帮我写个gtest的示例程序,要求在现有工程中新开一个线程运行gtest,测试项目为在5个线程调用函数1000次测试总耗时
时间: 2024-05-08 19:16:19 浏览: 7
以下是一个简单的gtest示例程序,用于测试在5个线程中调用函数1000次的总耗时。
```
#include <gtest/gtest.h>
#include <thread>
#include <chrono>
// The function to be tested
int myFunction() {
int sum = 0;
for (int i = 0; i < 1000; i++) {
sum += i;
}
return sum;
}
// The test fixture
class MyTest : public ::testing::Test {
protected:
void SetUp() override {
// Initialize any resources needed for the tests
}
void TearDown() override {
// Clean up any resources used by the tests
}
};
// The test case
TEST_F(MyTest, TestMyFunction) {
// Start the timer
auto start = std::chrono::high_resolution_clock::now();
// Create 5 threads and call myFunction 1000 times in each thread
std::vector<std::thread> threads;
for (int i = 0; i < 5; i++) {
threads.emplace_back([](){
for (int j = 0; j < 1000; j++) {
myFunction();
}
});
}
// Wait for all threads to finish
for (auto& thread : threads) {
thread.join();
}
// Stop the timer and calculate the elapsed time
auto end = std::chrono::high_resolution_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
// Print the elapsed time
std::cout << "Elapsed time: " << elapsed.count() << " ms" << std::endl;
// Assert that the elapsed time is less than 5000 ms
ASSERT_LE(elapsed.count(), 5000);
}
// Entry point for the program
int main(int argc, char** argv) {
// Initialize the gtest framework
::testing::InitGoogleTest(&argc, argv);
// Run the tests in a new thread
std::thread t([](){
RUN_ALL_TESTS();
});
// Wait for the test thread to finish
t.join();
return 0;
}
```
在这个示例程序中,我们定义了一个名为`myFunction`的函数,该函数将从0加到999并返回总和。我们的测试目标是在5个线程中调用这个函数1000次,并测试总共需要多长时间。
我们使用了gtest的测试框架来编写测试。我们创建了一个名为`MyTest`的测试夹具,并在其中定义了一个名为`TestMyFunction`的测试用例。在测试用例中,我们使用了C++11的`std::thread`来创建5个线程,并在每个线程中调用`myFunction`函数1000次。我们使用了C++11的`std::chrono`库来计算总共需要多长时间。最后,我们使用了gtest的`ASSERT_LE`宏来断言总共需要的时间不超过5000毫秒。
在`main`函数中,我们初始化了gtest框架,并在一个新线程中运行测试。我们使用了C++11的`std::thread`来创建一个新线程,并在其中调用`RUN_ALL_TESTS`函数来运行所有的gtest测试。最后,我们等待测试线程完成并返回0。
相关推荐
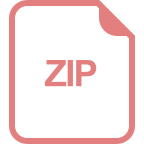
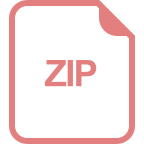
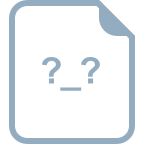














