给出用PHP和MySQL语言实现论坛(功能包括用户登录和注册,更改密码。发表帖子,回复帖子和删除帖子)代码和表创建代码
时间: 2023-06-12 19:07:30 浏览: 53
表创建代码:
```sql
CREATE TABLE `user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(50) NOT NULL,
`password` varchar(50) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `post` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(255) NOT NULL,
`content` text NOT NULL,
`author_id` int(11) NOT NULL,
`created_at` datetime NOT NULL,
PRIMARY KEY (`id`),
KEY `author_id` (`author_id`),
CONSTRAINT `post_ibfk_1` FOREIGN KEY (`author_id`) REFERENCES `user` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `comment` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`content` text NOT NULL,
`author_id` int(11) NOT NULL,
`post_id` int(11) NOT NULL,
`created_at` datetime NOT NULL,
PRIMARY KEY (`id`),
KEY `author_id` (`author_id`),
KEY `post_id` (`post_id`),
CONSTRAINT `comment_ibfk_1` FOREIGN KEY (`author_id`) REFERENCES `user` (`id`) ON DELETE CASCADE ON UPDATE CASCADE,
CONSTRAINT `comment_ibfk_2` FOREIGN KEY (`post_id`) REFERENCES `post` (`id`) ON DELETE CASCADE ON UPDATE CASCADE
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
PHP代码:
```php
<?php
// 连接数据库
$conn = mysqli_connect('localhost', 'root', 'password', 'forum');
// 检查连接是否成功
if (!$conn) {
die('连接数据库失败: ' . mysqli_connect_error());
}
// 处理注册请求
if (isset($_POST['register'])) {
$username = $_POST['username'];
$password = $_POST['password'];
// 检查用户名是否已经存在
$check_sql = "SELECT * FROM `user` WHERE `username` = '$username'";
$result = mysqli_query($conn, $check_sql);
if (mysqli_num_rows($result) > 0) {
echo '<script>alert("用户名已经存在");</script>';
} else {
// 插入新用户记录
$insert_sql = "INSERT INTO `user` (`username`, `password`) VALUES ('$username', '$password')";
if (mysqli_query($conn, $insert_sql)) {
echo '<script>alert("注册成功");</script>';
} else {
echo '<script>alert("注册失败,请稍后重试");</script>';
}
}
}
// 处理登录请求
if (isset($_POST['login'])) {
$username = $_POST['username'];
$password = $_POST['password'];
// 检查用户名和密码是否匹配
$check_sql = "SELECT * FROM `user` WHERE `username` = '$username' AND `password` = '$password'";
$result = mysqli_query($conn, $check_sql);
if (mysqli_num_rows($result) > 0) {
// 登录成功,记录用户信息到 session 中
session_start();
$_SESSION['username'] = $username;
$_SESSION['user_id'] = mysqli_fetch_assoc($result)['id'];
header('Location: index.php');
exit();
} else {
echo '<script>alert("用户名或密码错误");</script>';
}
}
// 处理发帖请求
if (isset($_POST['create_post'])) {
$title = $_POST['title'];
$content = $_POST['content'];
$author_id = $_SESSION['user_id'];
$created_at = date('Y-m-d H:i:s');
// 插入新帖子记录
$insert_sql = "INSERT INTO `post` (`title`, `content`, `author_id`, `created_at`) VALUES ('$title', '$content', $author_id, '$created_at')";
if (mysqli_query($conn, $insert_sql)) {
echo '<script>alert("发帖成功");</script>';
} else {
echo '<script>alert("发帖失败,请稍后重试");</script>';
}
}
// 处理回帖请求
if (isset($_POST['create_comment'])) {
$content = $_POST['content'];
$author_id = $_SESSION['user_id'];
$post_id = $_POST['post_id'];
$created_at = date('Y-m-d H:i:s');
// 插入新评论记录
$insert_sql = "INSERT INTO `comment` (`content`, `author_id`, `post_id`, `created_at`) VALUES ('$content', $author_id, $post_id, '$created_at')";
if (mysqli_query($conn, $insert_sql)) {
echo '<script>alert("回帖成功");</script>';
} else {
echo '<script>alert("回帖失败,请稍后重试");</script>';
}
}
// 处理删除帖子请求
if (isset($_POST['delete_post'])) {
$post_id = $_POST['post_id'];
// 删除帖子及其相关评论
$delete_post_sql = "DELETE FROM `post` WHERE `id` = $post_id";
$delete_comment_sql = "DELETE FROM `comment` WHERE `post_id` = $post_id";
if (mysqli_query($conn, $delete_post_sql) && mysqli_query($conn, $delete_comment_sql)) {
echo '<script>alert("删除帖子成功");</script>';
} else {
echo '<script>alert("删除帖子失败,请稍后重试");</script>';
}
}
```
注意:以上代码仅供参考,需要根据具体情况进行修改和完善。
相关推荐
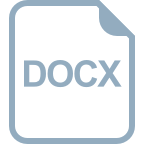
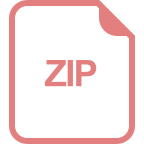














