用python代码实现X-扫描填充算法,使用matplotlib库
时间: 2024-02-12 07:07:56 浏览: 129
好的,下面是用 Python 实现 X-扫描填充算法并使用 Matplotlib 库进行可视化的代码:
```python
import matplotlib.pyplot as plt
# 定义填充颜色
fill_color = (0.8, 0.8, 0.8)
# 定义边框颜色
edge_color = (0.2, 0.2, 0.2)
# 定义扫描线颜色
scanline_color = (0.4, 0.4, 0.4)
# 定义图形大小
fig, ax = plt.subplots(figsize=(6, 6))
# 定义多边形顶点
vertices = [(1, 1), (3, 1), (3, 3), (2, 3), (2, 2), (1, 2)]
# 绘制多边形边框
ax.plot([v[0] for v in vertices + [vertices[0]]],
[v[1] for v in vertices + [vertices[0]]],
color=edge_color)
# 定义扫描线起点
y_start = 1
# 定义扫描线终点
y_end = 3
# 遍历扫描线
for y in range(y_start, y_end + 1):
# 记录交点
intersections = []
# 遍历多边形边界
for i in range(len(vertices)):
# 获取当前边和下一个边的顶点
current_vertex = vertices[i]
next_vertex = vertices[(i + 1) % len(vertices)]
# 判断当前边是否与扫描线相交
if (current_vertex[1] <= y and next_vertex[1] > y) or \
(current_vertex[1] > y and next_vertex[1] <= y):
# 计算交点的 x 坐标
x = (y - current_vertex[1]) * \
(next_vertex[0] - current_vertex[0]) / \
(next_vertex[1] - current_vertex[1]) + \
current_vertex[0]
# 添加交点
intersections.append(x)
# 对交点排序
intersections.sort()
# 根据交点坐标填充区域
for i in range(0, len(intersections), 2):
ax.fill_betweenx([y, y+1],
intersections[i],
intersections[i+1],
color=fill_color)
# 显示扫描线
ax.axhline(y=y_start, color=scanline_color)
ax.axhline(y=y_end, color=scanline_color)
# 设置坐标轴范围
ax.set_xlim(0, 4)
ax.set_ylim(0, 4)
# 显示图形
plt.show()
```
这段代码会绘制一个多边形,并使用 X-扫描填充算法对其进行填充。你可以根据需要修改填充颜色、边框颜色、扫描线颜色等配置。
阅读全文
相关推荐
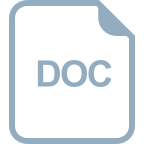
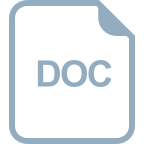
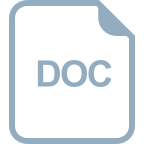
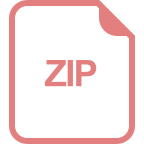
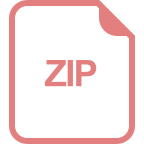
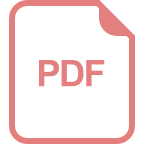
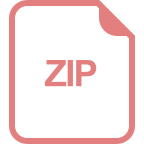
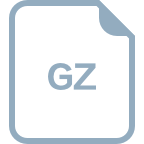
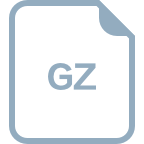
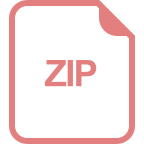
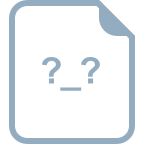
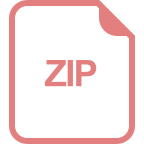
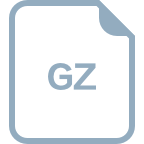
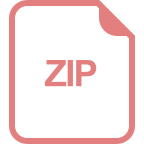
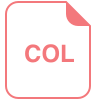


