gabor卷积 pytorch
时间: 2023-09-09 07:09:52 浏览: 176
Gabor卷积是一种特殊的卷积操作,它可以用于图像处理、特征提取等领域。在PyTorch中,可以使用torchvision中的transforms模块实现Gabor卷积。
首先,需要安装`torchvision`模块:
```python
pip install torchvision
```
然后,可以使用如下代码实现Gabor卷积:
```python
import torch
import torchvision.transforms as transforms
from torchvision.transforms.functional import pad
import numpy as np
def gabor_kernel(size, sigma, theta, lambd, psi=0, gamma=1):
"""Generate Gabor kernel"""
x, y = np.meshgrid(np.linspace(-1, 1, size), np.linspace(-1, 1, size))
x_theta = x * np.cos(theta) + y * np.sin(theta)
y_theta = -x * np.sin(theta) + y * np.cos(theta)
gb = np.exp(-0.5 * (x_theta**2 + gamma**2 * y_theta**2) / sigma**2) * np.cos(2 * np.pi * x_theta / lambd + psi)
return gb
class GaborConv2d(torch.nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, sigma, theta, lambd, psi=0, gamma=1):
super(GaborConv2d, self).__init__()
self.in_channels = in_channels
self.out_channels = out_channels
self.kernel_size = kernel_size
self.sigma = sigma
self.theta = theta
self.lambd = lambd
self.psi = psi
self.gamma = gamma
# Generate Gabor kernels
kernels = []
for i in range(out_channels):
kernel = gabor_kernel(kernel_size, sigma, theta, lambd, psi, gamma)
kernel = np.repeat(kernel[:, :, np.newaxis], in_channels, axis=2)
kernels.append(kernel)
self.kernels = torch.FloatTensor(kernels)
# Make kernels as parameters of the model
self.kernels = torch.nn.Parameter(self.kernels)
def forward(self, x):
# Pad input tensor
x = pad(x, (self.kernel_size // 2, self.kernel_size // 2, self.kernel_size // 2, self.kernel_size // 2))
# Convolve input tensor with Gabor kernels
out = torch.nn.functional.conv2d(x, self.kernels)
return out
```
上述代码中,`gabor_kernel`函数用于生成Gabor核,`GaborConv2d`类继承自`torch.nn.Module`,用于实现Gabor卷积。在`GaborConv2d`类的初始化函数中,生成Gabor核,并将其作为模型参数。在`forward`函数中,使用`torch.nn.functional.conv2d`函数进行卷积操作。
使用时,可以像使用其他PyTorch卷积层一样使用GaborConv2d层:
```python
gabor_conv = GaborConv2d(in_channels=3, out_channels=16, kernel_size=7, sigma=1.0, theta=0.0, lambd=5.0, psi=0.0, gamma=1.0)
input_tensor = torch.randn(1, 3, 224, 224)
output_tensor = gabor_conv(input_tensor)
```
上述代码中,`in_channels`表示输入通道数,`out_channels`表示输出通道数,`kernel_size`表示卷积核大小,`sigma`表示Gabor核的标准差,`theta`表示Gabor核的方向,`lambd`表示Gabor核的波长,`psi`表示Gabor核的相位,`gamma`表示Gabor核的纵横比。`input_tensor`是输入张量,`output_tensor`是输出张量。
阅读全文
相关推荐
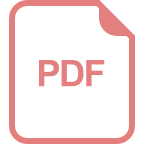
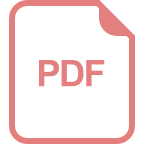


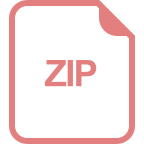
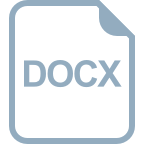
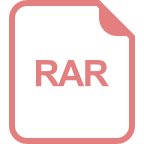
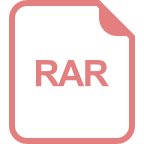
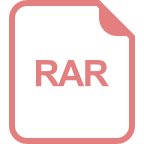
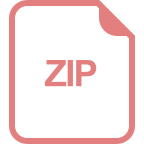
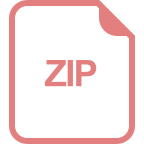
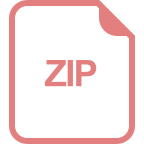
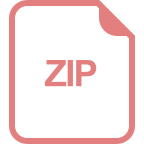