carID列为csv文件中出租车车牌号,去除表头后每一行表示一个订单,现需用python语言统计出租车完成的订单量数量分布,删除订单量大于200的数据并绘图,横坐标为车辆接收的订单数量,纵坐标为对应订单数量的出租车数量
时间: 2024-05-14 12:16:24 浏览: 107
首先需要使用Python读取CSV文件,并将车辆完成订单数量统计出来。假设CSV文件名为"orders.csv",代码如下:
```python
import csv
order_counts = {}
with open("orders.csv", newline='') as csvfile:
reader = csv.reader(csvfile)
next(reader) # skip header row
for row in reader:
car_id = row[0]
if car_id in order_counts:
order_counts[car_id] += 1
else:
order_counts[car_id] = 1
```
上述代码将CSV文件中的订单数据逐行读取,并统计每辆出租车完成的订单数量,结果存储在一个字典中,字典的键为车牌号,值为订单数量。
接下来,我们需要将订单数量进行分组,并计算每组的出租车数量。代码如下:
```python
from collections import defaultdict
car_counts = defaultdict(int)
for car_id, count in order_counts.items():
car_counts[count] += 1
```
上述代码使用了Python标准库中的`defaultdict`,它是一个可以自动初始化值的字典,这里我们将它初始化为0。然后遍历每辆出租车完成的订单数量,将它们按照数量分组,并统计每组的出租车数量。
最后,我们需要删除订单量大于200的数据,并绘制图表。代码如下:
```python
import matplotlib.pyplot as plt
filtered_car_counts = {k: v for k, v in car_counts.items() if k <= 200}
x = list(filtered_car_counts.keys())
y = list(filtered_car_counts.values())
plt.bar(x, y)
plt.xlabel("Order Count")
plt.ylabel("Number of Cars")
plt.show()
```
上述代码首先使用字典推导式将订单量大于200的数据过滤掉,然后将剩余的数据绘制成柱状图。图表的横坐标为订单数量,纵坐标为对应订单数量的出租车数量。
完整代码如下:
```python
import csv
from collections import defaultdict
import matplotlib.pyplot as plt
order_counts = {}
with open("orders.csv", newline='') as csvfile:
reader = csv.reader(csvfile)
next(reader) # skip header row
for row in reader:
car_id = row[0]
if car_id in order_counts:
order_counts[car_id] += 1
else:
order_counts[car_id] = 1
car_counts = defaultdict(int)
for car_id, count in order_counts.items():
car_counts[count] += 1
filtered_car_counts = {k: v for k, v in car_counts.items() if k <= 200}
x = list(filtered_car_counts.keys())
y = list(filtered_car_counts.values())
plt.bar(x, y)
plt.xlabel("Order Count")
plt.ylabel("Number of Cars")
plt.show()
```
阅读全文
相关推荐
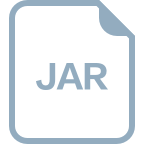

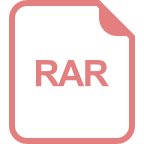












