for n in range(num_exhaustives): temp_database_path = f"{feature_dir}/colmap_{n}.db" shutil.copyfile(database_path, temp_database_path) pycolmap.match_exhaustive(temp_database_path) db = COLMAPDatabase.connect(temp_database_path) cursor = db.execute("SELECT * from two_view_geometries") matches = np.array(cursor.fetchall()) for row, m in enumerate(matches): if row not in all_matches: all_matches[row] = {} data = np.frombuffer(m[3], dtype=np.uint32).reshape(-1,2) if m[3] else None all_matches[row][n] = data db.close()代码中num_exhaustives是什么作用,怎么修改能提高匹配精度
时间: 2024-04-01 12:35:57 浏览: 129
在这段代码中,`num_exhaustives` 是指进行多少次匹配。此处的代码通过复制原始数据库 `database_path`,然后对每个副本进行匹配,最终将所有匹配结果存储在 `all_matches` 中。这里的目的是通过多次匹配来提高匹配的精度。
如果想提高匹配的精度,可以考虑增加 `num_exhaustives` 的值,即增加匹配的次数。但是,需要注意的是,过多的匹配可能会导致运行时间变长,同时也可能会导致匹配结果的质量变差。因此,需要在匹配次数和匹配质量之间做出平衡。
相关问题
详细解释一下这段代码,每一句都要进行注解:for dataset in datasets: print(dataset) if dataset not in out_results: out_results[dataset] = {} for scene in data_dict[dataset]: print(scene) # Fail gently if the notebook has not been submitted and the test data is not populated. # You may want to run this on the training data in that case? img_dir = f'{src}/test/{dataset}/{scene}/images' if not os.path.exists(img_dir): continue # Wrap the meaty part in a try-except block. try: out_results[dataset][scene] = {} img_fnames = [f'{src}/test/{x}' for x in data_dict[dataset][scene]] print (f"Got {len(img_fnames)} images") feature_dir = f'featureout/{dataset}{scene}' if not os.path.isdir(feature_dir): os.makedirs(feature_dir, exist_ok=True) t=time() index_pairs = get_image_pairs_shortlist(img_fnames, sim_th = 0.5644583, # should be strict min_pairs = 33, # we select at least min_pairs PER IMAGE with biggest similarity exhaustive_if_less = 20, device=device) t=time() -t timings['shortlisting'].append(t) print (f'{len(index_pairs)}, pairs to match, {t:.4f} sec') gc.collect() t=time() if LOCAL_FEATURE != 'LoFTR': detect_features(img_fnames, 2048, feature_dir=feature_dir, upright=True, device=device, resize_small_edge_to=600 ) gc.collect() t=time() -t timings['feature_detection'].append(t) print(f'Features detected in {t:.4f} sec') t=time() match_features(img_fnames, index_pairs, feature_dir=feature_dir,device=device) else: match_loftr(img_fnames, index_pairs, feature_dir=feature_dir, device=device, resize_to=(600, 800)) t=time() -t timings['feature_matching'].append(t) print(f'Features matched in {t:.4f} sec') database_path = f'{feature_dir}/colmap.db' if os.path.isfile(database_path): os.remove(database_path) gc.collect() import_into_colmap(img_dir, feature_dir=feature_dir,database_path=database_path) output_path = f'{feature_dir}/colmap_rec_{LOCAL_FEATURE}' t=time() pycolmap.match_exhaustive(database_path) t=time() - t timings['RANSAC'].append(t) print(f'RANSAC in {t:.4f} sec')
这段代码是Python代码,用于图像匹配和三维重建。以下是每行代码的注释:
```python
for dataset in datasets: # 遍历数据集列表
print(dataset) # 输出当前数据集
if dataset not in out_results: # 如果当前数据集不在输出结果中
