#include<iostream> #include<string> using namespace std; class shape { public: double width; double length; shape(double=4.0, double=3.0):width(width),length(length){} }; class Rectangle :public shape { public: Rectangle(double width=4, double length=3) {} double get_Aera() { double area; area = width * length; cout << area; return width*length; } }; struct Student { string name; int age; double score; Student(string name="",int age=0,double score=0):name(name),age(age),score(score){} }; int main() { Rectangle r(4,3); cout << r.get_Aera() << endl; Student s("Tom", 20, 90); cout << s.name << s.age << s.score << endl; return 0; }使其正确
时间: 2023-11-22 21:54:39 浏览: 26
以下是经过修改后的代码:
```cpp
#include<iostream>
#include<string>
using namespace std;
class shape {
public:
double width;
double length;
shape(double w = 4.0, double l = 3.0) : width(w), length(l) {}
};
class Rectangle : public shape {
public:
Rectangle(double w = 4.0, double l = 3.0) : shape(w, l) {}
double get_Area() {
double area = width * length;
cout << area << endl;
return area;
}
};
struct Student {
string name;
int age;
double score;
Student(string n = "", int a = 0, double s = 0.0) : name(n), age(a), score(s) {}
};
int main() {
Rectangle r(4.0, 3.0);
cout << r.get_Area() << endl;
Student s("Tom", 20, 90.0);
cout << s.name << " " << s.age << " " << s.score << endl;
return 0;
}
```
主要修改如下:
1. 在 `shape` 类的构造函数中,变量名应该与参数名相同,而不是与成员变量名相同。
2. 在 `Rectangle` 类的构造函数中,应该调用父类 `shape` 的构造函数来初始化父类成员变量。
3. 在 `get_Area` 函数中,应该将计算结果赋值给 `area` 变量,然后再输出和返回。
4. 在 `main` 函数中,构造 `Rectangle` 和 `Student` 对象时应该传入参数值,而不是默认值。同时,输出 `Student` 对象时应该在每个属性值之间添加空格。
相关推荐
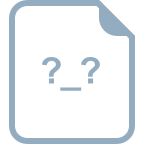











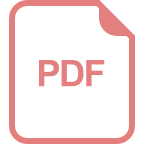
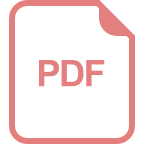
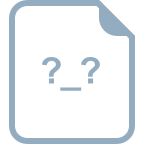
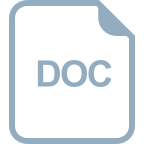