用Java输入5个学生的信息(包含学号、姓名、3科成绩),统计各学生的总分,然后将学生信息和统计结果存入二进制数据文件STUDENT.DAT中。从建立的STUDENT.DAT文件中读取数据,寻找平均分最高的学生,并输出该学生的所有信息。
时间: 2024-03-09 22:50:38 浏览: 156
以下是用Java实现该功能的代码:
```java
import java.io.*;
public class StudentDemo {
public static void main(String[] args) {
Student[] students = new Student[5];
DataOutputStream dos = null;
DataInputStream dis = null;
try {
dos = new DataOutputStream(new FileOutputStream("STUDENT.DAT"));
dis = new DataInputStream(new FileInputStream("STUDENT.DAT"));
for (int i = 0; i < 5; i++) {
System.out.println("请输入第" + (i + 1) + "个学生的信息:");
System.out.print("学号:");
int id = Integer.parseInt(System.console().readLine());
System.out.print("姓名:");
String name = System.console().readLine();
System.out.print("成绩1:");
double score1 = Double.parseDouble(System.console().readLine());
System.out.print("成绩2:");
double score2 = Double.parseDouble(System.console().readLine());
System.out.print("成绩3:");
double score3 = Double.parseDouble(System.console().readLine());
Student student = new Student(id, name, score1, score2, score3);
students[i] = student;
dos.writeInt(student.getId());
dos.writeUTF(student.getName());
dos.writeDouble(student.getScore1());
dos.writeDouble(student.getScore2());
dos.writeDouble(student.getScore3());
}
double maxAvg = 0;
int maxIndex = 0;
for (int i = 0; i < 5; i++) {
double totalScore = students[i].getScore1() + students[i].getScore2() + students[i].getScore3();
double avgScore = totalScore / 3;
students[i].setTotalScore(totalScore);
students[i].setAvgScore(avgScore);
if (avgScore > maxAvg) {
maxAvg = avgScore;
maxIndex = i;
}
}
System.out.println("学生信息和统计结果已存入STUDENT.DAT文件中。");
System.out.println("平均分最高的学生是:");
System.out.println("学号:" + students[maxIndex].getId());
System.out.println("姓名:" + students[maxIndex].getName());
System.out.println("成绩1:" + students[maxIndex].getScore1());
System.out.println("成绩2:" + students[maxIndex].getScore2());
System.out.println("成绩3:" + students[maxIndex].getScore3());
System.out.println("总分:" + students[maxIndex].getTotalScore());
System.out.println("平均分:" + students[maxIndex].getAvgScore());
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (dos != null) {
dos.close();
}
if (dis != null) {
dis.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
class Student {
private int id;
private String name;
private double score1;
private double score2;
private double score3;
private double totalScore;
private double avgScore;
public Student(int id, String name, double score1, double score2, double score3) {
this.id = id;
this.name = name;
this.score1 = score1;
this.score2 = score2;
this.score3 = score3;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getScore1() {
return score1;
}
public double getScore2() {
return score2;
}
public double getScore3() {
return score3;
}
public double getTotalScore() {
return totalScore;
}
public void setTotalScore(double totalScore) {
this.totalScore = totalScore;
}
public double getAvgScore() {
return avgScore;
}
public void setAvgScore(double avgScore) {
this.avgScore = avgScore;
}
}
```
请将代码保存为StudentDemo.java文件,并在命令行中输入以下命令来编译和运行程序:
```
javac StudentDemo.java
java StudentDemo
```
注意:在实现过程中,需要注意异常处理和资源释放,以保证程序的稳定性和可靠性。
阅读全文
相关推荐















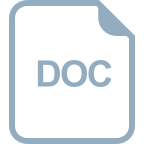


