QT读取音频文件并绘制图像
时间: 2024-05-11 15:14:05 浏览: 16
下面是一个基本的例子,可以读取音频文件并绘制出其波形图:
```python
import matplotlib.pyplot as plt
import numpy as np
import wave
# 打开音频文件
file = wave.open('audio.wav', 'r')
# 获取音频数据
signal = file.readframes(-1)
signal = np.fromstring(signal, 'Int16')
# 获取音频采样率
fs = file.getframerate()
# 获取音频时间轴
time = np.linspace(0, len(signal)/fs, num=len(signal))
# 绘制波形图
plt.figure(figsize=(10,5))
plt.plot(time, signal)
plt.xlabel('Time (s)')
plt.ylabel('Amplitude')
plt.show()
```
在这个例子中,我们使用了Python的wave模块来打开音频文件并读取其数据。我们使用numpy库将读取的音频数据转换为numpy数组,以便更容易地处理和绘制。然后,我们使用matplotlib库来绘制波形图。我们还使用了音频数据的采样率来创建时间轴,并将其用作x轴。
相关问题
QT使用C读取音频文件并绘制图像
首先,需要使用一个音频库来读取音频文件,常用的有libsndfile和portaudio等。这里以libsndfile为例,需要在代码中添加库文件的链接。
接下来,需要使用FFT(快速傅里叶变换)算法对音频数据进行频谱分析,得到音频的频域数据。常用的FFT库有FFTW和KISSFFT等,这里以KISSFFT为例。同样需要在代码中添加库文件的链接。
最后,使用绘图库(如Qt自带的QPainter)将频域数据绘制成图像。具体代码如下:
```c++
#include <QtCore/QCoreApplication>
#include <QtGui/QPainter>
#include <QDebug>
#include <kiss_fft.h>
#include <sndfile.h>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 打开音频文件
SF_INFO sfinfo;
SNDFILE* file = sf_open("test.wav", SFM_READ, &sfinfo);
if (!file) {
qDebug() << "Failed to open audio file!";
return -1;
}
// 读取音频数据
const int N = 1024;
float buf[N];
kiss_fft_cpx fft_in[N], fft_out[N];
kiss_fft_cfg cfg = kiss_fft_alloc(N, false, 0, 0);
QPainter painter;
QImage image(N, N, QImage::Format_RGB32);
painter.begin(&image);
int i, j, n;
while ((n = sf_read_float(file, buf, N)) > 0) {
// 对音频数据进行FFT变换
for (i = 0; i < N; i++) {
fft_in[i].r = buf[i];
fft_in[i].i = 0;
}
kiss_fft(cfg, fft_in, fft_out);
// 绘制频谱图
painter.fillRect(0, 0, N, N, Qt::black);
for (i = 0; i < N / 2; i++) {
float mag = sqrt(fft_out[i].r * fft_out[i].r + fft_out[i].i * fft_out[i].i);
mag = log(mag + 1);
mag = mag * 255 / 10;
QColor color(mag, mag, mag);
painter.setPen(color);
painter.drawLine(i, N, i, N - mag);
}
painter.end();
image.save(QString("test_%1.png").arg(j++));
}
// 释放资源
kiss_fft_free(cfg);
sf_close(file);
return a.exec();
}
```
这段代码使用了1024个采样点进行FFT变换,绘制了一个1024x1024的频谱图,并将每一帧保存为一张PNG图片。可以通过调整N的大小来改变频谱图的分辨率。同时,也可以在绘图过程中加入更多的细节,如绘制坐标轴、标注等。
qt绘制频IFFt图像
要绘制频率域的IFFT图像,首先需要进行以下步骤:
1. 从音频文件中读取数据并进行FFT变换,得到频域数据。
2. 对频域数据进行IFFT变换,得到时域数据。
3. 将时域数据绘制成图像。
下面是一个使用Qt库绘制频率域IFFT图像的代码示例:
```cpp
#include <QMainWindow>
#include <QGraphicsView>
#include <QGraphicsScene>
#include <QGraphicsPixmapItem>
#include <QImage>
#include <fftw3.h>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr)
: QMainWindow(parent)
{
// 读取音频文件
// 这里使用的是libsndfile库,需要自行安装
SNDFILE *infile;
SF_INFO sfinfo;
infile = sf_open("audio.wav", SFM_READ, &sfinfo);
// 分配内存并读取数据
int N = sfinfo.frames;
fftw_complex *in = (fftw_complex*) fftw_malloc(sizeof(fftw_complex) * N);
fftw_complex *out = (fftw_complex*) fftw_malloc(sizeof(fftw_complex) * N);
sf_readf_complex(infile, in, N);
// 进行IFFT变换
fftw_plan plan = fftw_plan_dft_1d(N, in, out, FFTW_BACKWARD, FFTW_ESTIMATE);
fftw_execute(plan);
// 创建图像
QImage image(N, 512, QImage::Format_RGB32);
image.fill(Qt::white);
// 绘制图像
QPainter painter(&image);
for (int i = 0; i < N; i++)
{
int y = (int)(512.0 - out[i][0] * 256.0);
painter.drawLine(i, 0, i, y);
}
// 显示图像
QGraphicsPixmapItem *item = new QGraphicsPixmapItem(QPixmap::fromImage(image));
QGraphicsScene *scene = new QGraphicsScene;
scene->addItem(item);
QGraphicsView *view = new QGraphicsView(scene);
setCentralWidget(view);
}
};
```
这个示例使用了fftw库进行FFT和IFFT变换,需要安装该库才能编译通过。代码中还使用了Qt库的一些绘图函数来绘制图像,最终结果会显示在一个QGraphicsView中。
相关推荐
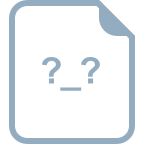






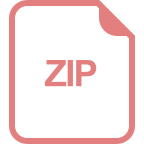
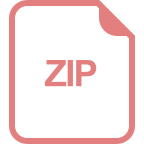
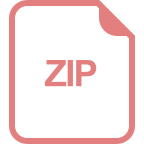
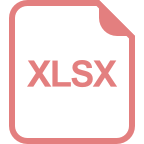
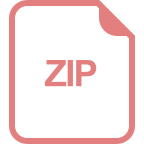