#include <iostream> #include <queue> using namespace std; const int MAXN = 1005; // 迷宫的最大大小 class Node { public: int x, y; // 当前节点的坐标 int step; // 到达当前节点的步数 }; int n, m; // 迷宫的大小 char maze[MAXN][MAXN]; // 迷宫矩阵 bool vis[MAXN][MAXN]; // 标记矩阵,表示该位置是否被访问过 int dx[] = { 0, 0, -1, 1 }; // 方向数组,表示上下左右四个方向 int dy[] = { -1, 1, 0, 0 }; Node start; Node end; // 起点和终点 bool bfs() { queue<Node> q; q.push(start); vis[start.x][start.y] = true; while (!q.empty()) { Node cur = q.front(); q.pop(); if (cur.x == end.x && cur.y == end.y) { // 到达终点 cout << "步数为:" << cur.step << endl; return true; } for (int i = 0; i < 4; i++) { int nx = cur.x + dx[i], ny = cur.y + dy[i]; if (nx >= 1 && nx <= n && ny >= 1 && ny <= m && maze[nx][ny] != '#' && !vis[nx][ny]) { Node next; next.x = nx; next.y = ny; next.step = cur.step + 1; q.push(next); vis[nx][ny] = true; } } } return false; } int main() { cin >> n >> m; for (int i = 1; i <= n; i++) { for (int j = 1; j <= m; j++) { cin >> maze[i][j]; if (maze[i][j] == 'S') { start.x = i; start.y = j; start.step = 0; } if (maze[i][j] == 'E') { end.x = i; end.y = j; } } } if (!bfs()) { cout << "无法到达终点!" << endl; } return 0; }这段代码有什么问题
时间: 2024-01-04 07:04:28 浏览: 60
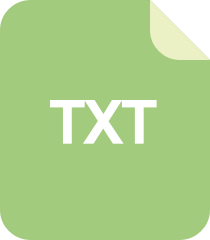
使用include <iostream>时一定要加using namespace std
这段代码看起来没有明显的语法问题,但可能存在以下问题:
1. 如果起点和终点不在迷宫的边缘,那么 `if (nx >= 1 && nx <= n && ny >= 1 && ny <= m && maze[nx][ny] != '#' && !vis[nx][ny])` 中的 `nx >= 1` 和 `ny >= 1` 可以省略,因为数组下标从 0 开始,应该改为 `nx >= 0` 和 `ny >= 0`。
2. 在 BFS 的过程中,需要记录每个节点的前驱节点,以便最后输出路径。可以使用一个二维数组来记录前驱节点。
3. 在输入迷宫时,需要判断迷宫的大小是否超过了数组的最大范围,以免发生数组越界的错误。
4. 在输出步数时,需要减去起点位置的步数,因为起点的步数为 0。
阅读全文
相关推荐
















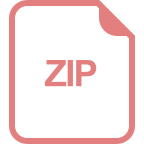