out_results[dataset] = {} # 添加一个空字典作为该数据集的输出结果
for scene in data_dict[dataset]: # 遍历当前数据集中的场景
print(scene) # 输出当前场景
# 如果在测试集中,但该场景的图像数据未被提供,则跳过
img_dir = f'{src}/test/{dataset}/{scene}/images'
if not os.path.exists(img_dir):
continue
try: # 尝试匹配图像并执行三维重建
out_results[dataset][scene] = {} # 添加一个空字典作为该场景的输出结果
img_fnames = [f'{src}/test/{x}' for x in data_dict[dataset][scene]] # 获取当前场景中的图像文件名列表
print(f"Got {len(img_fnames)} images")
feature_dir = f'featureout/{dataset}{scene}' # 设置特征输出目录
if not os.path.isdir(feature_dir): # 如果特征输出目录不存在,则创建该目录
os.makedirs(feature_dir, exist_ok=True)
t = time()
# 获取图像对的候选列表
index_pairs = get_image_pairs_shortlist(img_fnames, sim_th=0.5644583, min_pairs=33,
exhaustive_if_less=20, device=device)
t = time() - t
timings['shortlisting'].append(t)
print(f'{len(index_pairs)}, pairs to match, {t:.4f} sec')
gc.collect() # 执行垃圾回收以释放内存
t = time()
# 如果不使用LoFTR,则检测图像中的特征点
if LOCAL_FEATURE != 'LoFTR':
detect_features(img_fnames, 2048, feature_dir=feature_dir, upright=True,
device=device, resize_small_edge_to=600)
gc.collect()
t = time() - t
timings['feature_detection'].append(t)
print(f'Features detected in {t:.4f} sec')
# 匹配图像中的特征点
match_features(img_fnames, index_pairs, feature_dir=feature_dir, device=device)
# 如果使用LoFTR,则使用LoFTR进行特征匹配
else:
match_loftr(img_fnames, index_pairs, feature_dir=feature_dir, device=device,
resize_to=(600, 800))
t = time() - t
timings['feature_matching'].append(t)
print(f'Features matched in {t:.4f} sec')
database_path = f'{feature_dir}/colmap.db'
if os.path.isfile(database_path):
os.remove(database_path)
gc.collect()
# 将特征匹配结果导入到COLMAP数据库中
import_into_colmap(img_dir, feature_dir=feature_dir, database_path=database_path)
output_path = f'{feature_dir}/colmap_rec_{LOCAL_FEATURE}'
t = time()
# 使用COLMAP执行RANSAC算法进行三维重建
pycolmap.match_exhaustive(database_path)
t = time() - t
timings['RANSAC'].append(t)
print(f'RANSAC in {t:.4f} sec')
except Exception as e: # 捕获任何异常
print(f'Scene {scene} failed. Error: {e}')
```
此代码的主要目的是使用图像匹配和三维重建技术来重建场景。在此过程中,它使用了许多库和函数,例如os、time、gc、pycolmap等。
详细解释一下这段代码,每一句给出详细注解:results_df = pd.DataFrame(columns=['image_path', 'dataset', 'scene', 'rotation_matrix', 'translation_vector']) for dataset_scene in tqdm(datasets_scenes, desc='Running pipeline'): dataset, scene = dataset_scene.split('/') img_dir = f"{INPUT_ROOT}/{'train' if DEBUG else 'test'}/{dataset}/{scene}/images" if not os.path.exists(img_dir): continue feature_dir = f"{DATA_ROOT}/featureout/{dataset}/{scene}" os.system(f"rm -rf {feature_dir}") os.makedirs(feature_dir) fnames = sorted(glob(f"{img_dir}/*")) print('fnames',len(fnames)) # Similarity pipeline if sim_th: index_pairs, h_w_exif = get_image_pairs_filtered(similarity_model, fnames=fnames, sim_th=sim_th, min_pairs=20, all_if_less=20) else: index_pairs, h_w_exif = get_img_pairs_all(fnames=fnames) # Matching pipeline matching_pipeline(matching_model=matching_model, fnames=fnames, index_pairs=index_pairs, feature_dir=feature_dir) # Colmap