将代办事项内容保存至sqllite数据库中
时间: 2023-10-09 15:06:09 浏览: 99
好的,那么您需要在代码中添加对SQLite数据库的支持。以下是一个示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>待办事项</title>
<style>
body {
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
}
form {
display: flex;
flex-direction: column;
align-items: center;
}
input[type=text] {
padding: 10px;
margin: 10px;
border-radius: 5px;
border: none;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
width: 300px;
}
input[type=submit] {
background-color: #4CAF50;
color: white;
padding: 10px;
border: none;
border-radius: 5px;
cursor: pointer;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
width: 300px;
margin-top: 10px;
}
ul {
list-style: none;
padding: 0;
margin-top: 20px;
width: 300px;
}
li {
padding: 10px;
border-radius: 5px;
border: none;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
margin-bottom: 10px;
display: flex;
justify-content: space-between;
align-items: center;
}
li span {
flex: 1;
}
li button {
background-color: #f44336;
color: white;
padding: 5px 10px;
border: none;
border-radius: 5px;
cursor: pointer;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
}
</style>
</head>
<body>
<h1>待办事项</h1>
<form>
<label for="newItem">添加新事项:</label>
<input type="text" id="newItem" placeholder="输入新事项">
<input type="submit" value="添加">
</form>
<ul id="list">
</ul>
<script>
const form = document.querySelector('form');
const input = document.querySelector('input');
const ul = document.querySelector('ul');
// 打开数据库
const db = openDatabase('todo', '1.0', '待办事项数据库', 2 * 1024 * 1024);
// 创建表
db.transaction(function(tx) {
tx.executeSql('CREATE TABLE IF NOT EXISTS todo (id INTEGER PRIMARY KEY, text TEXT)');
});
// 从数据库中读取数据,并显示在页面上
db.transaction(function(tx) {
tx.executeSql('SELECT * FROM todo', [], function(tx, result) {
const rows = result.rows;
for (let i = 0; i < rows.length; i++) {
const li = document.createElement('li');
const span = document.createElement('span');
const button = document.createElement('button');
span.textContent = rows.item(i).text;
button.textContent = '删除';
li.appendChild(span);
li.appendChild(button);
ul.appendChild(li);
button.addEventListener('click', function() {
// 从数据库中删除数据
db.transaction(function(tx) {
tx.executeSql('DELETE FROM todo WHERE id = ?', [rows.item(i).id]);
});
li.remove();
});
}
});
});
form.addEventListener('submit', function(e) {
e.preventDefault();
const text = input.value.trim();
if (text !== '') {
const li = document.createElement('li');
const span = document.createElement('span');
const button = document.createElement('button');
span.textContent = text;
button.textContent = '删除';
li.appendChild(span);
li.appendChild(button);
ul.appendChild(li);
input.value = '';
// 将数据保存到数据库
db.transaction(function(tx) {
tx.executeSql('INSERT INTO todo (text) VALUES (?)', [text]);
});
button.addEventListener('click', function() {
// 从数据库中删除数据
db.transaction(function(tx) {
tx.executeSql('DELETE FROM todo WHERE id = ?', [li.dataset.id]);
});
li.remove();
});
}
});
</script>
</body>
</html>
```
在这个示例代码中,我们使用了Web SQL Database API来创建和操作SQLite数据库。在打开数据库后,我们创建了一个名为`todo`的表,并在其中保存待办事项的文本内容。在添加待办事项时,我们将文本内容保存到数据库中,并在页面上显示出来。在删除待办事项时,我们从数据库中删除对应的数据,并将它从页面上移除。
阅读全文
相关推荐
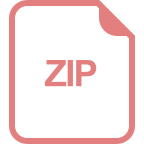
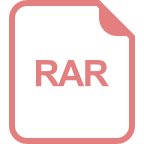
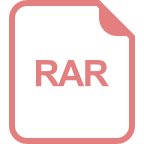
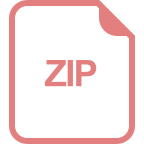
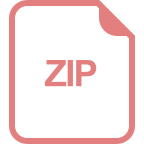
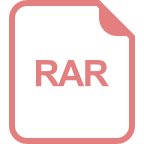
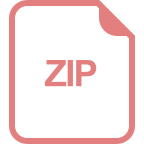




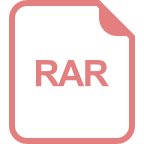

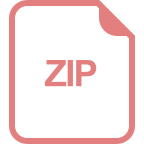
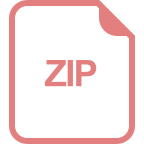
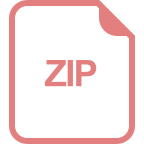