#include<iostream> using namespace std; class Date; //对 Date 类的提前引用声明 class Time //定义 Time 类 {public: Time (int, int,int); void display (Date &); //display 是成员函数,形参是 Date 类对象的引用 private: int hour; int minute; int sec; }; class Date //声明 Date 类 {public: Date(int, int, int) ; friend void Time::display Date &); //声明 Time 中的 display 函数为友元成员函数 private: int month; int day ; int year; Tine::Time(int h, int m, int s) //类 Time 的构造函数 {hour = h; minute = m; sec=s; } void Time::display (Date &d) //display 的作用是输出年、月、日和时、分、秒 {cout<<d.month<<"/"<<d.day<<"/"<<d.year<<endl; //引用 Date 类对象中的私有数据 cout<<hour<<":"<<minute<<":"<<sec<<endl; //引用本类对象 中的私有数据 Date::Date(int m, int d, int y) //类 Date 的构造函数 {month=m; day=d; year = y; } int main() {Time t1(10,13, 56) ; //定义 Time 类对象 t1 Date d1 (12, 25, 2004) ; //定义 Date 类对象 d1 t1.display(d1) ; //调用 t1 中的 display 函数,实参是 Date 类对象 d1 return 0; }将程序中的 display 函 数不放在 Time 类中,而作为类外的普通函数,然后分别在 Time 和 Date 类中将 display 声明 为友元函数。在主函数中调用 display 函数,display 函数分别引用 Time 和 Date 两个类的对 象的私有数据,输出年、月、日和时、分、秒。
时间: 2024-02-07 17:02:12 浏览: 74
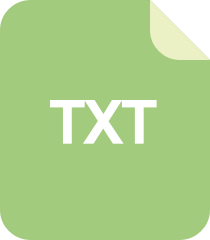
#include_iostream.h_

修改后的程序如下所示:
```cpp
#include<iostream>
using namespace std;
class Date; //对 Date 类的提前引用声明
class Time //定义 Time 类
{
public:
Time(int, int, int);
friend void display(Time&, Date&); //声明 display 函数为友元成员函数
private:
int hour;
int minute;
int sec;
};
class Date //声明 Date 类
{
public:
Date(int, int, int);
friend void display(Time&, Date&); //声明 display 函数为友元成员函数
private:
int month;
int day;
int year;
};
Time::Time(int h, int m, int s) //类 Time 的构造函数
{
hour = h;
minute = m;
sec = s;
}
Date::Date(int m, int d, int y) //类 Date 的构造函数
{
month = m;
day = d;
year = y;
}
void display(Time& t, Date& d) //类外的普通函数
{
cout << d.month << "/" << d.day << "/" << d.year << endl; //引用 Date 类对象中的私有数据
cout << t.hour << ":" << t.minute << ":" << t.sec << endl; //引用 Time 类对象中的私有数据
}
int main()
{
Time t1(10, 13, 56); //定义 Time 类对象 t1
Date d1(12, 25, 2004); //定义 Date 类对象 d1
display(t1, d1); //调用 display 函数,实参分别是 Time 类对象和 Date 类对象
return 0;
}
```
在修改后的程序中,我们将`display`函数从`Time`类中移除,成为了一个类外的普通函数。然后,在`Time`类和`Date`类中将`display`函数分别声明为友元函数。
在`main`函数中,我们创建了一个`Time`类对象`t1`和一个`Date`类对象`d1`,然后调用了`display`函数,该函数同时引用了`t1`和`d1`两个对象的私有数据,输出了年、月、日和时、分、秒。
阅读全文
相关推荐
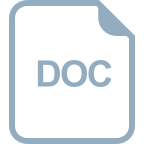
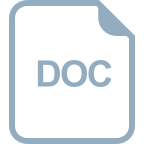
















