python画三维散点图,显示散点数据,将鼠标移动到散点上时可以显示散点数据,怎么实现
时间: 2024-05-03 17:21:04 浏览: 86
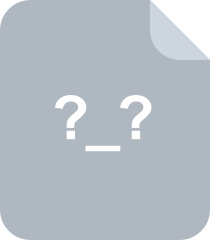
基于python的散点图绘制与实现
可以使用Python中的Matplotlib库和mpl_toolkits库来画三维散点图,并使用annot属性显示散点数据。具体实现方法请参考下面的代码:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 构造散点数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 1, 5, 3]
z = [3, 1, 4, 2, 5]
text = ['a', 'b', 'c', 'd', 'e']
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 画散点图
ax.scatter(x, y, z)
# 注释散点数据
for i, txt in enumerate(text):
ax.text(x[i], y[i], z[i], txt)
# 显示注释
annot = ax.annotate("", xy=(0,0), xytext=(20,20),textcoords="offset points",
bbox=dict(boxstyle="round", fc="w"),
arrowprops=dict(arrowstyle="->"))
annot.set_visible(False)
# 定义鼠标移动时的事件函数
def update_annot(ind):
pos = ax.transData.transform((x[ind], y[ind], z[ind]))
annot.xy = pos
text = f"x={x[ind]}, y={y[ind]}, z={z[ind]}"
annot.set_text(text)
# 鼠标移动时的事件
def hover(event):
vis = annot.get_visible()
if event.inaxes == ax:
cont, ind = ax.contains(event)
if cont:
update_annot(ind['ind'][0])
annot.set_visible(True)
fig.canvas.draw_idle()
else:
if vis:
annot.set_visible(False)
fig.canvas.draw_idle()
# 注册鼠标移动事件
fig.canvas.mpl_connect("motion_notify_event", hover)
# 显示图形
plt.show()
```
运行上述代码,就能够显示三维散点图并显示散点数据,当鼠标移动到某个散点上时,会显示该散点的数据。
阅读全文
相关推荐



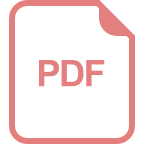











