用javabean实现购物车
时间: 2024-05-28 09:09:09 浏览: 64
Sure, using JavaBean to implement a shopping cart is a common practice in web development. Essentially, you can create a JavaBean class to represent a shopping cart item, and then use an ArrayList or LinkedList to store all the items in the cart. You can then manipulate this list (e.g., adding or removing items) and display the updated cart to the user. Do you need more information on this topic?
相关问题
应用javabean实现购物车
好的,这是一个关于Java编程的问题。可以使用JavaBean来实现购物车功能,JavaBean是一种符合特定规范的Java类,可以用于存储和操作数据。在购物车中,可以创建一个JavaBean类来表示购物车中的商品,包括商品名称、价格、数量等信息。通过使用JavaBean,可以方便地添加、删除和修改购物车中的商品,实现购物车功能。
javabean实现购物车功能
购物车功能一般需要以下几个步骤:
1. 将商品加入购物车;
2. 修改购物车中商品的数量;
3. 删除购物车中的商品;
4. 清空购物车;
5. 计算购物车中商品的总价。
下面是一个简单的购物车实现:
1. 定义一个商品类 Item,包含商品的编号、名称、价格和数量等属性。
```
public class Item {
private int id; // 商品编号
private String name; // 商品名称
private double price; // 商品价格
private int quantity; // 商品数量
// 构造方法
public Item(int id, String name, double price, int quantity) {
this.id = id;
this.name = name;
this.price = price;
this.quantity = quantity;
}
// getter 和 setter 方法
// ...
}
```
2. 定义一个购物车类 ShoppingCart,包含购物车中所有商品的列表。
```
public class ShoppingCart {
private List<Item> itemList; // 商品列表
public ShoppingCart() {
itemList = new ArrayList<Item>();
}
// 加入商品到购物车
public void addItem(Item item) {
itemList.add(item);
}
// 修改购物车中商品的数量
public void updateItemQuantity(int itemId, int quantity) {
for (Item item : itemList) {
if (item.getId() == itemId) {
item.setQuantity(quantity);
break;
}
}
}
// 从购物车中删除商品
public void removeItem(int itemId) {
Iterator<Item> iterator = itemList.iterator();
while (iterator.hasNext()) {
Item item = iterator.next();
if (item.getId() == itemId) {
iterator.remove();
break;
}
}
}
// 清空购物车
public void clear() {
itemList.clear();
}
// 计算购物车中商品的总价
public double getTotalPrice() {
double totalPrice = 0.0;
for (Item item : itemList) {
totalPrice += item.getPrice() * item.getQuantity();
}
return totalPrice;
}
// getter 和 setter 方法
// ...
}
```
3. 在页面中使用 ShoppingCart 类实现购物车功能。
```
<%-- 加入商品到购物车 --%>
<%
int itemId = Integer.parseInt(request.getParameter("itemId"));
String itemName = request.getParameter("itemName");
double itemPrice = Double.parseDouble(request.getParameter("itemPrice"));
int itemQuantity = Integer.parseInt(request.getParameter("itemQuantity"));
Item item = new Item(itemId, itemName, itemPrice, itemQuantity);
HttpSession session = request.getSession(true);
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute("cart", cart);
}
cart.addItem(item);
%>
<%-- 修改购物车中商品的数量 --%>
<%
int itemId = Integer.parseInt(request.getParameter("itemId"));
int itemQuantity = Integer.parseInt(request.getParameter("itemQuantity"));
HttpSession session = request.getSession(true);
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart != null) {
cart.updateItemQuantity(itemId, itemQuantity);
}
%>
<%-- 从购物车中删除商品 --%>
<%
int itemId = Integer.parseInt(request.getParameter("itemId"));
HttpSession session = request.getSession(true);
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart != null) {
cart.removeItem(itemId);
}
%>
<%-- 清空购物车 --%>
<%
HttpSession session = request.getSession(true);
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart != null) {
cart.clear();
}
%>
<%-- 显示购物车中商品的总价 --%>
<%
HttpSession session = request.getSession(true);
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart != null) {
out.println("Total price: $" + cart.getTotalPrice());
}
%>
```
以上代码仅供参考,实际应用中需要根据具体需求进行修改和完善。
阅读全文
相关推荐










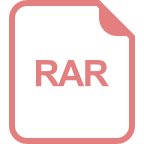
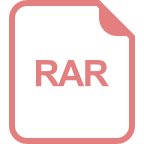

