Python代码怎么编写一个通信录管理系统(具有增加、修改、删除、查询等功能)。
时间: 2024-02-01 10:04:34 浏览: 93
您好!感谢您对ChitGPT的信任和使用。针对您的问题,如下是一个简单的Python代码实现:
```python
#通讯录管理系统
class AddressBook:
def __init__(self):
self.contacts = {}
def add_contact(self, name, phone_number, email_address):
self.contacts[name] = {'Phone Number': phone_number, 'Email Address': email_address}
print('{} has been added to the address book!'.format(name))
def update_contact(self, name, phone_number=None, email_address=None):
if name in self.contacts:
if phone_number is not None:
self.contacts[name]['Phone Number'] = phone_number
if email_address is not None:
self.contacts[name]['Email Address'] = email_address
print('{}\'s contact information has been updated!'.format(name))
else:
print('Sorry, {} is not in the address book.'.format(name))
def delete_contact(self, name):
if name in self.contacts:
del self.contacts[name]
print('{} has been deleted from the address book!'.format(name))
else:
print('Sorry, {} is not in the address book.'.format(name))
def search_contact(self, name):
if name in self.contacts:
print('{}\'s contact information:\nPhone Number: {}\nEmail Address: {}'.format(
name, self.contacts[name]['Phone Number'], self.contacts[name]['Email Address']))
else:
print('Sorry, {} is not in the address book.'.format(name))
#测试
my_address_book = AddressBook()
#添加联系人
my_address_book.add_contact('Tom', '1234567890', 'tom@example.com')
my_address_book.add_contact('Lisa', '0987654321', 'lisa@example.com')
#更新联系人
my_address_book.update_contact('Tom', phone_number='1111111111')
my_address_book.update_contact('David', phone_number='2222222222')
#删除联系人
my_address_book.delete_contact('Lisa')
my_address_book.delete_contact('Mike')
#查询联系人
my_address_book.search_contact('Tom')
my_address_book.search_contact('Lisa')
```
代码说明:
- AddressBook类是一个通讯录管理系统,保存在内存中,使用字典数据结构实现;
- add_contact方法接收3个参数:联系人姓名、电话号码、电子邮件地址,将联系人信息添加到通讯录;
- update_contact方法接收3个参数:联系人姓名、电话号码、电子邮件地址,根据参数更新联系人信息;
- delete_contact方法接收1个参数:联系人姓名,根据参数删除联系人;
- search_contact方法接收1个参数:联系人姓名,根据参数查询联系人信息。
祝您编写愉快!
阅读全文
相关推荐
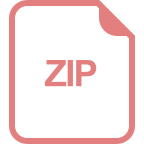
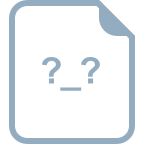
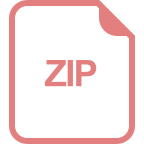















