使用 C++设计一个程序,功能如下: 从文件“input.txt”中读入数据(一组二维坐标(x,y)),文件格式如下: 大 34.1 45,44 -323.67 493.56 42.55 -89.22 34.11 99.56 ...... 每行第一个数为 x,第二个数为y。 读取数据后,通过按键控制程序: 1.输入字符为's’时,在屏幕上显示所有坐标; 2.输入字符为'x’时,依据x对所有坐标进行排序并输出到屏幕; 3.输入字符为'y’时,依据y对所有坐标进行排序并输出到屏幕; 4.输入字符为'n’时,在屏幕上输出坐标数目; 5.输入字符为'c’时,在屏幕上输出所有点的质心位置(假设每个点质量相同); 6.输入字符为'l’时,对坐标进行最小二乘法拟合,输出拟合的斜率、截距 和误差; 7.输入字符为'i’时,要求用户再输入一个整数 n,输入后屏幕显示第n个 发给 坐标值; 8.输入字符为'v’时,所有坐标沿x轴进行镜像变换,并将变换后的坐标屏幕上输出; 9.输入字符为'h’时,所有坐标沿y轴进行镜像变换,并将变换后的坐标在屏幕上输出; 10.输入字符为'r’时,要求用户再输入一个数 a,输入后所有坐标绕原点旋转a度; 11.输入字符为'm’时,要求用户再输入两个数 x0,y0,输入后将所有坐标沿 x和y轴分别平移 x0,y0,并在屏幕上输出所有坐标;12.输入字符为'e’时,退出程序。
时间: 2024-03-18 16:41:56 浏览: 90
好的,以下是 C++ 实现程序的代码,注释中有详细说明:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
#include <math.h>
using namespace std;
// 定义坐标点类
class Point {
public:
double x, y;
Point() {}
Point(double a, double b) { x = a; y = b; }
// 重载小于符号,用于排序
bool operator<(const Point& rhs) const { return x < rhs.x; }
};
// 定义文件读取函数
vector<Point> readFile(string filename) {
vector<Point> points;
ifstream fin(filename);
if (!fin) {
cerr << "Failed to open file: " << filename << endl;
exit(1);
}
double x, y;
while (fin >> x >> y) {
points.push_back(Point(x, y));
}
fin.close();
return points;
}
// 定义输出所有坐标函数
void printPoints(vector<Point>& points) {
for (int i = 0; i < points.size(); i++) {
cout << "(" << points[i].x << ", " << points[i].y << ")" << endl;
}
}
// 定义按 x 排序输出函数
void sortByX(vector<Point>& points) {
sort(points.begin(), points.end());
printPoints(points);
}
// 定义按 y 排序输出函数
void sortByY(vector<Point>& points) {
sort(points.begin(), points.end(), [](const Point& a, const Point& b) { return a.y < b.y; });
printPoints(points);
}
// 定义输出坐标数目函数
void printNumOfPoints(vector<Point>& points) {
cout << "Number of points: " << points.size() << endl;
}
// 定义输出质心位置函数
void printCenterOfGravity(vector<Point>& points) {
double cx = 0, cy = 0;
for (int i = 0; i < points.size(); i++) {
cx += points[i].x;
cy += points[i].y;
}
cx /= points.size();
cy /= points.size();
cout << "Center of gravity: (" << cx << ", " << cy << ")" << endl;
}
// 定义最小二乘法拟合函数
void leastSquaresFitting(vector<Point>& points) {
double sumX = 0, sumY = 0, sumXY = 0, sumXX = 0;
for (int i = 0; i < points.size(); i++) {
sumX += points[i].x;
sumY += points[i].y;
sumXY += points[i].x * points[i].y;
sumXX += points[i].x * points[i].x;
}
double n = points.size();
double a = (n * sumXY - sumX * sumY) / (n * sumXX - sumX * sumX);
double b = (sumY - a * sumX) / n;
double err = 0;
for (int i = 0; i < points.size(); i++) {
err += pow(points[i].y - a * points[i].x - b, 2);
}
err = sqrt(err / n);
cout << "Slope: " << a << endl;
cout << "Intercept: " << b << endl;
cout << "Error: " << err << endl;
}
// 定义按序号输出函数
void printPointByIndex(vector<Point>& points) {
int index;
cout << "Please enter the index: ";
cin >> index;
if (index < 1 || index > points.size()) {
cerr << "Invalid index." << endl;
return;
}
cout << "(" << points[index - 1].x << ", " << points[index - 1].y << ")" << endl;
}
// 定义 x 轴镜像变换函数
void mirrorByXAxis(vector<Point>& points) {
for (int i = 0; i < points.size(); i++) {
points[i].y = -points[i].y;
}
printPoints(points);
}
// 定义 y 轴镜像变换函数
void mirrorByYAxis(vector<Point>& points) {
for (int i = 0; i < points.size(); i++) {
points[i].x = -points[i].x;
}
printPoints(points);
}
// 定义旋转函数
void rotate(vector<Point>& points) {
double angle;
cout << "Please enter the angle (in degrees): ";
cin >> angle;
double rad = angle * M_PI / 180;
for (int i = 0; i < points.size(); i++) {
double x = points[i].x * cos(rad) - points[i].y * sin(rad);
double y = points[i].x * sin(rad) + points[i].y * cos(rad);
points[i].x = x;
points[i].y = y;
}
printPoints(points);
}
// 定义平移函数
void translate(vector<Point>& points) {
double x, y;
cout << "Please enter the x and y translation: ";
cin >> x >> y;
for (int i = 0; i < points.size(); i++) {
points[i].x += x;
points[i].y += y;
}
printPoints(points);
}
int main() {
// 读取文件
vector<Point> points = readFile("input.txt");
char command;
while (true) {
cout << "Please enter a command: ";
cin >> command;
switch (command) {
case 's':
printPoints(points);
break;
case 'x':
sortByX(points);
break;
case 'y':
sortByY(points);
break;
case 'n':
printNumOfPoints(points);
break;
case 'c':
printCenterOfGravity(points);
break;
case 'l':
leastSquaresFitting(points);
break;
case 'i':
printPointByIndex(points);
break;
case 'v':
mirrorByXAxis(points);
break;
case 'h':
mirrorByYAxis(points);
break;
case 'r':
rotate(points);
break;
case 'm':
translate(points);
break;
case 'e':
return 0;
default:
cerr << "Unknown command." << endl;
break;
}
}
return 0;
}
```
阅读全文
相关推荐
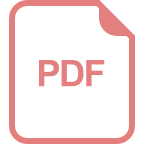
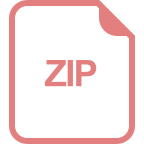


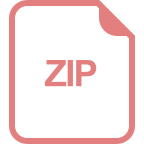
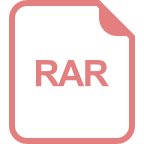
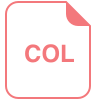

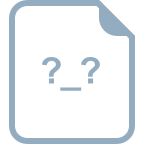
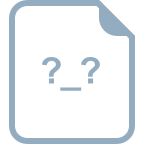
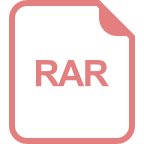
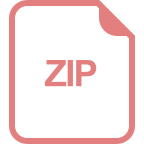