基于合力的平面点云边缘提取代码
时间: 2023-08-15 14:08:43 浏览: 107
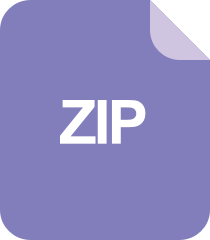
基于合力的点云边缘提取源代码(c++)

以下是基于合力的平面点云边缘提取的示例代码:
```
#include <iostream>
#include <pcl/point_types.h>
#include <pcl/features/normal_3d.h>
#include <pcl/filters/extract_indices.h>
#include <pcl/kdtree/kdtree_flann.h>
#include <pcl/features/integral_image_normal.h>
int main(int argc, char** argv)
{
// Load input point cloud
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud(new pcl::PointCloud<pcl::PointXYZ>);
pcl::io::loadPCDFile("input_cloud.pcd", *cloud);
// Compute normals for all points
pcl::NormalEstimation<pcl::PointXYZ, pcl::Normal> ne;
ne.setInputCloud(cloud);
pcl::search::KdTree<pcl::PointXYZ>::Ptr tree(new pcl::search::KdTree<pcl::PointXYZ>());
ne.setSearchMethod(tree);
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals(new pcl::PointCloud<pcl::Normal>);
ne.setRadiusSearch(0.03);
ne.compute(*cloud_normals);
// Compute integral image normal for all points
pcl::IntegralImageNormalEstimation<pcl::PointXYZ, pcl::Normal> ne_integral;
ne_integral.setNormalEstimationMethod(ne_integral.AVERAGE_3D_GRADIENT);
ne_integral.setMaxDepthChangeFactor(0.02f);
ne_integral.setNormalSmoothingSize(10.0f);
ne_integral.setInputCloud(cloud);
pcl::PointCloud<pcl::Normal>::Ptr cloud_normals_integral(new pcl::PointCloud<pcl::Normal>);
ne_integral.compute(*cloud_normals_integral);
// Compute curvature for all points
pcl::PointCloud<pcl::PointXYZINormal>::Ptr cloud_curvature(new pcl::PointCloud<pcl::PointXYZINormal>);
pcl::concatenateFields(*cloud, *cloud_normals_integral, *cloud_curvature);
pcl::search::KdTree<pcl::PointXYZINormal>::Ptr tree_curvature(new pcl::search::KdTree<pcl::PointXYZINormal>);
tree_curvature->setInputCloud(cloud_curvature);
pcl::CurvatureEstimation<pcl::PointXYZINormal, pcl::PointXYZINormal> ce;
ce.setInputCloud(cloud_curvature);
ce.setSearchMethod(tree_curvature);
ce.setRadiusSearch(0.03);
ce.compute(*cloud_curvature);
// Compute forces for all points
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_forces(new pcl::PointCloud<pcl::PointXYZ>);
pcl::PointXYZ force;
for (int i = 0; i < cloud->size(); ++i)
{
pcl::PointXYZ& p = cloud->points[i];
pcl::Normal& n = cloud_normals_integral->points[i];
pcl::PointXYZINormal& c = cloud_curvature->points[i];
force.x = n.normal_x * c.curvature;
force.y = n.normal_y * c.curvature;
force.z = n.normal_z * c.curvature;
cloud_forces->push_back(force);
}
// Extract edge points based on forces
pcl::ExtractIndices<pcl::PointXYZ> extractor;
pcl::IndicesPtr indices(new std::vector<int>);
for (int i = 0; i < cloud->size(); ++i)
{
pcl::PointXYZ& p = cloud->points[i];
pcl::PointXYZ& f = cloud_forces->points[i];
if (f.norm() > 0.1)
indices->push_back(i);
}
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_edges(new pcl::PointCloud<pcl::PointXYZ>);
extractor.setInputCloud(cloud);
extractor.setIndices(indices);
extractor.filter(*cloud_edges);
// Save edge points to file
pcl::io::savePCDFile("output_edges.pcd", *cloud_edges);
return 0;
}
```
这段代码中,我们首先加载了一个点云输入文件,然后计算了每个点的法向量和曲率。接着,我们通过计算每个点的合力来判断它是否为边缘点。最后,我们提取出所有的边缘点,并将它们保存到一个输出文件中。需要注意的是,这段代码中只处理了平面点云,如果要处理曲面点云,还需要进行额外的处理。
阅读全文
相关推荐
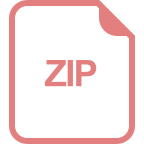















