c++俄罗斯方块用easyx
时间: 2023-06-27 11:06:37 浏览: 164
好的,下面是一个简单的 C++ 俄罗斯方块游戏,使用 EasyX 图形库实现。在代码中,我使用了 EasyX 的绘图函数来绘制方块和游戏界面,使用了 EasyX 的键盘事件处理函数来响应玩家的操作。具体实现细节请看注释。
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
#define ROWS 20 // 游戏界面行数
#define COLS 10 // 游戏界面列数
#define BLOCK_SIZE 20 // 方块大小
int score = 0; // 分数
// 游戏界面
int map[ROWS][COLS] = {0};
// 方块类型
enum BlockType {
I_BLOCK, // I型方块
J_BLOCK, // J型方块
L_BLOCK, // L型方块
O_BLOCK, // O型方块
S_BLOCK, // S型方块
T_BLOCK, // T型方块
Z_BLOCK // Z型方块
};
// 方块结构体
struct Block {
int x, y; // 方块左上角的坐标
BlockType type; // 方块类型
int state; // 方块状态
};
// 方块数组,每种方块有4种状态
int block[7][4][5] = {
// I型方块
{
{1, 1, 1, 1, I_BLOCK},
{1, 1, 1, 1, I_BLOCK},
{1, 1, 1, 1, I_BLOCK},
{1, 1, 1, 1, I_BLOCK}
},
// J型方块
{
{1, 0, 0, 0, J_BLOCK},
{1, 1, 1, 0, J_BLOCK},
{0, 0, 0, 0, J_BLOCK},
{0, 0, 0, 0, J_BLOCK}
},
// L型方块
{
{0, 0, 1, 0, L_BLOCK},
{1, 1, 1, 0, L_BLOCK},
{0, 0, 0, 0, L_BLOCK},
{0, 0, 0, 0, L_BLOCK}
},
// O型方块
{
{1, 1, 0, 0, O_BLOCK},
{1, 1, 0, 0, O_BLOCK},
{0, 0, 0, 0, O_BLOCK},
{0, 0, 0, 0, O_BLOCK}
},
// S型方块
{
{0, 1, 1, 0, S_BLOCK},
{1, 1, 0, 0, S_BLOCK},
{0, 0, 0, 0, S_BLOCK},
{0, 0, 0, 0, S_BLOCK}
},
// T型方块
{
{0, 1, 0, 0, T_BLOCK},
{1, 1, 1, 0, T_BLOCK},
{0, 0, 0, 0, T_BLOCK},
{0, 0, 0, 0, T_BLOCK}
},
// Z型方块
{
{1, 1, 0, 0, Z_BLOCK},
{0, 1, 1, 0, Z_BLOCK},
{0, 0, 0, 0, Z_BLOCK},
{0, 0, 0, 0, Z_BLOCK}
}
};
// 绘制单个方块
void drawBlock(int x, int y, COLORREF color) {
setfillcolor(color);
setlinecolor(RGB(0, 0, 0));
rectangle(x, y, x + BLOCK_SIZE, y + BLOCK_SIZE);
floodfill(x + 1, y + 1, RGB(0, 0, 0));
}
// 绘制游戏界面
void drawMap() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (map[i][j] != 0) {
drawBlock(j * BLOCK_SIZE, i * BLOCK_SIZE, RGB(255, 255, 255));
}
}
}
}
// 绘制方块
void drawBlock(Block b) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[b.type][b.state][i * 4 + j] == 1) {
drawBlock((b.x + j) * BLOCK_SIZE, (b.y + i) * BLOCK_SIZE, RGB(255, 255, 255));
}
}
}
}
// 检查方块是否能够放置在指定位置
bool check(Block b) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[b.type][b.state][i * 4 + j] == 1) {
int x = b.x + j;
int y = b.y + i;
if (x < 0 || x >= COLS || y < 0 || y >= ROWS || map[y][x] != 0) {
return false;
}
}
}
}
return true;
}
// 添加方块到游戏界面
void add(Block b) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[b.type][b.state][i * 4 + j] == 1) {
int x = b.x + j;
int y = b.y + i;
map[y][x] = b.type + 1;
}
}
}
}
// 移除方块从游戏界面
void remove(Block b) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (block[b.type][b.state][i * 4 + j] == 1) {
int x = b.x + j;
int y = b.y + i;
map[y][x] = 0;
}
}
}
}
// 随机生成一个方块
Block generateBlock() {
Block b;
b.x = 3;
b.y = 0;
b.type = rand() % 7;
b.state = 0;
return b;
}
// 方块下落
void down(Block &b) {
remove(b);
b.y++;
if (check(b)) {
add(b);
} else {
b.y--;
add(b);
// 判断游戏是否结束
if (b.y <= 0) {
settextstyle(40, 0, "黑体");
outtextxy(200, 200, "Game Over!");
getch();
exit(0);
}
// 消行
int lines = 0;
for (int i = ROWS - 1; i >= 0; i--) {
bool flag = true;
for (int j = 0; j < COLS; j++) {
if (map[i][j] == 0) {
flag = false;
break;
}
}
if (flag) {
for (int k = i; k >= 1; k--) {
for (int j = 0; j < COLS; j++) {
map[k][j] = map[k - 1][j];
}
}
lines++;
i++;
}
}
// 计算分数
score += lines * 100;
}
}
int main() {
// 初始化窗口
initgraph(COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE);
setbkcolor(RGB(0, 0, 0));
cleardevice();
// 随机生成一个方块
srand(time(0));
Block b = generateBlock();
// 游戏主循环
while (true) {
// 绘制游戏界面
drawMap();
// 绘制方块
drawBlock(b);
// 显示分数
settextcolor(RGB(255, 255, 255));
settextstyle(20, 0, "黑体");
char buf[16];
sprintf(buf, "Score: %d", score);
outtextxy(10, 10, buf);
// 方块下落
down(b);
// 处理键盘事件
if (kbhit()) {
char ch = getch();
switch (ch) {
case 'a': // 左移
remove(b);
b.x--;
if (check(b)) {
add(b);
} else {
b.x++;
add(b);
}
break;
case 'd': // 右移
remove(b);
b.x++;
if (check(b)) {
add(b);
} else {
b.x--;
add(b);
}
break;
case 's': // 加速下落
down(b);
break;
case 'w': // 旋转
remove(b);
b.state = (b.state + 1) % 4;
if (check(b)) {
add(b);
} else {
b.state = (b.state + 3) % 4;
add(b);
}
break;
case 'q': // 退出游戏
exit(0);
break;
}
}
Sleep(100);
}
return 0;
}
```
希望对你有所帮助!
阅读全文
相关推荐
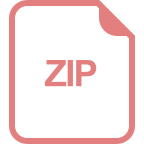

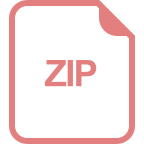
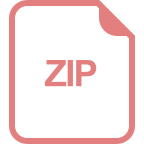
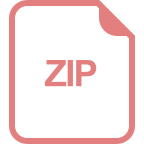
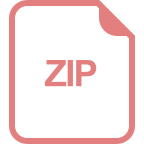
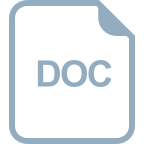
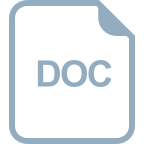






