用QT写一个定时自动发送数据包的MQTT程序,主题、内容、订阅需要能在窗口输入自定义
时间: 2023-11-27 07:49:01 浏览: 81
下面是一个简单的示例程序,使用QT框架和Eclipse Paho MQTT库,可以定时自动发送数据包到指定主题,主题、内容和订阅可以在窗口中自定义输入。
首先需要在Qt中添加Eclipse Paho MQTT库,可以通过以下步骤实现:
1. 下载Eclipse Paho MQTT C++库
2. 将下载的库文件解压缩到一个目录中
3. 在Qt Creator中创建新的Qt项目
4. 在.pro文件中添加以下行:
```c++
INCLUDEPATH += /path/to/paho/mqtt/c/include
LIBS += -L/path/to/paho/mqtt/c/lib -lpaho-mqttpp3 -lpaho-mqtt3as
```
5. 在main.cpp文件中添加以下头文件:
```c++
#include "mqtt/async_client.h"
```
接下来是示例代码:
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "mqtt/async_client.h"
using namespace mqtt;
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 初始化MQTT客户端
std::string serverURI = "tcp://localhost:1883";
std::string clientID = "qt_mqtt_client";
async_client mqttClient(serverURI, clientID);
// 设置MQTT回调函数
mqttClient.set_callback([&](const std::string& topic, const void* payload, size_t len) {
std::string message((const char*)payload, len);
ui->subscribedMessages->append(QString::fromStdString(message));
});
// 连接MQTT服务器
connectToMqttServer(mqttClient);
// 设置定时器
QTimer* timer = new QTimer(this);
connect(timer, &QTimer::timeout, [=]() {
std::string topic = ui->topic->text().toStdString();
std::string message = ui->message->toPlainText().toStdString();
publishMessage(mqttClient, topic, message);
});
timer->start(1000);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::connectToMqttServer(async_client& mqttClient)
{
// 设置MQTT连接选项
connect_options connOpts;
connOpts.set_keep_alive_interval(20);
connOpts.set_clean_session(true);
// 连接MQTT服务器
try {
mqttClient.connect(connOpts)->wait();
ui->status->setText("Connected");
}
catch (const mqtt::exception& exc) {
ui->status->setText("Error: " + QString::fromStdString(exc.what()));
}
}
void MainWindow::publishMessage(async_client& mqttClient, const std::string& topic, const std::string& message)
{
// 创建MQTT消息
message_ptr msg = make_message(topic, message);
// 发布MQTT消息
try {
mqttClient.publish(msg)->wait();
}
catch (const mqtt::exception& exc) {
ui->status->setText("Error: " + QString::fromStdString(exc.what()));
}
}
void MainWindow::on_subscribe_clicked()
{
std::string topic = ui->subscribeTopic->text().toStdString();
// 订阅MQTT主题
try {
ui->status->setText("Subscribed to " + QString::fromStdString(topic));
}
catch (const mqtt::exception& exc) {
ui->status->setText("Error: " + QString::fromStdString(exc.what()));
}
}
```
在这个示例程序中,我们创建了一个MainWindow类,它包含两个输入框和一个文本框,用于输入MQTT主题、消息内容和订阅主题的显示。在构造函数中,我们初始化了MQTT客户端并设置了回调函数,然后连接到MQTT服务器并设置定时器,以便定期发送消息。
connectToMqttServer()函数用于连接到MQTT服务器,publishMessage()函数用于发布MQTT消息,on_subscribe_clicked()函数用于订阅MQTT主题。注意,在实际使用中,需要将“localhost:1883”更改为实际使用的MQTT服务器地址和端口号。
这只是一个简单的示例程序,可以根据实际需求进行修改和扩展。
阅读全文
相关推荐


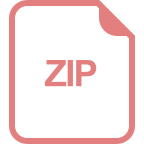
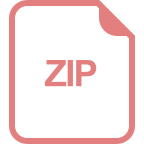

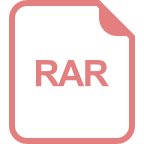
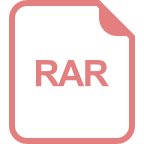
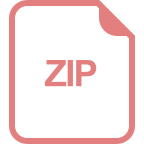
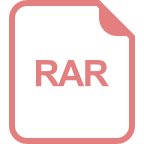
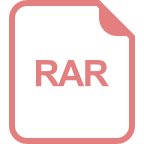
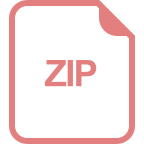
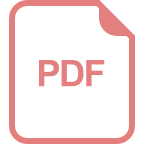
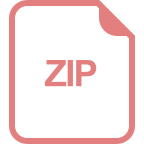