pipeline maps = colmap_pipeline(img_dir, feature_dir, h_w_exif=h_w_exif) # Postprocessing results = postprocessing(maps, dataset, scene) # Create submission for fname in fnames: image_id = '/'.join(fname.split('/')[-4:]) if image_id in results: R = results[image_id]['R'].reshape(-1) T = results[image_id]['t'].reshape(-1) else: R = np.eye(3).reshape(-1) T = np.zeros((3)) new_row = pd.DataFrame({'image_path': image_id, 'dataset': dataset, 'scene': scene, 'rotation_matrix': arr_to_str(R), 'translation_vector': arr_to_str(T)}, index=[0]) results_df = pd.concat([results_df, new_row]).reset_index(drop=True)
这段代码运行了一个三维重建的 pipeline,以下是每一行的详细注释:
```python
results_df = pd.DataFrame(columns=['image_path', 'dataset', 'scene', 'rotation_matrix', 'translation_vector'])
```
创建一个空的 pandas DataFrame,用于存储结果。
```python
for dataset_scene in tqdm(datasets_scenes, desc='Running pipeline'):
dataset, scene = dataset_scene.split('/')
img_dir = f"{INPUT_ROOT}/{'train' if DEBUG else 'test'}/{dataset}/{scene}/images"
if not os.path.exists(img_dir):
continue
feature_dir = f"{DATA_ROOT}/featureout/{dataset}/{scene}"
os.system(f"rm -rf {feature_dir}")
os.makedirs(feature_dir)
```
遍历数据集和场景的组合,为每个场景的图像创建一个特征目录。如果图片目录不存在则跳过。
```python
fnames = sorted(glob(f"{img_dir}/*"))
print('fnames',len(fnames))
# Similarity pipeline
if sim_th:
index_pairs, h_w_exif = get_image_pairs_filtered(similarity_model, fnames=fnames, sim_th=sim_th, min_pairs=20, all_if_less=20)
else:
index_pairs, h_w_exif = get_img_pairs_all(fnames=fnames)
# Matching pipeline
matching_pipeline(matching_model=matching_model,
fnames=fnames,
index_pairs=index_pairs,
feature_dir=feature_dir)
```
获取图像文件名列表,按名称排序,并运行相似性和匹配管道。如果存在相似性阈值,则使用 `get_image_pairs_filtered` 函数过滤匹配对,否则使用 `get_img_pairs_all` 函数获取所有匹配对。然后,使用 `matching_pipeline` 函数进行特征匹配。
```python
maps = colmap_pipeline(img_dir, feature_dir, h_w_exif=h_w_exif)
```
运行 Colmap 管道,使用 Colmap 进行三维重建。
```python
results = postprocessing(maps, dataset, scene)
```
对 Colmap 重建结果进行后处理,以获得更准确的结果。
```python
for fname in fnames:
image_id = '/'.join(fname.split('/')[-4:])
if image_id in results:
R = results[image_id]['R'].reshape(-1)
T = results[image_id]['t'].reshape(-1)
else:
R = np.eye(3).reshape(-1)
T = np.zeros((3))
new_row = pd.DataFrame({'image_path': image_id,
'dataset': dataset,
'scene': scene,
'rotation_matrix': arr_to_str(R),
'translation_vector': arr_to_str(T)}, index=[0])
results_df = pd.concat([results_df, new_row]).reset_index(drop=True)
```
对每个图像,将其重建结果加入到结果 DataFrame 中。首先,使用 `image_id` 变量生成图像的唯一标识符。然后,从 `results` 字典中获取该图像的旋转矩阵和平移向量。如果没有结果,则将旋转矩阵设置为单位矩阵,将平移向量设置为零向量。最后,将新结果添加到结果 DataFrame 中。
阅读全文
相关推荐
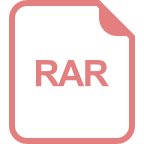
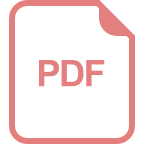
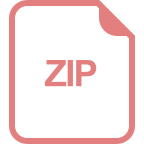


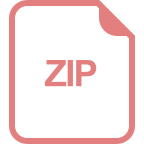
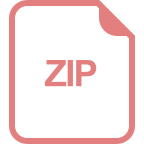
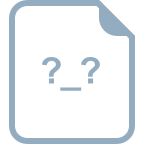
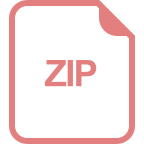





