentTypes参数放到[]enum.ComponentType{}中,Status参数放到[]enum.ComponentStatus{}中,你可以进行以下更改:
首先,你需要在GetComponentListReq结构体中将ComponentTypes和Status字段的类型更改为[]enum.ComponentType和[]enum.ComponentStatus,如下所示:
```go
type GetComponentListReq struct {
AdvertiserId uint64 `json:"advertiser_id" v:"required" dc:"广告主id"`
Page int `json:"page" dc:"页码"`
PageSize int `json:"page_size" dc:"每页数量。默认值20,最新值10,最大值40"`
ComponentId uint64 `json:"component_id" dc:"组件id"`
ComponentName string `json:"component_name" dc:"组件名称"`
ComponentTypes []enum.ComponentType `json:"component_types" dc:"组件类型"`
Status []enum.ComponentStatus `json:"status" dc:"组件审核状态"`
}
```
接下来,你需要在GetFiltering结构体中将ComponentTypes和Status字段的类型更改为[]enum.ComponentType和[]enum.ComponentStatus,如下所示:
```go
type GetFiltering struct {
ComponentID uint64 `json:"component_id,omitempty"`
ComponentName string `json:"component_name,omitempty"`
ComponentTypes []enum.ComponentType `json:"component_types,omitempty"`
Status []enum.ComponentStatus `json:"status,omitempty"`
}
```
这样的更改将使代码能够正确地将ComponentTypes参数放到[]enum.ComponentType{}中,Status参数放到[]enum.ComponentStatus{}中。
相关推荐
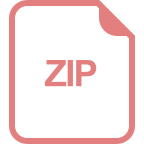
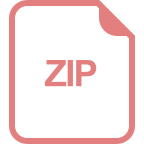
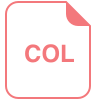
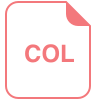
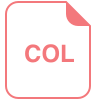
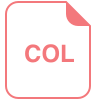









