用python获取boss招聘网上的招聘信息,并保存为csv文件以及进行可视化数据分析处理
时间: 2024-05-10 16:20:47 浏览: 87
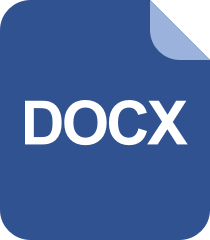
python爬取招聘网信息并保存为csv文件
作为AI语言模型,我无法进行网页爬取,但是我可以给您提供思路和代码示例供参考。
1. 网页爬取
Python中常用的网页爬取库有requests、BeautifulSoup、Scrapy等。在爬取boss招聘网的时候,可以先通过requests库获取网页源代码,再通过BeautifulSoup库解析网页,获取需要的招聘信息。
示例代码:
```
import requests
from bs4 import BeautifulSoup
# 请求网页
url = 'https://www.zhipin.com/job_detail/?query=Python&city=101010100&industry=&position='
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
# 解析网页
soup = BeautifulSoup(response.text, 'html.parser')
job_list = soup.find_all('div', class_='job-list')
# 获取招聘信息
for job in job_list:
job_name = job.find('div', class_='job-title').text.strip()
salary = job.find('span', class_='red').text.strip()
company = job.find('div', class_='company-text').find('a').text.strip()
location = job.find('div', class_='info-primary').find_all('p')[0].text.strip()
experience = job.find('div', class_='info-primary').find_all('p')[1].text.strip()
education = job.find('div', class_='info-primary').find_all('p')[2].text.strip()
print(job_name, salary, company, location, experience, education)
```
2. 保存为csv文件
在获取招聘信息之后,可以使用Python内置的csv库将数据保存为csv文件,方便后续的数据分析处理。
示例代码:
```
import csv
# 写入csv文件
with open('job.csv', 'w', newline='', encoding='utf-8') as f:
writer = csv.writer(f)
writer.writerow(['职位名称', '薪资', '公司名称', '工作地点', '工作经验', '学历要求'])
for job in job_list:
job_name = job.find('div', class_='job-title').text.strip()
salary = job.find('span', class_='red').text.strip()
company = job.find('div', class_='company-text').find('a').text.strip()
location = job.find('div', class_='info-primary').find_all('p')[0].text.strip()
experience = job.find('div', class_='info-primary').find_all('p')[1].text.strip()
education = job.find('div', class_='info-primary').find_all('p')[2].text.strip()
writer.writerow([job_name, salary, company, location, experience, education])
```
3. 可视化数据分析处理
在保存为csv文件之后,可以使用Python中的pandas、matplotlib等库进行数据分析和可视化处理。
示例代码:
```
import pandas as pd
import matplotlib.pyplot as plt
# 读取csv文件
df = pd.read_csv('job.csv')
# 数据分析处理
salary_group = df.groupby('薪资')['职位名称'].count().reset_index()
salary_group = salary_group.rename(columns={'职位名称': '数量'})
# 可视化处理
plt.bar(salary_group['薪资'], salary_group['数量'])
plt.title('不同薪资水平的职位数量')
plt.xlabel('薪资')
plt.ylabel('数量')
plt.show()
```
以上是对boss招聘网的简单爬取和数据分析处理,具体实现还需要根据实际需求进行调整和完善。
阅读全文
相关推荐
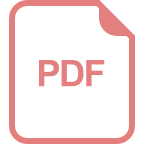
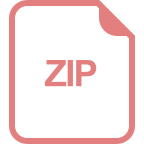


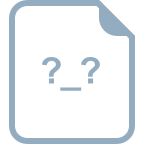
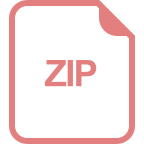
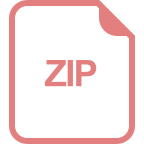
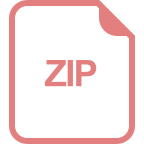
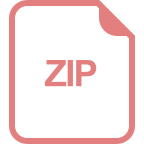
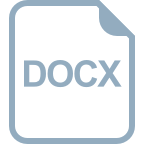
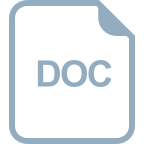
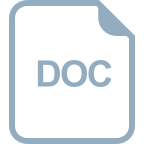
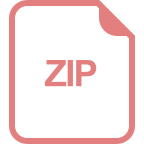
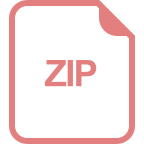
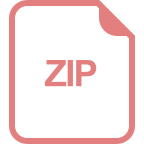
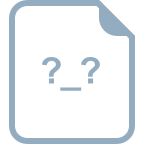