pyhton多线程通信
时间: 2023-09-12 18:10:14 浏览: 41
在 Python 中,可以使用内置的 `threading` 模块来创建和管理线程。多线程通信可以使用共享变量或队列来实现。
共享变量是多个线程可以同时访问和修改的变量,当多个线程同时修改同一个共享变量时,可能会出现竞争条件和数据不一致的问题,因此需要使用锁来保护共享变量。Python 提供了 `Lock`、`RLock`、`Semaphore` 等锁来实现多线程同步。
例如,以下是使用 `Lock` 来保护共享变量的示例代码:
```python
import threading
# 共享变量
count = 0
# 创建锁
lock = threading.Lock()
# 线程函数
def worker():
global count
# 获取锁
lock.acquire()
count += 1
# 释放锁
lock.release()
# 创建线程
threads = []
for i in range(10):
t = threading.Thread(target=worker)
threads.append(t)
# 启动线程
for t in threads:
t.start()
# 等待线程结束
for t in threads:
t.join()
# 输出结果
print(count)
```
队列是一种线程安全的数据结构,可以用来在多个线程之间传递数据。Python 提供了 `queue` 模块来实现队列。
例如,以下是使用队列进行多线程通信的示例代码:
```python
import threading
import queue
# 创建队列
q = queue.Queue()
# 线程函数
def worker():
while True:
# 从队列中获取数据
data = q.get()
if data is None:
break
# 处理数据
print(data)
# 创建线程
threads = []
for i in range(10):
t = threading.Thread(target=worker)
threads.append(t)
# 启动线程
for t in threads:
t.start()
# 向队列中添加数据
for i in range(100):
q.put(i)
# 结束线程
for i in range(10):
q.put(None)
for t in threads:
t.join()
```
在以上示例中,创建了一个队列 `q`,并创建了多个线程来处理队列中的数据。主线程向队列中添加数据,然后向队列中添加多个 `None`,表示所有数据已经添加完毕,线程可以退出了。
相关推荐
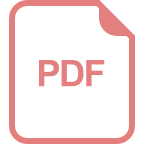
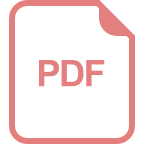
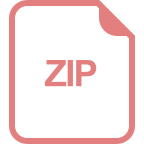















